Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial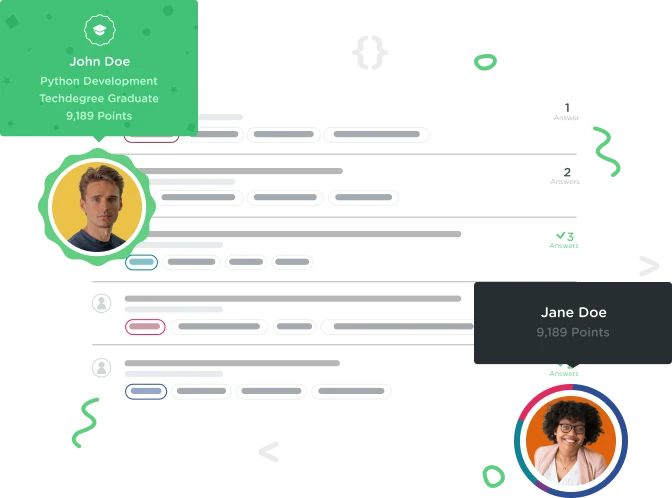
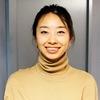
Flore W
4,744 PointsHas anyone done the Extra Credit? Can you share what you've done?
At the end of the Regex course, Kenneth mentions some extra credit:
"Write a class to represent a person based on the information in the text file. They should have names, email addresses, phone numbers, jobs, and Twitter accounts. Remember, some of these can be blank, though!
To go ever further, make a class to act as as address book to hold all of the people class instances created above. Can you make it searchable?"
Can anyone who's done it share their solutions? I am still not comfortable with classes, so would appreciate to get hints of how to do it. Thanks!
1 Answer
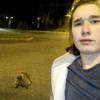
Oskar Lundberg
9,534 PointsI know this was asked a long time ago, but if someone wants to have a look, here is some code I wrote: I know this could be done it better ways, but at this point, I'm just gonna leave the extra credits behind.
import re
names_file = open("names.txt", encoding="utf-8")
data = names_file.read()
names_file.close()
line = re.compile(r"""
^(?P<name>(?P<last>[-\w ]*),\s(?P<first>[-\w ]+))\t # Last and first names
(?P<email>[-\w\d.+]+@[-\w\d.]+)\t # Email
(?P<phone>\(?\d{3}\)?\-?\s?\d{3}-\d{4})?\t # Phone
(?P<job>[\w\s]+,\s[\w\s.]+)\t? # Job and company
(?P<twitter>@[\w\d]+)?$ # Twitter
""", re.X|re.M)
class Person():
def __init__(self, name, email, job, phone=None, twitter=None, **kwargs):
self.name = name
self.email = email
self.job = job
self.phone = phone
self.twitter = twitter
for key, value in kwargs.items():
setattr(self, key, value)
def __repr__(self):
cool_list = []
if self.phone:
cool_list.append("Phone: <{}>".format(self.phone))
if self.twitter:
cool_list.append("Twitter: <{}>".format(self.twitter))
return "Name: <{}>, Email: <{}>, Job: <{}>, ".format(self.name, self.email, self.job) + ", ".join(cool_list)
def __str__(self):
return self.name
class AddressBook(list):
def __init__(self):
self.address_book = []
def add_person(self, person):
# Todo: fix so this goes smoother
"""Supply with a Person. must have the attributes 'first' and 'last' for first and last name
Adds that person to the addres_book
"""
try:
person.first
person.last
except AttributeError:
print("The person needs to have the attributes 'first' and 'last' for first and last name")
else:
return self.append(person)
def show_person(self, first_name=None, last_name=None):
# Todo: Add so it's not case sensitive
person_list = []
if first_name:
first_name = first_name.lower()
if last_name:
last_name = last_name.lower()
for person in self:
if str(person.first).lower() == first_name or str(person.last).lower() == last_name:
person_list.append(person)
if len(person_list) == 1:
return person_list[0]
elif len(person_list) == 0:
if first_name and last_name:
print("Couldn't find any person with the firstname: {} and/or lastname: {}".format(first_name, last_name))
elif first_name:
print("Couldn't find any person with the firstname: {}".format(first_name))
elif last_name:
print("Couldn't find any person with the lastname: {}".format(last_name))
else:
person_list_exact = []
for person in person_list:
if str(person.first).lower() == first_name and str(person.last).lower() == last_name:
person_list_exact.append(person)
if len(person_list_exact) == 1:
return person_list_exact[0]
elif len(person_list_exact) == 0:
while True:
print("There are multiple persons who match some of your criteria")
print("Please choose one from the below choices, choose by index")
for index, person in enumerate(person_list, 1):
print("{}: {}".format(index, person))
answer = input("> ")
if isinstance(answer, str): # <-- Fix this
answer = answer.lower()
possible_persons = []
for person in person_list:
if answer in str(person.name).lower():
possible_persons.append(person)
if len(possible_persons) == 1:
return possible_persons[0]
if first_name == None:
for person in person_list:
if answer == str(person.first).lower():
return person
if last_name == None:
for person in person_list:
if answer == str(person.last).lower():
return person
try:
person_index = int(answer)
if person_index <= 0 or person_index > len(person_list):
raise IndexError
except ValueError:
print("\n ** {} wasn't even a number lmao **".format(answer))
continue
except IndexError:
print("\n ** {} wasn't even on the list lmao **".format(answer))
continue
return person_list[person_index-1]
elif len(person_list_exact) >= 2:
print("Found multiple persons who matched exact your criterias:")
return person_list_exact
my_address_book = AddressBook()
for match in line.finditer(data):
my_address_book.append(Person(**match.groupdict()))
my_address_book.add_person(Person(name="Boi", email=None, job=None, first="Boi", last="boiiiiiiiii"))
print("My address book:")
for person in my_address_book:
print(person.first)
Aaron Banerjee
6,876 PointsAaron Banerjee
6,876 PointsHi Flore, I by mistake did the extra credit challenge during the last code challenge of regular expressions because I was not thinking properly. I did it for a first name, last name, and score, but a twitter account ,phone number, and job can easily be added here by changing the players variable. Here is what I did