Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial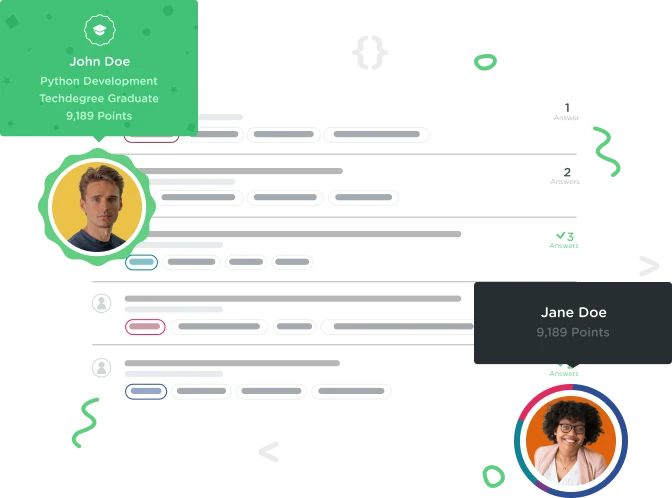

Kevin Jervis
884 PointsHaving a little trouble with this task. Started but not sure if I'm on the right track.
The task is below:
"Fix the getLineNumberFor method to return a 1 if the first character of lastName is between A and M or else return 2 if it is between N and Z."
Hopefully you guys can show me where I have gone wrong :) Many thanks
public class ConferenceRegistrationAssistant {
/**
* Assists in guiding people to the proper line based on their last name.
*
* @param lastName The person's last name
* @return The line number based on the first letter of lastName
*/
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
/*
lineNumber should be set based on the first character of the person's last name
Line 1 - A thru M
Line 2 - N thru Z
*/
if(lastName.charAt[0] => A && <= M)
return 1;
}
else(lastName.charAt[0] => N && <= Z)
return 2;
}
public class Example {
public static void main(String[] args) {
/*
IMPORTANT: You can compare characters using <, >. <=, >= and == just like numbers
*/
if ('C' < 'D') {
System.out.println("C comes before D");
}
if ('B' > 'A') {
System.out.println("B comes after A");
}
if ('E' >= 'E') {
System.out.println("E is equal to or comes after E");
}
// This code is here for demonstration purposes only...
ConferenceRegistrationAssistant assistant = new ConferenceRegistrationAssistant();
/*
Remember that there are 2 lines.
Line #1 is for A-M
Line #2 is for N-Z
*/
int lineNumber = 0;
/*
This should set lineNumber to 2 because
The last name is Zimmerman which starts with a Z.
Therefore it is between N-Z
*/
lineNumber = assistant.getLineNumberFor("Zimmerman");
/*
This method call should set lineNumber to 1, because 'A' from "Anderson" is between A-M.
*/
lineNumber = assistant.getLineNumberFor("Anderson");
/*
Likewise Charlie Brown's 'B' is between 'A' and 'M', so lineNumber should be set to 1
*/
lineNumber = assistant.getLineNumberFor("Brown");
}
}
1 Answer
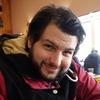
Eric M
11,546 PointsHi Kevin,
You're on the right track conceptually but there are a couple of issues with your code syntactically.
I've removed the comments and restyled using the Allman-8 format (putting braces on a second line and using 8 space indents), hopefully this makes it easier to see some of the issues.
public class ConferenceRegistrationAssistant
{
public int getLineNumberFor(String lastName)
{
int lineNumber = 0;
if(lastName.charAt[0] => A && <= M)
return 1;
}
else(lastName.charAt[0] => N && <= Z)
return 2;
}
Hopefully here you can see that your else clause is not within the getLineNumberFor
method. I wouldn't expect this to compile and it's certainly not going to do what you want if it did.
So, first off, let's fix that up.
public class ConferenceRegistrationAssistant
{
public int getLineNumberFor(String lastName)
{
int lineNumber = 0;
if(lastName.charAt[0] => A && <= M)
return 1;
else(lastName.charAt[0] => N && <= Z)
return 2;
}
}
While this looks a lot nicer (and has our code in the right places) we still have some issues.
