Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial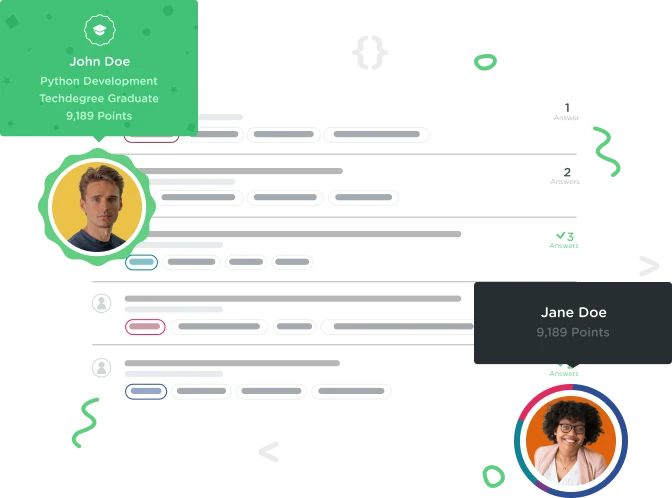
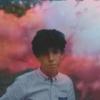
William J Kelly
2,935 PointsHaving compiler errors. I'm completely lost
Having a few problems with this challenge.
var europeanCapitals: [String] = []
var asianCapitals: [String] = []
var otherCapitals: [String] = []
let world = [
"BEL": "Brussels",
"LIE": "Vaduz",
"BGR": "Sofia",
"USA": "Washington D.C.",
"MEX": "Mexico City",
"BRA": "Brasilia",
"IND": "New Delhi",
"VNM": "Hanoi"]
for (key, value) in world {
// Enter your code below
switch world {
case "BEL": europeanCapitals.append("Brussels")
case "LIE": europeanCapitals.append("Vaduz")
case "BGR": europeanCapitals.append("Sofia")
case "IND": asianCapitals.append("New Delhi")
case "VNM": asianCapitals.append("Hanoi")
default: otherCapitals.append()
}
// End code
}
1 Answer

andren
28,558 PointsYour code is not too far off. The main issue is that you switch
on the world
array itself, rather than the key
of the array and that you aren't filling in a value for the otherCapitals
append method.
The for
loop that the switch
statement is inside of takes the world
array and separates it into two variables key
and value
. During the first loop key
equals the first key of the array (BEL) and value
the first value (Brussels), the second loop you get the second key (LIE) and second value (Vaduz) and so on.
Therefore you can use key
within the switch
to only compare things to the key, and you can also use value
to insert whatever value is currently being looped though.
So if you simply switch on the key
and append value
to the otherCapitals
array like this:
for (key, value) in world {
// Enter your code below
switch key {
case "BEL": europeanCapitals.append("Brussels")
case "LIE": europeanCapitals.append("Vaduz")
case "BGR": europeanCapitals.append("Sofia")
case "IND": asianCapitals.append("New Delhi")
case "VNM": asianCapitals.append("Hanoi")
default: otherCapitals.append(value)
}
// End code
}
Then your code will work. Though It's worth pointing out that the code can be simplified a bit. Since you can use value
to insert whatever value is being looped through you can condense the switch
into only 3 case checks. One that checks for European Capitals, one for Asian Capitals and one for other. Like this:
for (key, value) in world {
// Enter your code below
switch key {
case "BEL", "LIE", "BGR": europeanCapitals.append(value)
case "IND", "VNM": asianCapitals.append(value)
default: otherCapitals.append(value)
}
// End code
}