Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial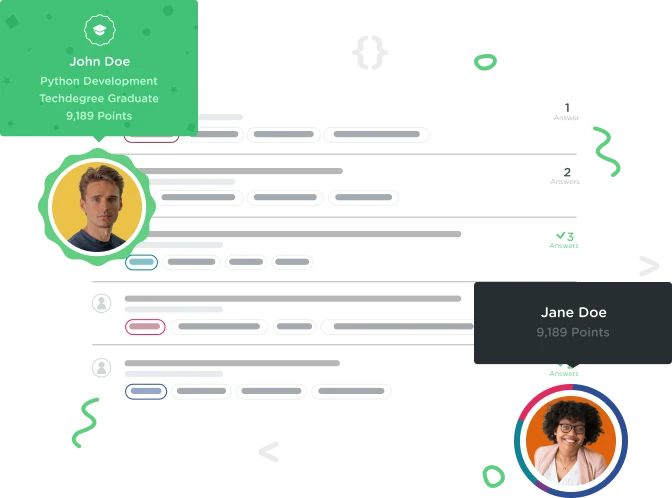

Matt Smith
878 PointsHave I used Init correct for this challenge? And what is my next step?
What is my next step and why?
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
// Add your code below
init(red: Double, green: Double, blue: Double, alpha: Double) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
}
}
2 Answers
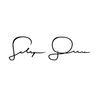
Filip Diarra
6,647 PointsI've been stuck on this one for a while now and can't get it to work. My code is:
init(red: Double, green: Double, blue: Double, alpha: Double, description: String) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
self.description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
and it still doesn't work. Can you guys help?
Thanks!

Art Hayes
7,251 PointsHi Filip,
I had a look at the Challenge... The problem appears to be that you've included the 'description' parameter in the init AND you're setting it within the init as well. Just remove the 'description' parameter from the init and you'll be good to go:
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
init(red: Double, green: Double, blue: Double, alpha: Double) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
self.description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
}
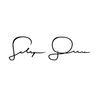
Filip Diarra
6,647 PointsHey Art,
Thanks for the answer! I don't understand why do we have to initialize the colors, but we don't initialize the description? I thought if I don't initialize the description, it won't work. Could you explain how come it works without being initialized?
Thanks!

Art Hayes
7,251 PointsSure, I think here's where you're confused... you don't init items in this line:
init(red: Double, green: Double, blue: Double, alpha: Double) {
This is where you're bringing in the parameters from within the application... but you are initializing the properties INSIDE the init brackets { }
Have a look in the brackets - you are setting all the properties there!
Here's another example... this is valid code as well (though it's not very useful in the real world)...
We're initializing the properties inside the init (but now you won't be able to send arguments into the init now when you initialize this struct because we removed the parameters from the init!)
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
init() {
self.red = 30
self.green = 210
self.blue = 0
self.alpha = 1
self.description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
}
Art Hayes
7,251 PointsArt Hayes
7,251 PointsHi Matt,
I'm not sure what challenge this is for... but here are a couple of hints.
1) With structs you get 'free' initializers, but if you create your own init you need to initialize ALL of the stored properties in the struct. When you take that into consideration and look up at your code, do you see one problem you might be having?
2) I highly suggest always keeping an open Playground session going in Xcode. It's a fantastic way to learn. (If you plug your code above into a Playground, it'll tell you what the error is) :)
EDIT: Matt - see my answer below.