Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial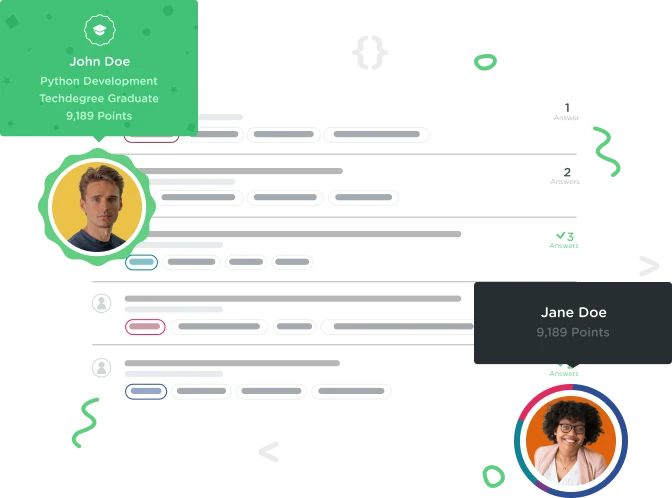

Tim Miller
781 PointsHave been stuck on this one for over two days.
I have watched the video 5 times and feel like I have used every combination possible and still am getting nowhere. I'm not asking for an answer, but any sway in the right direction would put me in a better place. This one has been beyond discouraging.
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
int lapsDriven = barCount - laps;
if (lapsDriven > MAX_BARS) {
throw new IllegalArgumentException("Not enough bars");
}
barCount = lapsDriven;
}
}
2 Answers
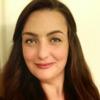
Jennifer Nordell
Treehouse TeacherHi there, Tim Miller! I can tell that you've tried and worked on this. In fact, I think you've probably overworked this a little. It happens to us all. At the beginning, that method contained a couple of statements that adjusted the laps driven by the laps we sent in and subtracted the laps that we sent in from the barCount.
Let's think about this for a minute. The maximum number of "energy bars" our car can have is 8. But let's say that I want to specify the number of laps at the time I tell it to drive
. So I say drive(5)
. That's going to drive 5 laps. That's all well and good because we're starting at fully charged. After it has driven those 5 laps, I'm expecting lapsDriven to be at 5 and the barCount to be at 3. We started at full but now we've driven 5 laps.
The problem could come when we call the drive method again and send in 4 laps. That's not going to work. We only have 3 bars left and we didn't charge it in between. The car is going to run 3 laps and then just become useless before the last lap. One way to disallow this is to throw a new IllegalArgumentException that alerts the person/dev trying to send the car on more laps than it has bars left for.
I'm going to toss some pseudocode up here:
public void drive(int laps) {
// if laps is greater than barCount {
// throw a new IllegalArgumentException
}
lapsDriven += laps; // this was in here from the start
barCount -= laps; // this was in here from the start
}
Remember that if an Exception is thrown, the method ceases execution right then. So if we were to send in too many laps, it would hit the exception and the laps would not be added to lapsDriven nor would it be subtracted from barCount. That will only happen if an exception isn't thrown.
Hope this helps!

Tim Miller
781 PointsYou definitely shined some light on a couple very dark days. Thank you 😃