Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial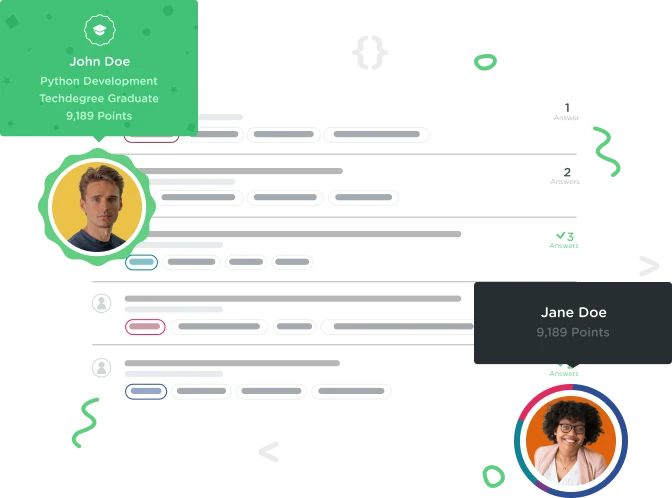
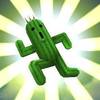
Sam Muir
16,745 PointsHaving a few issues with my app.js code. Some items stay where they are even after checking their checkbox?
Hi team,
I'm having a few difficulties with the additional questions towards the end of this course. My app.js file doesn't seem to toggle tasks from TODO to COMPLETED more than once? Below is my code - I've gone through the video's a number of times and I can't manage to figure out where it's gone wrong... I haven't been able to fix this issue, so I haven't been able to make a start on the next additional questions (Change the text from Edit to Save and vice versa).
Any help would be greatly appreciated.
Thanks,
//Problem: No user interaction produces the desired results
//Solution: Add interactivity so the user can manage daily tasks
var taskInput = document.getElementById("new-task"); //new-task
var addButton = document.getElementsByTagName("button")[0]; //new-button
var incompleteTasksHolder = document.getElementById("incomplete-tasks"); //incomplete tasks
var completedTasksHolder = document.getElementById("completed-tasks"); //completed tasks
//New task list item
var createNewTaskElement = function(taskString) {
//Create list item
var listItem = document.createElement("li");
//input (checkbox)
var checkBox = document.createElement("input"); //checkbox
//label
var label = document.createElement("label"); //label
//input (text)
var editInput = document.createElement("input"); // text
//button.edit
var buttonEdit = document.createElement("button");
//button.delete
var buttonDelete = document.createElement("button");
//Each element, needs to be modified
checkBox.type = "checkbox";
editInput.type = "text";
buttonEdit.innerText = "Edit";
buttonEdit.className = "edit";
buttonDelete.innerText = "Delete";
buttonDelete.className = "delete";
label.innerText = taskString;
//Each element needs appending
listItem.appendChild(checkBox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(buttonEdit);
listItem.appendChild(buttonDelete);
return listItem;
}
//Add a new task
var addTask = function() {
console.log("Add task...");
//Create a new list item with the text from the #new-task:
var listItem = createNewTaskElement(taskInput.value);
//Append listItem to incompleteTasksHolder
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
taskInput.value = "";
}
//Edit an existing task
var editTask = function() {
console.log("Edit task...")
var listItem = this.parentNode;
var editInput = listItem.querySelector("input[type=text]");
var label = listItem.querySelector("label");
var containsClass = listItem.classList.contains("editMode")
//if the class of the parent is .editMode
if (containsClass) {
//Switch from .editMode
//label text becomes the input's value
label.innerText = editInput.value;
} else {
//Switch to editMode
//input value becomes to the label text
editInput.value = label.innerText;
}
//Toggle .editMode on listItem
listItem.classList.toggle("editMode");
}
//Mark a task as complete
var taskCompleted = function() {
console.log("Complete task...")
//Append the task list item to the #completed-tasks
var listItem = this.parentNode;
completedTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
//Mark a task as incomplete
var taskIncomplete = function() {
console.log("Incomplete task...")
//When the checkbox is unchecked
//Append the task list item to the #incomplete-tasts
var listItem = this.parentNode;
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskIncomplete);
}
//Delete an existing task
var deleteTask = function() {
console.log("Delete task...")
var listItem = this.parentNode;
var ul = listItem.parentNode;
//Remove the parent list item from the ul
ul.removeChild(listItem);
}
//taskListItem = li of ul's
var bindTaskEvents = function(taskListItem, checkBoxEventHandler) {
console.log("Bind list item...")
//select list item's children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//bind editTask to edit button
editButton.onclick = editTask;
//bind deleteTask to delete button
deleteButton.onclick = deleteTask;
//bind checkBoxEventHandler to checkbox
checkBox.onchange = checkBoxEventHandler;
}
//Set the click handler to the addTask function
addButton.onclick = addTask;
var ajaxRequest = function() {
console.log("AJAX request");
}
addButton.onclick = ajaxRequest;
addButton.addEventListener("click", addTask);
addButton.addEventListener("click", ajaxRequest);
//cycle over incompleteTasksHolder ul list items
for(var i = 0; i < incompleteTasksHolder.children.length; i++) {
//for each list item
//bind events to list item's children (taskCompleted)
bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted);
}
//cycle over completeTasksHolder ul list items
for(var x = 0; x < completedTasksHolder.children.length; x++) {
//bind events to list item's children (taskIncomplete)
bindTaskEvents(completedTasksHolder.children[x], taskIncomplete);
}
1 Answer
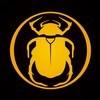
rydavim
18,814 PointsLooks like you've got your bindings transposed in your taskCompleted
and taskIncomplete
functions. You want to bind each list item to the function associated with the list you're moving it to, the opposite one.
// Mark the task as complete.
var taskCompleted = function() {
// . . .
bindTaskEvents(listItem, taskIncomplete);
}
// Mark the task as incomplete.
var taskIncomplete = function() {
// . . .
bindTaskEvents(listItem, taskCompleted);
}
The reason some of the items work and some of them don't, is that you've got it the right way round at the bottom when you're binding already existing items. Hopefully that helps, happy coding! :)