Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial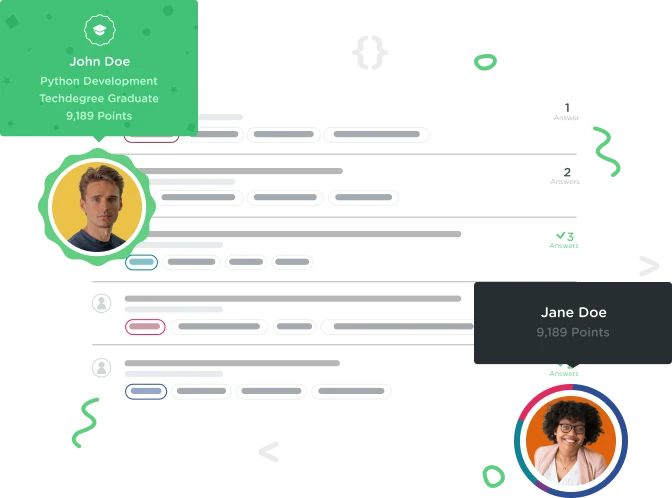

Alex Crump-Haill
7,819 PointsHave been struggling with this code challenge for days. Can someone tell me how to properly answer the 'Try and Except"?
This is for the third part of the challenge where you are asked for a bigger code writing section. I am not sure whether you are supposed to indent all code as per the function or if try (to check 'buggy' code) comes before the function. Also for return(None) is that meant to be return("None"). It is a really easy one but the right answer would be appreciated!
3 Answers
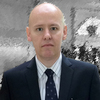
Nathan Tallack
22,159 PointsOk, it works like this.
def add(num1, num2): # Here we take in two args
try: # If anything inside this block errors the except block will run
f1 = float(num1) # Trying to change our first arg to a float
f2 = float(num2) # Trying to change our second arg to a float
except ValueError: # If any of the try block returns a ValueError this except block runs
return None # If it runs our function returns None
else: # If the try block threw no errors this else block runs
return f1 + f2 # We return the result of adding our two floats together
I hope this helps. Feel free to ask for any clarification if required. :)

Alex Crump-Haill
7,819 PointsHi Eric, agreed the answer provided by Nathan was very helpful and clear. In answer to your question, the whole point of inserting a 'try' followed by an 'except ValueError' is to catch an error if it happens to be input into the function by the user. In this case, a ValueError would pop up if the user input a str() and not an int().
For example, only in the circumstance that either num1 or num2 (or both) were not int()s would the function then fail to convert the expected int()s into float()s and a ValueError would occur. Hopefully this demonstrates that a ValueError occurs as a result of finding an error in the try block where num1 or num2 are incompatible with float() conversion!

Eric Chan
4,254 PointsHi Nathan, thanks for providing the answer to this challenge. What I do not understand is this: When the float() function convert whatever value of num1 and num2 into float, will there still be a ValueError? Thanks