Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial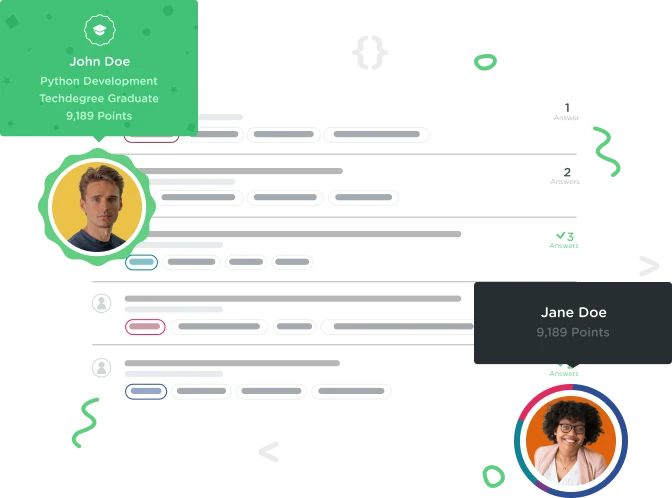

Ivan Saveliev
9,153 PointsHave I understood task incorrectly?
I thought we need to create a lists of questions that were answered correctly and incorrectly, but the solution is to count correct answers. That's what I did to print lists of questions answered correctly and incorrectly.
P.S. Sorry for absolute stupid questions.
// Array of questions with answers
var questionBox = [
['What is the Web school where you learn JavaScript?', 'Treehouse'],
['Do you like JavaScript so far?', 'Yes'],
['Are you going to continue learning JavaScript if you fail this challenge?', 'Yes']
];
// Array of correct and wrong answers array with index 0 = correctly answered, index 1 = incorrectly.
var answerBox = [
[],
[]
];
// handmade printer
function print(message) {
document.write(message);
}
// loop to get answers and store in corresponding arrays
for (var i = 0; i < questionBox.length; i++) {
var getAnswer = prompt(questionBox[i][0]);
if (getAnswer === questionBox[i][1]) {
answerBox[0].push(questionBox[i][0]);
} else {
answerBox[1].push(questionBox[i][0]);
}
}
// creates printable list of questions answered correctly
function printCorrectResult ( array ) {
var listCorrect = '<ol>';
for (var i = 0; i < array[0].length; i++) {
listCorrect += '<li>' + array[0][i] + '</li>';
}
listCorrect += '</ol>';
print(listCorrect);
}
// creates printable list of questions answered incorrectly
function printIncorrectResult ( array ) {
var listIncorrect = '<ol>';
for (var i = 0; i < array[1].length; i++) {
listIncorrect += '<li>' + array[1][i] + '</li>';
}
listIncorrect += '</ol>';
print(listIncorrect);
}
print('<h1> Questions answered correctly: </h1>');
printCorrectResult(answerBox);
print('<h1> Questions answered incorrectly: </h1>');
printIncorrectResult(answerBox);
1 Answer
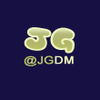
Jonathan Grieve
Treehouse Moderator 91,253 PointsThat's what the program does. It uses the 2 arrays to decide whether an answer is correct or wrong and tots the totals up in both arrays at the end of the program. It's a scorekeeper :)
Ivan Saveliev
9,153 PointsIvan Saveliev
9,153 PointsHi Jonathan, yep that's what I intended when I've written it. I thought that it was a task as per challenge instructions but Dave's solution does absolutely another thing, it just hows count of correct answers.
Ivan Saveliev
9,153 PointsIvan Saveliev
9,153 PointsI just realized that this task will be in the next challenge.