Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial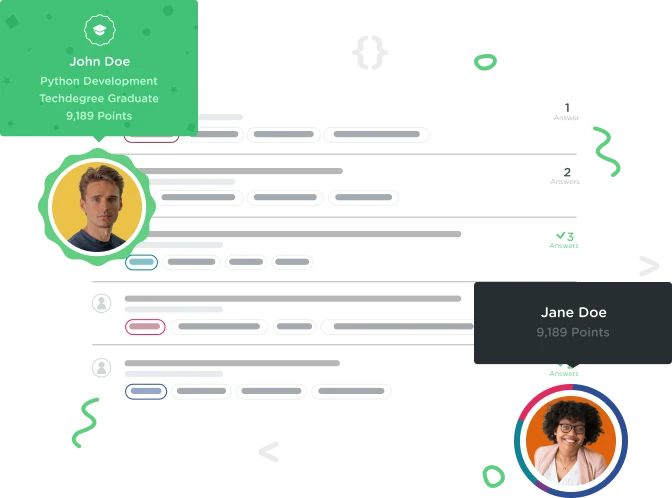

Steven Walters
10,573 PointsHas anybody done the Interactive Web Pages project with jQuery?
I'd like to see how it would differ. I think it would be a great bonus lesson to have Andrew come back and show how he would do the same project using jQuery.
4 Answers
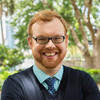
Andrew Chalkley
Treehouse Guest TeacherI'm currently working on Node.js material at the moment. But when you're done with jQuery Basics see if you can build it using jQuery.
Also, when you do – don't be shy – share your code!
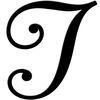
Tahseen Malik
16,770 PointsHi, I just took this course and have started learning JQuery. This is the Todo list in JQuery. I have Improved on the instructor's version
- You can't add a empty task
- When the edit button is clicked, Edit changes to Save (and back to Edit when clicked again)
- When the task is in Edit mode, in incomplete or completed list, and the checkbox is clicked, the task will change out of Edit mode before getting added to the other list.
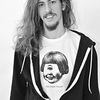
eck
43,038 PointsThat sounds like a good exercise, you should totally try it :D
If you do, come back and share the experience!
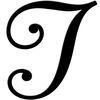
Tahseen Malik
16,770 Points//--- The TodoList in JQuery ---
var incompleteTasksHolder = $('#incomplete-tasks');
var completedTasksHolder = $('#completed-tasks');
// --- Function for Editing an existing task ---
var editTask = function () {
var listItem = $(this).parent();
var listTextInput = listItem.find('input[type="text"]');
var listLabel = listItem.find('label');
if ( listItem.hasClass('editMode') ) {
listLabel.text( listTextInput.val() );
listTextInput.removeAttr('value');
listItem.removeAttr('class');
$(this).text('Edit');
} else {
listTextInput.val( listLabel.text() );
listItem.addClass('editMode');
$(this).text('Save');
}
};
// --- Function for Deleting an existing task ---
var deleteTask = function () {
$(this).parent().remove();
};
// --- Function for Adding a new task ---
var addTask = function() {
var taskInput = $('#new-task');
if ( taskInput.val() == '' ) {
alert('You Can\'t add a Empty Task');
} else {
var listItem = $('<li><input type="checkbox"><label>' + taskInput.val() + '</label><input type="text"><button class="edit">Edit</button><button class="delete">Delete</button></li>');
incompleteTasksHolder.append(listItem);
taskInput.val('');
//Bind Event Handler to the task that was Added
var newTaskAdded = incompleteTasksHolder.find('li').last();
newTaskAdded.find('.edit').on('click', editTask);
newTaskAdded.find('.delete').on('click', deleteTask);
newTaskAdded.find('input[type="checkbox"]').on('click', markTask);
}
};
// --- Function for Marking a task Complete or Incomplete ---
var markTask = function() {
//This function will check if the task is in edit mode, if true will change it
function checkEditState(task) {
if ( task.hasClass('editMode') ) {
task.find('label').text( task.find('input[type="text"]').val() )
task.find('input[type="text"]').removeAttr('value');
task.removeAttr('class');
task.find('.edit').text('Edit');
}
}
if ( $(this).closest('ul').attr('id') == 'incomplete-tasks' ) {
var completedTask = $(this).parent().remove();
checkEditState(completedTask);
completedTask.find('input[type="checkbox"]').prop( "checked", true );
bindTaskEvents( completedTask );
completedTasksHolder.append(completedTask);
} else {
var taskToComplete = $(this).parent().remove();
checkEditState(taskToComplete);
taskToComplete.find('input[type="checkbox"]').removeAttr('checked');
bindTaskEvents( taskToComplete );
incompleteTasksHolder.append(taskToComplete);
}
};
// --- Function for binding Event Handler ---
function bindTaskEvents (item) {
item.find('.edit').on('click', editTask);
item.find('.delete').on('click', deleteTask);
item.find('input[type="checkbox"]').on('click', markTask);
}
// Binding Event handlers to Add, checkboxes, edit and delete
$('#new-task').next().on('click', addTask);
bindTaskEvents( $('li') );
Ken Alger
Treehouse TeacherKen Alger
Treehouse TeacherTagging Andrew Chalkley.