Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial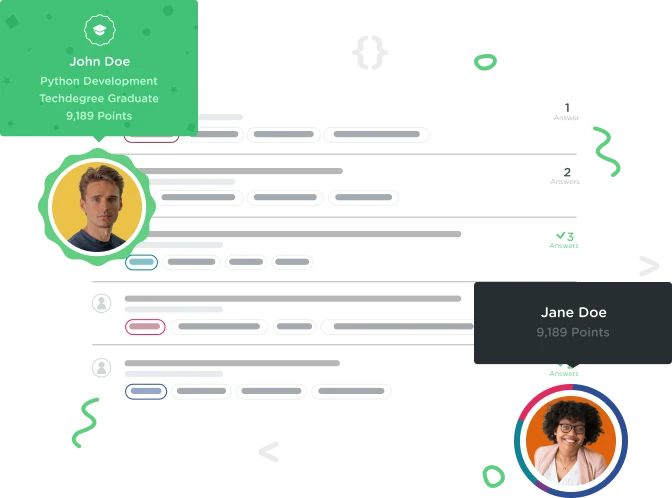

Justin Enayati
2,985 PointsHaving problems with Code Challenge: Introducing User-Defined Functions
Hey Guys,
I seem to be having issues with the entire challenge, except for the first task. which is just to create the function name.
now i simply thought i am just not understanding what i was doing, i watched the tutorials numerous times and have a pretty solid understanding of what i am trying to do.
just seems i cant pass the challenge, the confusing part of this is that i was able to pass the 2nd part once or twice, to then be stuck on part 3.
i then decided to restart the challenge to assure that i was "getting" what i was doing. just to fail part 2 again.
the wording of the challenge just seems to be a bit confusing on what is being asked from me.
from my understanding this is the code i tried to generate, although i either get a bummer! try again error or an error that says i have no value indicated in my mimic_array_sum. My guess is that i am a step ahead of the challenge in adding the $sum variable and the returning the $sum.
it seems like i was suppose to just add the foreach loop plus the variable to loop through the argument (($palindromic_primes as $palindromic_prime), if anything i'm confused by the part of just adding the return command without having the $sum variable declared.
<?php
function mimic_array_sum($array){
$sum = 0;
foreach($array as $number){
$sum = $sum + $number;
}
return $sum;
}
mimic_array_sum($palindromic_primes);
$palindromic_primes = array(11, 757, 16361);
?>
any help would be great ! ( looking for you Randy ;))
thanks!
10 Answers

Randy Hoyt
Treehouse Guest TeacherHere's the answer as I would write it:
<?php
function mimic_array_sum($array){
$sum_working = 0;
foreach($array as $number){
$sum_working = $sum_working + $number;
}
return $sum_working;
}
$palindromic_primes = array(11, 757, 16361);
$sum = mimic_array_sum($palindromic_primes);
echo $sum;
?>
You want to declare a variable inside the function and set it equal to zero before starting the foreach loop. It doesn't need to be called $sum
. Variables inside of a function are not accessible outside of the function, so you can call them whatever you want.
You could get the right output in this example by just echoing out the return value of the function like you did. But most of the time you'll need to do more than just echo out the return value from a function to the screen; you'll usually need to store the return value in a variable so that you can do more with it later in code. I wanted to make sure everyone had a good understanding of how to do that.
Does that help?

Christer Nordbø
2,133 PointsJust looking over your code - You are calling the function before it knows that the array exists.
Try moving:
mimic_array_sum($palindromic_primes);
To the bottom.

Christer Nordbø
2,133 PointsI just tried that code challenge myself! And it doesnt seem like its working properly!
I tried outputting something at all to the preview screen. That didnt work.
I created the full script on the page, and it didnt output anything in the preview. Pasted it to my localhost: worked flawless!
They might have some issues.

Justin Enayati
2,985 PointsHey Chris !
thanks for the reply, i did notice i did not place the call to the function after the array. good find! the task 2 seems to work properly for me and im guessing that was probably what i did to get pass the the other couple times.
but now my original problem insists,
task 3; <p>In the main code, outside of the function, use the new mimic_array_sum() function you just wrote. Store the return value in a variable called $sum, and then display that sum to the screen.</p>
my code already has $sum variable, i understand the challenge is asking me to place it outside my function, this is what is essentially missing for me to pass
<?php
function mimic_array_sum($array){
$sum = 0;
foreach($array as $number){
$sum = $sum + $number;
}
return $sum;
}
$palindromic_primes = array(11, 757, 16361);
mimic_array_sum($palindromic_primes);
?>
now do i need to make it: <?php
$sum = 0 //*declare sum*//
function mimic_array_sum($array);
foreach(yadda yadda)
$sum = //* decalre here*//
?>

Justin Enayati
2,985 PointsHey Chris,
I also noticed that the preview screen does not seem to work properly, which was confusing me to the point i was just using notepad++ to validate the code myself.
but before you had checked it and helped with the flaw i had, the error i was getting simply was that my argument was incorrect.
hope the guys at treehouse fix that little bug. if you have any solutions to my problem for task 3 of the challenge, please feel free to chime in
thanks guys!

Christer Nordbø
2,133 Pointstry printing the value of $sum in your function instead of returning the value! :)
OR
print out the return value of the function.
As the challenge doesnt work for me, i dont know the specifics of the code challenge.

Justin Enayati
2,985 PointsNo that didnt seem to do the trick . the error i seem to keep receiving is that my $sum variable is not showing a value .
at this point the $sum variable is not in the function and is declared outside of the mimi_array_sum( to my knowledge)
when i preview the page it gives the sum of all three numbers : 17129
code i entered for task 3:
<?php
$sum = 0;
function mimic_array_sum($array){
foreach($array as $number){
$sum = $sum + $number;
}
return $sum;
}
$palindromic_primes = array(11, 757, 16361);
echo mimic_array_sum($palindromic_primes);
?>
i have been stuck on this too long. any help would be great.
any teamtreehouse help would also be great !
thanks,
-justin

Randy Hoyt
Treehouse Guest TeacherHey Justin,
This last code snippet is almost there. Your mimic_array_sum()
looks good, though I'd recommend having $sum = 0
inside the function to start.
Here are the instructions for the last step:
In the main code, outside of the function, use the new mimic_array_sum() function you just wrote. Store the return value in a variable called $sum, and then display that sum to the screen.
This one line ...
echo mimic_array_sum($palindromic_primes);
... should first store the return value in a variable, like this ...
$sum = mimic_array_sum($palindromic_primes);
echo $sum;
Does that make sense?

Justin Enayati
2,985 Pointsyea that makes sense as to what your doing, but in regards to why your doing it i am confused Randy, why do i need to declare $sum =mimic_array_sum($palindromic_primes) outside of the function, when the function itself is returning the $sum of the of the array in with the loop? i am also still confused as to the placement of the declared value of $sum = mimic_array_sum($palindromic_primes) does this variable get placed after the function between the two curly brackets ? or im assuming its before you print out the command and list it next to the $palindromic_prime variable.
thanks for the help randy!
i look forward to your response asap.
-justin

Justin Enayati
2,985 PointsGotcha !
yes that makes much more sense. I guess the fact that i was just declaring the same name for the variable was confusing me, but as you stated the name makes no difference due to the function only being able to see the variable.
thank you sir!
-justin
Shawn Stewart
7,746 PointsShawn Stewart
7,746 PointsI was stumped on that last task, too. However, as it turns out I had already submitted my code for the second task as such:
Therefore, I kept trying to add to the code, getting more errors -- and more confused -- when all I needed to do was edit what I already had.