Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial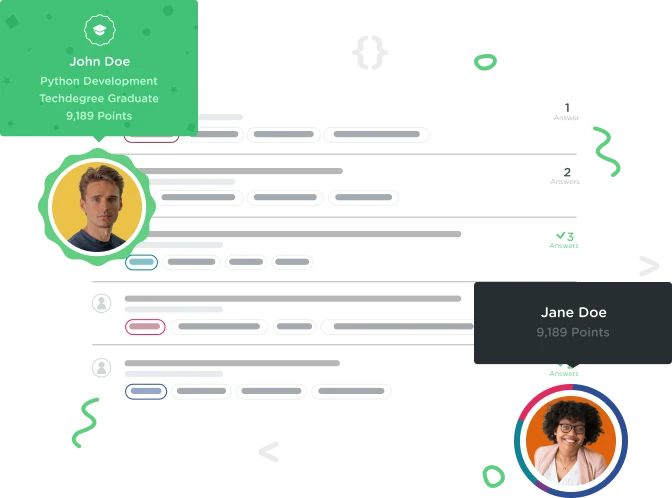

Patrick Thomas
Full Stack JavaScript Techdegree Student 3,529 PointsHaving a bit of trouble with this problem?
I think it's the way I have the code set up but I understand what the problem is asking me. If you can help me out that would be greatly appreciated!
function reformatName(text) {
const rawName = /^(\w+)\s(\w+)$/;
// Type your answer on line 5, below:
const newText = /^(\w)\s(\w)*/;
return text.replace(rawName, newText);
}
const form = document.querySelector("form");
const input = form.querySelector("input");
const reformatted = document.getElementById("reformatted");
form.addEventListener("submit", e => {
e.preventDefault();
reformatted.textContent = reformatName(input.value);
});
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<div id="content">
<form>
<label for="name">Enter your first and last name, please.</label>
<br />
<input type="text" id="name" name="name">
<button type="submit">Reformat</button>
</form>
<div>
<h2>Reformatted name:</h2>
<p id="reformatted"></p>
</div>
</div>
<script src="app.js"></script>
</body>
</html>
1 Answer
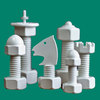
Steven Parker
231,275 PointsThe replacement string won't be a regex, and the tokens used to represent captured groups from the pattern are different from those used in a regex.
You might want to review the lesson and/or check the replacement string patterns on the MDN page for .replace.
Patrick Thomas
Full Stack JavaScript Techdegree Student 3,529 PointsPatrick Thomas
Full Stack JavaScript Techdegree Student 3,529 PointsHey, thanks for your help, I appreciate it. I still don't get what to do, I watched all the videos before the code challenge. I still need little guidance. Do you think you can help me?
Steven Parker
231,275 PointsSteven Parker
231,275 PointsSure, let's start with a 2nd try at the challenge. So given the hints I made and what you read on the MDN page and reviewed in the lesson, how might you construct the assignment for newText now?