Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial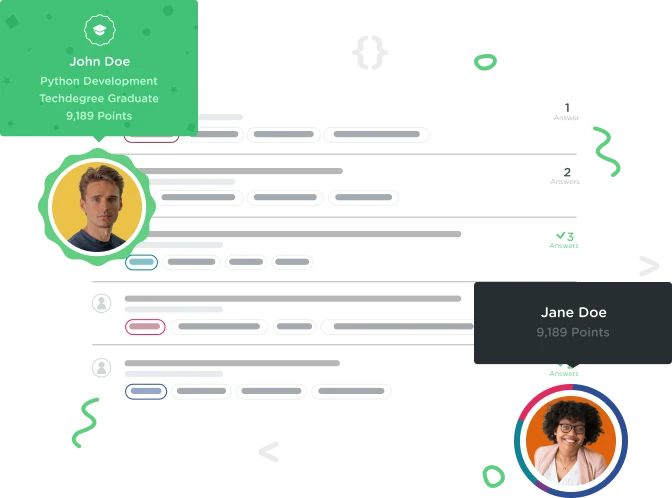

oz izhak
1,940 PointsHave one simple thing that i dont understand
import os
import random
import sys
words = [
'banana',
'orange',
'strewberry',
'cocunat',
'lime',
'kumquat',
'grapefruit',
'apple',
'lemon',]
def clear():
if os.name == 'nt':
os.system('cls')
else:
os.system('clear')
def draw(bad_guesses, good_guesses, secret_word):
clear()
print('Strikes: {}/7'.format(len(bad_guesses)))
print('')
for letter in bad_guesses:
print(letter, end=' ')
print('\n\n')
for letter in secret_word:
if letter in good_guesses:
print(letter, end='')
else:
print('_', end='')
print('')
def get_guess(bad_guesses, good_guesses):
while True:
guess = input("Guess a letter: ").lower()
if len(guess) != 1:
print("You can only guess a single letter")
elif guess in bad_guesses or guess in good_guesses:
print("You've already guess that letter!")
elif not guess.isalpha():
print("That not a letter, You can only guess a letters!")
else:
return guess
def play(done):
clear()
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while True:
draw(bad_guesses, good_guesses, secret_word)
guess = get_guess(bad_guesses, good_guesses)
if guess in secret_word:
good_guesses.append(guess)
found = True
for letter in secret_word:
if letter not in good_guesses:
found = False
if found:
print("You win!")
print("The secret word was {}".format(secret_word))
done = True
else:
bad_guesses.append(guess)
if len(bad_guesses) == 7:
draw(bad_guesses, good_guesses, secret_word)
print("You lost!")
print("The secret word was {}".format(secret_word))
done = True
if done:
play_again = input("Play again Y/n ").lower()
if play_again != 'n':
return play(done=False)
else:
sys.exit()
def welcome():
start= input("Press enter/return to start or Q to quit ").lower
if start == 'q':
print("Bye!")
sys.exit
else:
return True
print('Welcome to Letter Guess!' )
done = False
while True:
clear()
welcome()
play(done)
how the Shel system know it need to run the welcome() function first?? because i see that he write it as last function. i very confused about that. Actually my question is:
Although the functions all over the code doesn't arranged in the order, how Python or the OS know what to print 1st/2/3/4/5.............? and if thier any order, how i dicide what come first?
another thing,
the 'q' does not print Bye, it just start the game again.
tnx alot ! .
[MOD: added ```python formatting -cf]
5 Answers
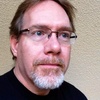
Chris Freeman
Treehouse Moderator 68,441 PointsHere's how I see the execution flow:
Import stuff; define a list, define functions; print "Welcome..." [1st print]; set done
to False
, then start the while
loop. It is the while
loop that determines the order of the function execution: clear()
, then welcome()
, then play(done)
.
In execution, welcome()
prints the prompt [2nd]. play()
will then call get_guess()
which will print prompt for guess [3rd]. The remaining printing depends on the flow caused by user responses.
> the 'q' does not print Bye, it just start the game again.
sys.exit()
is a function so it must be called with parens.
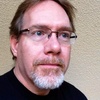
Chris Freeman
Treehouse Moderator 68,441 PointsAfter executing clear()
, and welcome()
, then play(done)
is executed. The flow stays within the while
loop of play()
until it reaches the sys.exit()
command. Occasionally, play()
will call the other functions defined above in the file, but the flow will fall back into play()
when the called function returns. So in the end, once within play
the code will basically stay until exit is reached.
Is this helping?

oz izhak
1,940 Pointsthe print and the while i understand . after that i confuse..:S
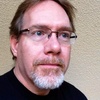
Chris Freeman
Treehouse Moderator 68,441 PointsCan you be more specific on what is confusing you?

oz izhak
1,940 Pointsafter the while that exeuction clear(), welcome(), then play(done) i just go up and start from draw and getting down that how i see it .

oz izhak
1,940 PointsVery much!! tnx Chriss, you help alot to understood one step more the code. Have great weekend !!