Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial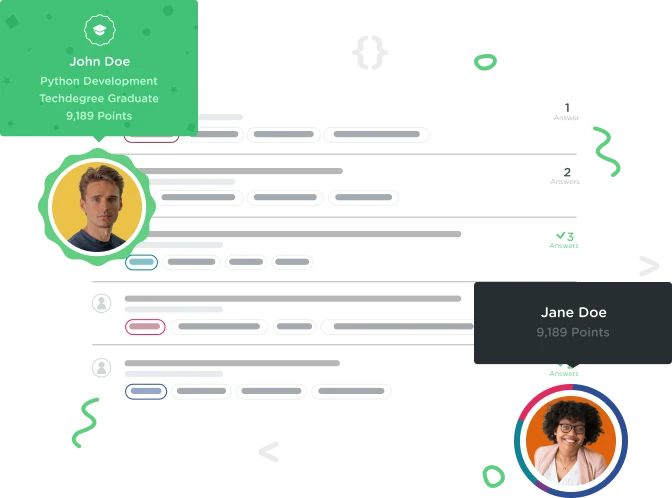

stevenmcnitt
9,437 PointsHarnessing the Power of Objects- helper method
I would like to run this method with an if statement however I get this error..
./GoKart.java:24: error: incompatible types if(MAX_BARS) { ^ required: boolean found: int 1 error
How can I make my 'if' statement compatible with a boolean so this code will run???
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
mBarsCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
if(MAX_BARS) {
return true;
}
}
}
2 Answers

Jess Sanders
12,086 PointsUse the following snippet of code as your example to follow in changing the implementation details of the charge method:
public boolean dispense() {
boolean wasDispensed = false;
if (!isEmpty()) {
mPezCount--;
wasDispensed = true;
}
return wasDispensed;
}
- Instead of if, use while
- increment, rather than decrement
- The charge method doesn't need to return a boolean, so ignore all of the code involving the boolean wasDispensed
Keep isFullyCharged as it was from the previous challenge.
public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
}

Ken Alger
Treehouse TeacherSteven;
As you figured out, Java is expecting a Boolean value in the if condition and you are passing it an integer value.
One way to solve this for this code example would be to check if MAX_BARS
is equal to mBarsCount
in the if condition. That would generate a true/false condition, correct?
Happy coding,
Ken

stevenmcnitt
9,437 PointsThanks Ken! Would it also be possible to initialize MAX_BARS to a boolean value just before my isFullyCharged method and then run the code by passing the newly initialized MAX_BARS to my 'if' condition?
I suppose it is a matter of scope as well; for example, could run the following:
someIntVar = 0; // initialize to int data type
public boolean someBoolVar() { boolean newDataType = someIntVar //change data type from int to bool if(newDataType) { //passes bool data type to bool method? return true; }else{ return false; }
}
Or is it not possible to change data types through variable assignment this way? If not, perhaps java already has a method for this I haven't learned yet? Just some thoughts..

Ken Alger
Treehouse TeacherSteven;
I see what you are getting at, but I am having difficulty understanding why you would do that in this case as it seems much simpler to pass the if conditional the check for equality of MAX_BARS
versus mBarsCount
. Seems much easier and less code to write than creating a bunch of intermediary variables.
In terms of converting a Boolean value to an Integer, take a look at this post or this one.
Post back if this is not what you are talking about.
Ken