Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial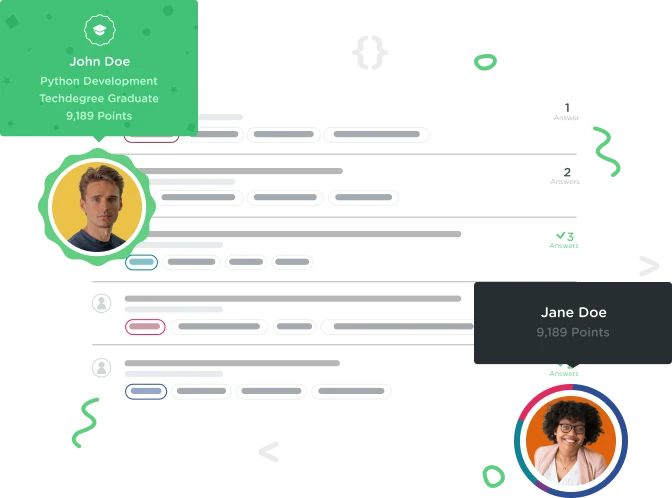
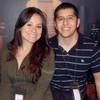
Pierre Ramirez
5,632 PointsHaving issues with this function returning a zero
So in this function I have an if statement where if it's blank then it shows zero and if it's not it shows length. Am I doing something wrong here?
function arrayCounter(arg1){
if(typeof arg1 === 'undefined'){
return 0;
}else{
return arg1.length;
}
}
arrayCounter();
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Foundations: Functions</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>JavaScript Foundations</h1>
<h2>Functions: Return Values</h2>
<script>
function arrayCounter(arg1){
if(typeof arg1 === 'undefined'){
return 0;
}else{
return arg1.length;
}
}
arrayCounter();
</script>
</body>
</html>
1 Answer
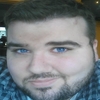
Marcus Parsons
15,719 PointsHey Pierre Ramirez,
You have to check for more than just undefined in your if statement. You need to also check for a string or number so your if statement needs to be expanded like so:
function arrayCounter(arg1){
//Check to see if arg1 is undefined, string, or number
if(typeof arg1 === 'undefined' || typeof arg1 === 'string' || typeof arg1 === 'number'){
return 0;
}else{
return arg1.length;
}
}
arrayCounter();