Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial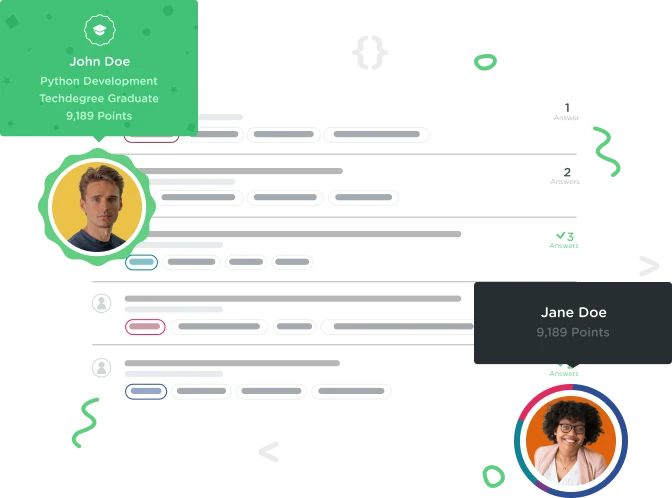
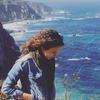
Deniz Kahriman
8,538 PointsHave no idea where to start. Can someone help/give an idea? Thanks!
Do I need to go back to the previous videos? Not sure how to proceed...
from dice import D6
class Hand(list):
def __init__(self, size=0, die_class=None, *args, **kwargs):
if not die_class:
raise ValueError("You must provide a die class")
super().__init__()
for _ in range(size):
self.append(die_class())
self.sort()
def _by_value(self, value):
dice = []
for die in self:
if die == value:
dice.append(die)
return dice
class CapitalismHand(Hand):
@property
def ones(self):
return self._by_value(1)
@property
def twos(self):
return self._by_value(2)
@property
def threes(self):
return self._by_value(3)
@property
def fours(self):
return self._by_value(4)
@property
def fives(self):
return self._by_value(5)
@property
def sixes(self):
return self._by_value(6)
@property
def _sets(self):
return {
1: len(self.ones),
2: len(self.twos),
3: len(self.threes),
4: len(self.fours),
5: len(self.fives),
6: len(self.sixes)
}
def roll(self):
1 Answer
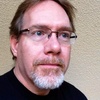
Chris Freeman
Treehouse Moderator 68,423 PointsAs it is now, CapitalismHand
has no __init__
method of its own so it runs the parentinit, which has a default
size` of 0.
How could you create a CapitalismHand.__init__
that overrides the parent but selects a size of 2?
Post back if you need more help. Good luck!!!
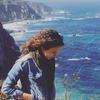
Deniz Kahriman
8,538 PointsThanks, Chris!!! Very helpful.
class CapitalismHand(Hand):
def __init__(self):
super().__init__(size = 2, die_class = D6)
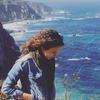
Deniz Kahriman
8,538 PointsI'm stuck again :/ So for the next challenge, we need to add a new property called doubles. It should return True if both of the dice have the same value. Otherwise, return False. Here is what I have but not sure why it's not working. I'm checking the list, dice.
@property
def doubles(self):
x = super()._by_value()
if x[0] == x[1]:
return True
else:
return False
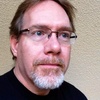
Chris Freeman
Treehouse Moderator 68,423 PointsThe _by_value()
method takes an argument value
that is used to determine which die with matching values exist in the Hand. In this challenge, for doubles you are looking to see if both die values match regardless of what the value is.
Since CapitalismHand
is a list of die
, you can reference each die directly as self[0]
, self[1]
. These can be compared simply with == and return the results. Shortcut, since the comparison yields a truth value it can be returned directly.
Also, for clarification, if _by_value()
had been part of the solution, you would not need to use super()
to call it. Since it is not overridden locally, it can be referenced using self._by_value()
.
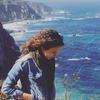
Deniz Kahriman
8,538 PointsAhh, ok, that makes perfect sense. Thanks so much, Chris!!! I sometimes confuse myself...
For the next challenge, I realized that I won't be able to use "self". My initial idea is below but of course, it isn't correct.
Q - if I have doubles, I want to reroll the hand. Add a classmethod to CapitalismHand named reroll that returns a new instance of the class, effectively rerolling the hand.
@classmethod
def reroll(cls):
if self[0] == self[1]:
cls.__init__()
else:
return cls.self
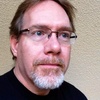
Chris Freeman
Treehouse Moderator 68,423 PointsThe method reroll
does not have to reconfirm that double had occured, it only needs to return a new CapitalismHand
object.
Be aware of the difference:
return cls.__init__() # fails since there is no instance, hence no "self"
return cls().__init__() # creates instance first, then calls __init__,
# but this also fails since __init__ returns None
# best to use
return cls()
The second example is what you want for `CapitalismHand.reroll()
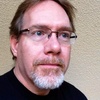
Chris Freeman
Treehouse Moderator 68,423 PointsMy last comment was updated to reflect an update in the challenge checker.
Deniz Kahriman
8,538 PointsDeniz Kahriman
8,538 PointsThank you so much, Chris! You really helped me understand how classes and instances work :)