Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial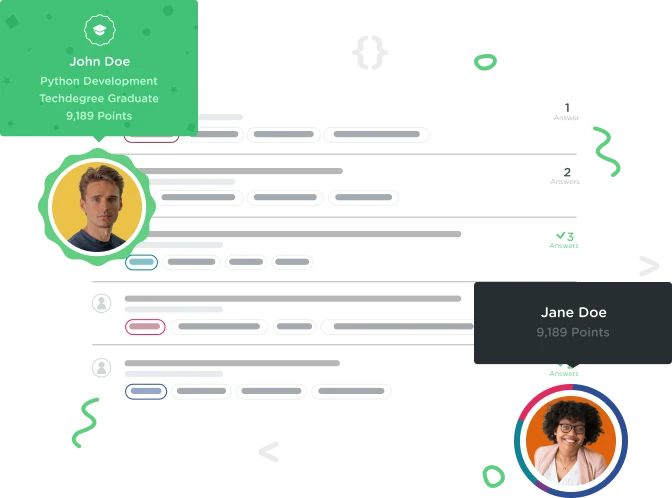
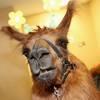
A X
12,842 PointsHaving Difficulty Understanding Object Literals in JS
Hi TH Community, Just diving into Dave's JS course on Object Literals, and he presented literals as an object that you can store information about your object into. Maybe it's because I've taken some of the SQL classes, but how is an object literal any different than creating a small database? Meaning that you're putting potentially a bunch of information in about 1 object...I liken it to describing all the parts that make up the human body and calling it an instance of a human object...but it's just information...so can someone describe simply to me what exactly an Object Literal is?
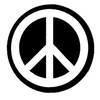
john larson
16,594 Pointsnekilof, I 'm wondering about SQL. I met a programmer that only works with SQL (and a php framework to manage it). He seemed to be gainfully employed and quite happy with his position. What's your impression of it?
1 Answer

Aaron Martone
3,290 PointsAn Object is simply a collection of key/value pairs. And yes, they both hold data. But a database is designed to do much more than hold data. It indexes it, provides optimizations to access, read, define in-depth meta about each field, restrict the values to data types (JS is a loose language, ie, it does not support strict data typing).
Simply put, an Object literal is a JS object, described in a literal method. What is a literal string? 'Hello, I am a literal String.'
. What is a String Object? var myStringObject = new String('Hello, I am a String Object');
. One was created via a 'literal' expression, the other via Object (because in JS, everything is an Object).
So I can create an object literal by using the following syntax:
var objectLiteral = {
myKey1: 'A string value',
myKey2: 23,
myKey3: true
};
That is a object literal. If I want to create that same Object, I can do so with the Object.create() method as well as defining its individual key names and properties, like:
var pureObject = Object.create(Object.prototype);
pureObject.defineProperties({
'myKey1': {
value: 'A string value'
},
'myKey2': {
value: 23
},
'myKey3': {
value: true
}
});
Literals are usually much less code to create.
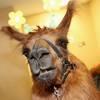
A X
12,842 PointsI much appreciate your examples, and that helps clear up the topic some for me.
Aaron Martone
3,290 PointsAaron Martone
3,290 PointsAs for object instantiation (making an instance of an object), the great thing you'll learn about JS is that, when done well, you can define properties on the object that are unique (like a
name
orage
of aPerson
object), but then you can use theprototype
to add common functions or properties. Defining them on theprototype
means they are inherited, but not defined directly on the object. This saves memory. Think of it this way:var Person1 = { name: 'John", age: 32, walk: function() { /* I am walking... */ }};
As we make more and more Persons with different names/ages, the
walk
function defined on it is duplicated, wasting memory. Down the line, when you want to define a shared method, you do it like so:Person.prototype.walk = function() { /* I am walking... */ };
And now, each
Person
object you create will have its ownname
andage
property, but awalk
function isn't defined ON the object. But due to JavaScript's prototypal nature, it will look up it'sprototype chain
when you ask for a method/property that isn't defined directly on the object. And using the aforementioned method, it will find thewalk
method and allow aPerson
object to use it.There's lots of great stuff about JS. We're all getting better at it step by step.