Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial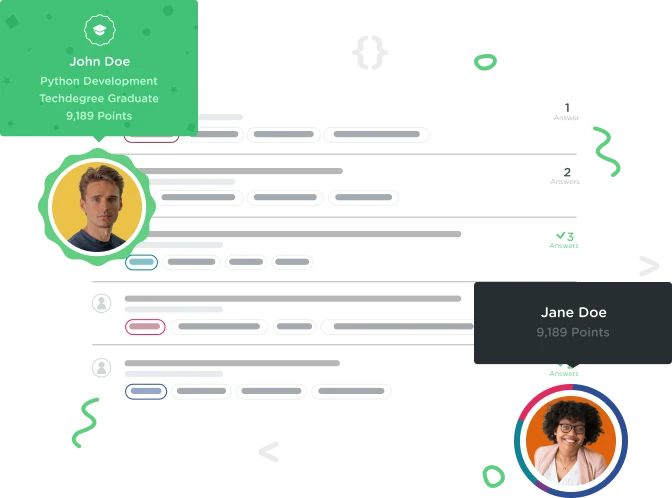

Nuradin Sheikh
6,211 PointsHaving an issue displaying the word "FizzBuzz" if it is a multiple of both 3 and 5?
I'm making a console application that follows the game fizz buzz. I managed to display the words "fizz" for multiples of 3 and "buzz" for multiples of 5 but can't seem to display "Fizz Buzz" if the number is multiples of 3 and 5. Can someone show me where I'm going wrong?
using System;
using System.IO;
namespace Practice
{
class Practice
{
public static void FizzBuzz()
{
for (int i = 1; i <= 100; i++) {
if (i % 3 == 0) {
Console.WriteLine("Fizz");
}
else if (i % 5 == 0)
{
Console.WriteLine("Buzz");
}
else if (i % 3 == 0 && i % 5 ==0) {
Console.WriteLine("both");
}
else
{
Console.WriteLine(i);
}
}
}
public static void Main(string[] args)
{
FizzBuzz();
Console.WriteLine("Press Enter to exist the application");
Console.ReadKey();
}
}
}
2 Answers
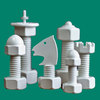
Steven Parker
229,744 PointsYou just need to re-order your tests so that the one for "both" is done first. As it is now, if either of the simple conditions is true, the test chain stops there and never gets to the combined test.
Also, if you actually want to display the word "FizzBuzz", you'll need to substitute it for "both" in the output statement.
And instead of "exist", you probably meant to write "exit".

Nuradin Sheikh
6,211 PointsThank you so I guess getting the order of the if statements right is important?
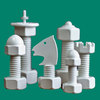
Steven Parker
229,744 PointsAn important part of program design is determining the order of operations. This is particularly important with statements that control the program flow, such as the "if" tests here.

Ariel Rzeszowski
5,482 PointsYea, ofcourse is very important. When if statment is executed, other else statments followed aren't executed because one part break another.
So when you iterate ex. "15" you will se only Fizz. If you want to get result what you expected you should to split this if-else statment to 4 small if statments
Nuradin Sheikh
6,211 PointsNuradin Sheikh
6,211 PointsI know I'm printing the word "both" instead of "Fizz buzz" even with that it still won't show up on the console application