Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial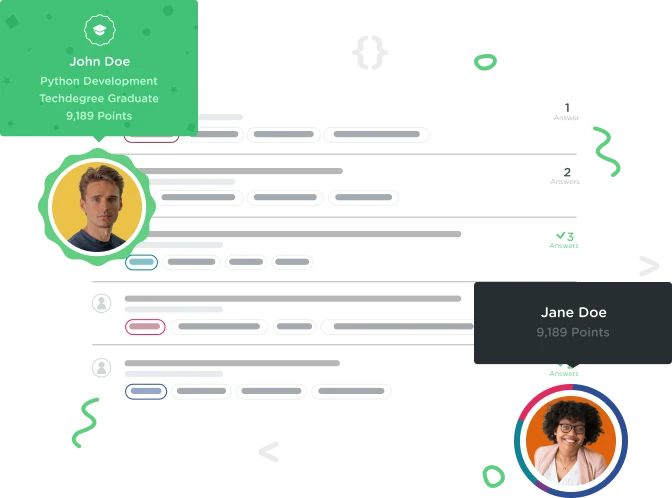
MUZ140151 Gilbert Chapara
2,621 Pointsharnessing the power of objects
I have added a method drive that defines a parameter laps. These high-powered GoKarts use 1 energy bar off their battery per lap. Using method signatures, add a new default method called drive that performs just 1 lap and takes no arguments.
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
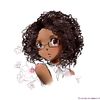
Gloria Dwomoh
13,116 PointsMUZ140151 Chapara Try posting your effort ie. how you tried to solve it. So that others can be able to help you better :)

Spencer Saunders
1,981 PointsI'm not understanding this concept either. If somebody could please help me, it would be much appreciated.

Thomas Biddulph
2,184 PointsPay special attention to these statements: GoKarts use 1 energy bar off their battery per lap, Using method signatures, add a new default method called drive that performs just 1 lap and takes no arguments.
In other words, write a new drive method with no parameters that decrements (decreases by 1) mBarsCount.
2 Answers
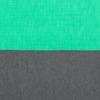
Victor Levytskiy
7,608 PointsYou just need to create a drive method in which you call drive method with parameter
public void drive()
{
drive(1);
}

Pavel Piskunov
2,410 PointsWhy it works? We have two methods with same name. Why Java compiler not change stuff in method, when you declare it in second time, or get an error?

Andrew Winkler
37,739 PointsWe are looking to "perform" a method called drive that takes 1 lap with no arguments. It is considered a public method and it is not looking to return anything either so public void starts of the method notation:
public void drive() { drive(1); }
To break it down piece by piece: It's a public function/ that doesn't return anything/ where drive is the method name/ takes no arguments/ and it runs the other method signature (taking 1 lap as the parameter).
Tom Schinler
21,052 PointsTom Schinler
21,052 PointsAre you just looking for the answer or are you not understanding the task at hand and looking for hints and a breakdown?
The task is looking for you to create a default method that runs 1 lap. The method should be made available to other classes, and return nothing.