Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial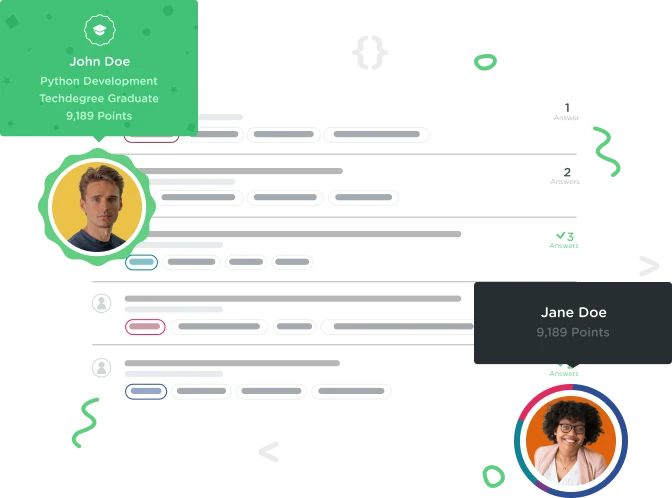
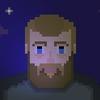
leonvsthings
12,236 PointsHaving problems understanding the shorthand version of the boolean value.
var correctGuess = false;
// we declare that correctGuess is false by default
var randomNumber = Math.floor(Math.random() * 6 ) +1;
var guess = prompt("Guess the number I'm thinking");
if (parseInt(guess) === randomNumber) {
correctGuess = true;
}
// we ask for user input, if the user is able to guess the number, switch is flipped, boolean is now true
if ( correctGuess ) {
document.write('<p>Correct.</p>');
}
// here, instead of saying if correctGuess === true - we use the shorthand and this confuses me.
else {
document.write('<p>Wrong</p>');
}
The usage of the shorthand correctGuess makes the code incoherent for me. As I commented in between the code, I understand why the code works as it is but I don't understand how we are able to create a shorthand that looks so vague on the surface. Can anyone give me any examples of advantages of using a shorthand rather than declaring the whole statement so I'll understand the reasoning behind.
4 Answers

Erik Nuber
20,629 PointsJust trying to break it down...
correctGuess can either be True or False (this is based on the code above assigning it either of these values)
in an if/else statement, it is looking for a boolean value which is a statement that is true or false
an if/else statement can always default to true by saying if(true) { do this }
so if we replace true here with correctGuess which could hold the value of true if(correctGuess) {do this}
as the code above is simplified for a single question and answer, correctGuess is set to false to begin with
because of this if (correctGuess) { will not do this} else {will do this}
but, as a question is asked and checked correctGuess can be changed to true.
ultimately, the code would be rewritten and correctGuess would be reset elsewhere to false or true based on the given answer.
by my understanding of what you are asking correctGuess is a declared value. It is defined and given a value both initially and changed based on a condition. So can be used. If you are more comfortable, there is no reason you can't say
if(correctGuess === true) {} but, it is not necessary.
as you may be suggesting by calling it a probe value, the program could technically crash if correctGuess were set to anything but true or false. However, as you are writing the code, you would know not to let the value be anything else, it is why it is declared as holding a value.
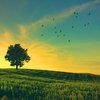
dbrink dbrink
6,258 PointsThis works because correctGuess was assigned the value of true, which makes this conditional the same as saying correctGuess === true ( and saying it the longer way would work, but would be redundant).
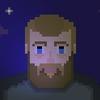
leonvsthings
12,236 PointsThe problem is, we are not just declaring that the correctGuess is true at the time. We're using this in a conditional. When the condition begins, how does the program know that it's true? Maybe the user gave us the wrong answer and the correctGuess was never turned into true.
The thing which makes me confused is the else statement. If we use the longhand correctGuess === true, we know that it's true and else will handle the false.
But when we use the shorthand in the conditional, we assume that the user triggered the true value, which might be or might not be true. I hope I was able to explain.

Erik Nuber
20,629 PointsNot sure if what you want to know is what correctGuess is really doing in the if/else statement.
The area between the ( ) is looking for a boolean answer, wether its 1 < 10 or ruby === ruby....correctGuess is either True or False so just having correctGuess in this statement makes it okay to use.
As D Brink said, it would be the same as writing if (true) {} or if (false) {}
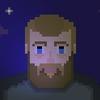
leonvsthings
12,236 PointsYeah as you said, the () area is not declaring an answer but it's looking for a boolean answer- meaning the answer can be more than 1 thing. What I'm asking is how can we make an if/else statement based on a probe of a value rather than a declaration of a value.

Erik Nuber
20,629 PointsGlad that helped and makes sense.
leonvsthings
12,236 Pointsleonvsthings
12,236 Points" an if/else statement can always default to true by saying if(true) { do this } "
Thanks Erik, this clarifies the problem for me! Great explanation.
Before this information, this was literally a Schrodinger's cat scenario for me.