Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial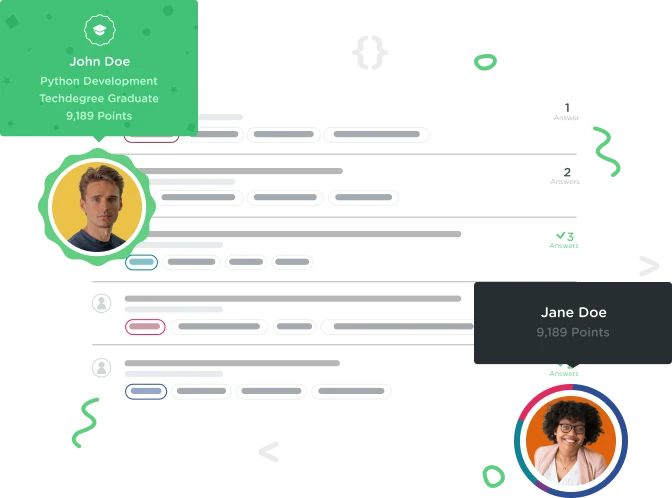
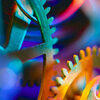
ja5on
10,338 PointsHaving JavaScript inside a table?
I have run my JavaScript code inside a table, so that table displays the JavaScript results of a set of random numbers, and although I get the desired effect I cant help but think I've used bad practise or I'm semantically wrong.
Please could someone provide some support.
1 Answer
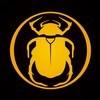
rydavim
18,814 PointsFrom a strictly JavaScript standpoint, there's nothing wrong with your code in my opinion.
However...
You should really only use HTML tables for tabular data, never for layout or formatting purposes. Given that you're outputting a <p>
element, I would say you should not be using a table. It would be better to handle the visual side of things using CSS.
There are quite a number of articles discussing this topic if you're interested in the web dev community's views on it.
Now, the other thing you could do in this case is make your JS output tabular data. Given that you are basically generating data that could be put into a table, it would be a reasonable alternative to format it that way. In that case, you'd probably want a column for tracking each roll (1, 2, 3...) and then a column for the results. I think either solution would be in keeping with good practices.
Some of these things are open to interpretation, and you won't always get the same answer from everyone regarding how you should structure your webpages and files. Hopefully this was at least somewhat helpful, but let me know if you have any lingering questions.
Good luck, and happy coding!
Update: Oct 10, 2020
You can find the sample code below, or you can use this workspace snapshot to play around with it.
If you're working with normal HTML elements like <p>
, you'll need to use CSS to get it to look the way you want. I didn't do very extensive work in the demo, so it could definitely be improved, but hopefully that gives you an idea of what I'm talking about. Of course, <table>
elements can also always use some CSS love.
My demo example may use some code that you're not familiar with yet, it depends where you are in your courses. I've tried to comment the parts that might be confusing, but let me know if there's stuff that's still not clear.
Note: When using a table, the table should contain data. This means there are few circumstances where you would want to insert a <p>
element into it. In this case, we're work with integers - so you can just insert them as that.
Example Code:
HTML
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
<link href="style.css" rel="stylesheet">
<script src="while.js"></script>
</head>
<body>
<main>
<h1>Random Numbers Between 1 and 10</h1>
<div id="cssLayout">
<h3>CSS Layout</h3>
</div>
<div id="tableLayout">
<h3>Table Format</h3>
<table id="randTable">
<tr>
<th>Count</th>
<th>Rand Num</th>
</tr>
</table>
</div>
</main>
</body>
</html>
CSS
body {
margin: 1rem;
padding: 1rem;
font-family: sans-serif;
}
div {
display: inline-block;
float: left;
margin-right: 2rem;
}
h1 {
padding: 0.5rem;
font-size: 2rem;
}
#cssLayout {
padding: 1rem;
background-color: #ADD8E6;
}
#tableLayout {
padding: 1rem;
background-color: #E6E6FA;
}
table {
border: 5px solid #778899;
}
th, td {
border: 1px solid #778899;
padding: 0.5rem;
}
tr:nth-child(even) {
background-color: #FAF0E6;
}
tr:nth-child(odd) {
background-color: #B0C4DE;
}
JavaScript
// window.onload ensures your html has loaded before executing the JS
window.onload = function() {
function getRandomNumber(upper) {
return Math.floor(Math.random()*upper) + 1;
}
let counter = 0;
while (counter<10) {
// create <p> and text node elements
let randElem = document.createElement("P");
let randText = document.createTextNode("Random Number " + (counter+1) + " is: " + getRandomNumber(10));
// append the text with random number output to your new <p> element
randElem.appendChild(randText);
// append the new <p> element to the layout div
document.getElementById("cssLayout").appendChild(randElem);
counter++;
}
// reset the counter to 0, since we're doing multiple demos
counter = 0;
while (counter<10) {
// select the HTML table
let table = document.getElementById("randTable");
// insert table rows and data for our random numbers
let row = table.insertRow(counter+1);
let cell1 = row.insertCell(0);
let cell2 = row.insertCell(1);
// fill in the data for which "roll" we're on, and what the random number is
cell1.innerHTML = counter+1;
cell2.innerHTML = getRandomNumber(10);
counter++;
}
}
Hope that helps, happy coding!
ja5on
10,338 Pointsja5on
10,338 Pointshttps://teamtreehouse.com/workspaces/41637132#
I got rid of the table and it just made things a lot worse.
I tried this way as well but I can't get to how your describing. - https://w.trhou.se/h9fbvt2oou
rydavim
18,814 Pointsrydavim
18,814 Pointsjasonj7 - I've updated my answer above with some more information, along with some example code and a workspace snapshot you can use to experiment. Let me know if you still feel like you're struggling, but I hope it helps! This was a great question to have.
ja5on
10,338 Pointsja5on
10,338 Pointsok thanks, but its way more advanced than id like.... plus I cant see how to incorporate the JavaScript file? I'm just gonna have to struggle along by myself. a lot of people show their programming might but I'm only after something simple. I'll go back to my original code... Btw you should've noticed my points compared to yours so it would be obvious to most that your code is way over my head making it not very helpful at all...