Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial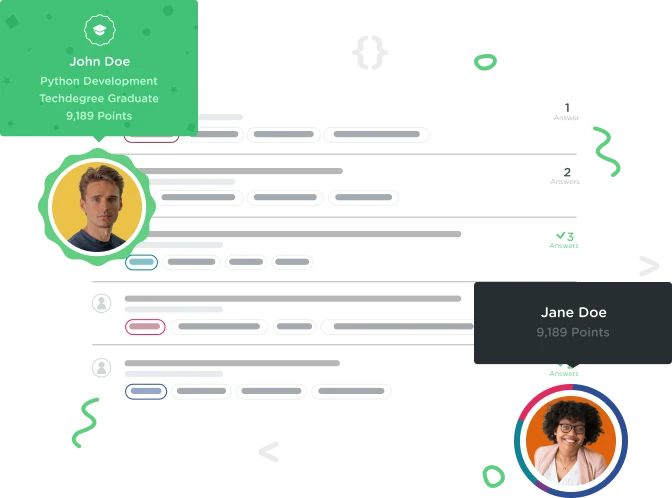

Steven Ossorio
1,706 PointsHaving issue with prepareForSegue. Please help
My PlayListMasterViewController.swift is
import UIKit
class PlaylistMasterViewController: UIViewController {
@IBOutlet weak var aButton: UIButton!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
aButton.setTitle("Press me!", forState: .Normal)
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject?) {
if segue.identifier == "showPlaylistDetail" {
let playListDetailedController = segue.destinationViewController as PlaylistDetailViewController
}
}
}
Currently I get an error on line 30 let playListDetailController = segue.destinationViewController as PlaylistDetailViewController. The error says:
'UIViewController' is not convertible to 'PlaylistDetailViewController';
did you mean to use 'as!' to force do...
In the lesson video there wasn't any error which would require an as!. Did I mess up my code somewhere that I haven't realized?
Incase it's required my PlayListDetailViewController.swift code is:
import UIKit
class PlaylistDetailViewController: UIViewController {
@IBOutlet weak var buttonPressedLabel: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
5 Answers

Nicoara Talpes
7,354 Pointsusing as! works for me.

Martin Wildfeuer
Courses Plus Student 11,071 PointsThis most likely means you have not set the custom class of your destination view controller in storyboard. Select your view controller in storyboard, bring up the Utilities tab (upper right) -> Identity inspector and insert your custom class name (PlaylistDetailViewController) in Custom class -> Class.
Let me know if that helps :)

Steven Ossorio
1,706 PointsHello Martin. Thank you for replying to my question. I checked my code today and it's already set as you've mentioned. When I select the originally view controller which has been renamed to PlaylistMasterController, the custom class is set to PlaylistMasterController. Same is set for the detail one such as PlaylistDetailViewController. Any ideas why it might be failing? if you need more info regarding what I've done thus far, please ask.
Thank you again for your help.

Martin Wildfeuer
Courses Plus Student 11,071 PointsHey everyone!
Let's give it a try, where do you end up with unwrapping? Yep or Nope?
if let playListDetailedController = segue.destinationViewController as? PlaylistDetailViewController {
print("Yep")
} else {
print ("Nope")
}

Steven Ossorio
1,706 PointsMartin Wildfeuer Thank you for your reply. I added the code you've mentioned but get a new error now.
override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject?) {
if segue.identifier == "showPlaylistDetail" {
if let playlistDetailController = segue.destinationViewController as? PlaylistDetailViewController {
print("Yep")
} else {
print ("Nope")
}
}
}
The error I get is for if let playlistDetailController which is "Immutable value 'playlistDetailController' was never used; consider replacing it with '_' or remove it"

Martin Wildfeuer
Courses Plus Student 11,071 PointsSure, we don't really use "playlistDetailController" by now, so Xcode issues a warning. That does not prevent you from running the app, though. So let's give it a try and let me know what the console output is :)

Alam Castillo
Courses Plus Student 505 PointsHave you found a solution for this yet? I am facing the same issue.

Steven Ossorio
1,706 PointsNone so far.

ian value
1,772 PointsHI Alam,
Did you ever figure this out? I'm having the same issue.

boris said
3,607 PointsI am also having the same issue. I tried using as! and it crashed. The breakpoint was on that line. Here is my code:
PlaylistMasterViewController
'''import UIKit
class PlaylistMasterViewController: UIViewController {
override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject?) {
if segue.identifier == "showPlaylistDetail" {
let playlistDetailController = segue.destinationViewController as! PlaylistDetailViewController
playlistDetailController.segueLabelText = "Something"
}
}
@IBOutlet weak var aButton: UIButton!
override func viewDidLoad() {
super.viewDidLoad()
aButton.setTitle("Press Me!", forState: .Normal)
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
'''
PlaylistDetailViewController:
'''
// // PlaylistDetailViewController.swift // Algorhythm // // Created by Mac Owner on 2/29/16. // Copyright © 2016 Boris Said Developtment. All rights reserved. //
import UIKit
class PlaylistDetailViewController: UIViewController {
@IBOutlet weak var buttonPress: UILabel!
var segueLabelText: String = ""
override func viewDidLoad() {
super.viewDidLoad()
buttonPress.text = segueLabelText
// Do any additional setup after loading the view.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
'''

boris said
3,607 PointsActually, the error appears to be happening on line 17
huckleberry
14,636 Pointshuckleberry
14,636 PointsAdded formatting to your code there for ya. In the future, wrap your code with 3 backticks (these ```... they're the key next to the 1) before and after your code. For language specific syntax, put the name of the language after the 3 beginning backticks.
here is a more detailed explanation of everything.
Cheers,
Huck -