Character literals in Java are enclosed in single quotes, e.g. 'a', 'b', 'c'. So your use of A, M, N, and Z will be interpreted by the compiler as referring to other variables within the program (e.g. A = 42521
) but these variables don't actually exist. So let's put quotes around these to make them character literals.
I can see what you're going for with charAt[0]
, but charAt
is a method of the String object, and methods get called like functions (e.g. charAt(0)
). This is actually a really easy mistake to make, and I will admit that not having used Java for six months I initially tried lastName[0]
for this challenge. It's great to understand that a String is an array of characters! While some languages let you access this array directly with array index notation, Java requires you to use the charAt()
method. This is because Strings in Java are somewhat more complex objects than the arrays of chars in other languages.
After your && operator you need to fully restate the condition. You can check anything after an AND or OR operator, it doesn't have to be related to the prior condition, so this means you need to be explicit. That is to say, we write if (a > b && b > c)
not if (a > b && > c)
Also, else
clauses don't take conditions, only if
and else if
do. So you'll need to either remove the condition or change to an else if
clause. If you change to an else if
you'll need to also add an else
at the end to return something in the event that none of the conditions match. Or you could restructure your program a little, for instance: mutating the provided variable in your conditional branches and then returning that.
Okay, so now we have:
public class ConferenceRegistrationAssistant
{
public int getLineNumberFor(String lastName)
{
int lineNumber = 0;
if(lastName.charAt(0) => 'A' && lastName.charAt(0) <= 'M')
return 1;
else if(lastName.charAt(0) => 'N' && lastName.charAt(0) <= 'Z')
return 2;
else
return 0;
}
}
if
and else
aren't functions, so we can go ahead and put a space between them and their conditions, this makes things a little nicer to read and is what other programmers will expect. Any code you write outside of learning exercises if likely to be read more often than written, even if by yourself, so keeping things neat and to convention can really save time in the long run. It'll become second nature very quickly too.
public class ConferenceRegistrationAssistant
{
public int getLineNumberFor(String lastName)
{
int lineNumber = 0;
if (lastName.charAt(0) => 'A' && lastName.charAt(0) <= 'M')
return 1;
else if (lastName.charAt(0) => 'N' && lastName.charAt(0) <= 'Z')
return 2;
else
return 0;
}
}
Okay! That passes.
If you're curious, here's how I did it:
public class ConferenceRegistrationAssistant
{
public int getLineNumberFor(String lastName)
{
int lineNumber = 0;
if (lastName.charAt(0) < 'N')
lineNumber = 1;
else
lineNumber = 2;
return lineNumber;
}
}
Kevin Jervis
884 PointsKevin Jervis
884 PointsHi Eric Thanks so much for taking the time to create such a detailed breakdown. The final solution is really clean and easy to read. I'm slowly getting to grips with the fundamentals of Java. I appreciate your help.
Andrew Crossley
6,244 PointsAndrew Crossley
6,244 PointsHi,
When using the above code I am getting illegal start of expression. It then shows me the area of the if and else if statements. Yet can not see why I am getting this illegal start of expression message.
Eric M
11,546 PointsEric M
11,546 PointsHi Andrew,
There is an issue in Kevin's code that I forgot to fix up that will cause that error. You won't get that error with the "here's how I did it" sample at the end of my post.
The caret (^) character actually points to exactly what's wrong. It is pointing at the illegal start of the expression. This is a pretty simple compiler error to fix so I encourage you to go back to it, really take a look at the code and maybe even rewrite it yourself from scratch before going onto my next paragraph. I highly suspect if you rewrite this code from scratch you will be able to write it without the error.
Okay, here it is: The issue is that the "greater than or equal to" operator in the conditional expression is malformed as
=>
instead of>=
, fixing that up will allow the last version of Kevin's code in my post to pass.Syntax errors similar to this are common and it's worth being able to debug them.
Cheers,
Eric