Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial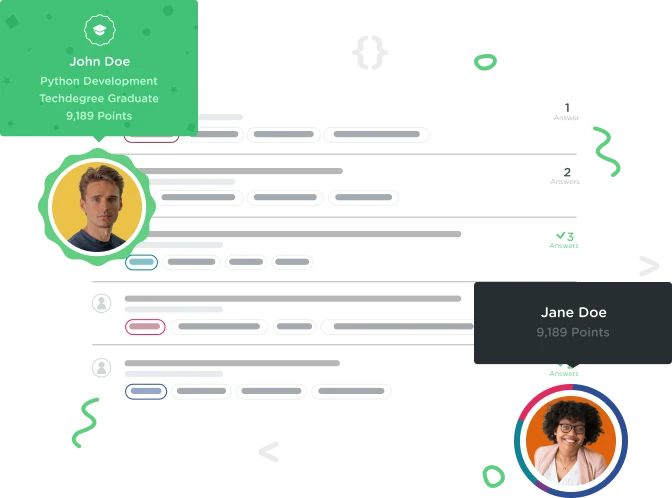

Andrei Oprescu
9,547 Pointshave I done something wrong?
Hi!
I had a challenge to complete and it asked me to do this:
That one wasn't too bad, right? Let's try something a bit more challenging. Create a new function named num_courses that will receive the same dictionary as its only argument. The function should return the total number of courses for all of the teachers.
I have completed the challenge with the code at the bottom of the question.
Can someone tell me the flaws in my code?
Thanks!
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(teacher_course):
return len(teacher_course)
def num_courses(teacher_course):
for key in teacher_course.keys():
return len(teacher_course.values())
6 Answers
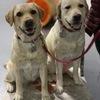
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Andrei,
The great thing about using .items() with a for loop instead of .keys() is that you get access to the values right in the body of the loop.
During each iteration of the loop, you have a variable value
that contains whatever value is associated with that dictionary item, just like you would have had access to the key
when you ran the loop with .keys().
Therefore to get a property of the value you just access value
directly. In our case, value
is a list, so you can do all the normal operations on a list right in your loop body.
E.g., Suppose we were iterating through the dictionary and the current key was 'Kenneth Love':
for key, value in teacher_course.items():
print(key) # would print 'Kenneth Love'
print(value) # would print ['Python Basics', 'Python Collections']
print(value[-1]) # would print the last element in the list, 'Python Collections'
Hope this clarifies everything for you.
Cheers
Alex
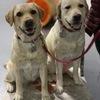
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Andrei,
To understand where you're going wrong with your function, remember what the return
statement does: it ends the function at that moment and sends back out of the function whatever value follows the return keyword (in your case len(teacher_course.values())
).
So let's work through the example dictionary in the challenge and see how that will play out. We pass the dictionary into the num_courses
function. We go into a for loop, let's say that we start the first iteration with key
being 'Andrew Chalkley'. The next line says return len(teacher_course.values())
so the function will end and the function will return with the length of all the values in teacher_course
: 2.
What we really want to do is accumulate a value over repeated cycles of the loop. This means we want to:
- define some variable to hold our accumulator before we go into the loop (e.g.,
running_total = 0
); - update the value of that accumulator while inside the loop;
- then after the loop finishes, return the final value of the accumulator (e.g.,
return running_total
). To get the right values during the loop, I would suggest that instead of just looping through the keys, you loop through the items (for key, value in teacher_course.items()
) which will give you access to each teacher's list of courses in each iteration of the loop. Hope that helps,
Cheers
Alex

Andrei Oprescu
9,547 PointsHi!
I have read your comment and I came up with this code:
def num_courses(teacher_course): courses = 0 for key, value in teacher_course.items(): courses += 1 return courses
Do I have to return a number of courses for each teacher or only all of the courses combined?
Can you give me some clue?
Thanks!
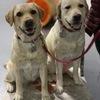
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Andrei,
As always it depends on what the question is asking for. In the real world circumstances will dictate whether you want a function to return a result based on a subset of the input or on the whole of the input.
In this case, let's see if Treehouse has given us any indication of what result they are looking for. The last line of the challenge says, "The function should return the total number of courses for all of the teachers."
It seems pretty clear based on this wording that the number they are looking for is the total number of courses. E.g., if there were 10 teachers and each teacher had 5 courses, it would be expecting the total number of courses: 10 * 5 = 50 as the answer.
Note that the number you are returning is the number of teachers, not the number of courses.

Andrei Oprescu
9,547 PointsHi again!
Can you please tell me a way to receive all of the values? I am struggling to count all of the values for a key.
The only idea I had was:
def num_courses(teacher_course):
courses = 0
for key, value in teacher_course.items():
courses += len(list(key))
return courses
Sorry for not understanding.

Andrei Oprescu
9,547 PointsOh, Thanks!
The solution is much more clear to me now that you explained that the value is a list.
Thanks!