Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial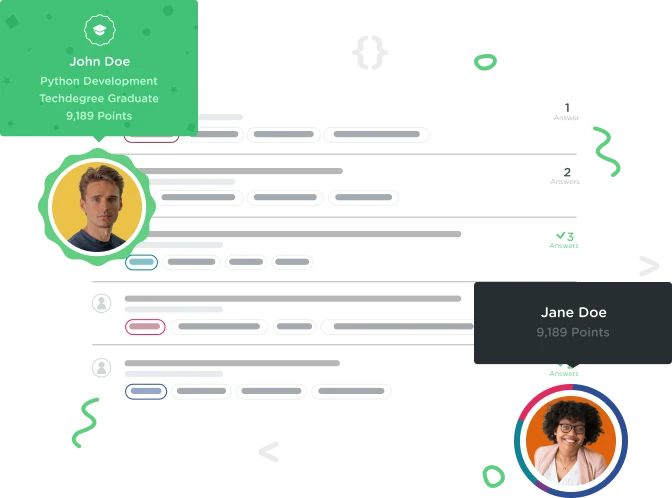
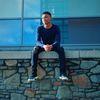
Nathan Marshall
6,031 PointsHave I done this correctly? I checked the console and it still brought up the error to a certain extent
function getRandomNumber(lower,upper) { if (lower||upper === isNaN) {
throw new Error("Please enter a number");
} var random = Math.floor(Math.random() * (upper - lower + 1)) + lower;
return random
}
alert(getRandomNumber("hi","nine" ));
2 Answers
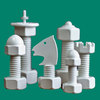
Steven Parker
231,268 PointsOne side of your logical expression was not a comparison, so the overall condition would be true based on the value of the "lower" argument. But to fix that, and to account for "isNaN" being a function, you could perform your test like this instead:
if ( isNaN(lower) || isNaN(upper) ) {
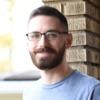
Justin Cantley
18,068 PointsI notice 2 issues with your code.
1)
You don't want to check if lower or upper are === to isNaN, because isNaN is a function which determines whether the argument you enter is not a number. You want that part of your code to look like this: if (isNaN(lower) || isNaN(upper))
2)
You are missing a semi-colon after your return random. That part of your code should look like this: return random;
The complete code should look more like this:
function getRandomNumber(lower,upper) { if (isNaN(lower) || isNaN(upper)) { throw new Error("Please enter a number"); } var random = Math.floor(Math.random() * (upper - lower + 1)) + lower; return random; }
Also in order to get a better idea of what's going on in your code (debugging) try using console.log() in every area of your code where it should be outputting.
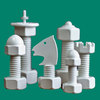
Steven Parker
231,268 PointsFYI: Unless you're in "strict" mode, semicolons are optional at the end of a line, though considered a "best practice".