Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial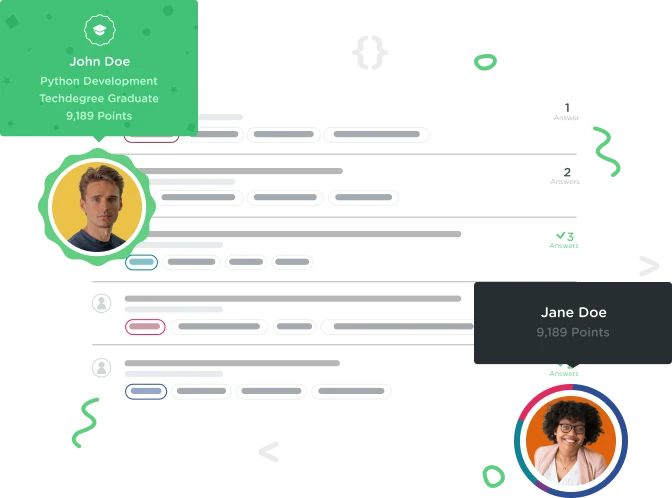

dlpuxdzztg
8,243 PointsHaving Trouble Adding Items to My Array
Hello.
So the scenario is I have a list of ListItem items that I'm displaying through a RecyclerView, I wan't them to be displayed as checkbox items (the only parameter they have is the parameter for the text of the checkbox.) When I try to add the item to the list however, I get an error:
AlertDialog.Builder builder = new AlertDialog.Builder(MainActivity.this);
View view = getLayoutInflater().inflate(R.layout.dialog_fragment_layout, null);
mConfirmTextView = (TextView) view.findViewById(R.id.confirmTextView);
mCancelTextView = (TextView) view.findViewById(R.id.cancelTextView);
mAssignmentEditText = (EditText) view.findViewById(R.id.assignmentEditText);
builder.setView(view);
final AlertDialog dialog = builder.create();
dialog.show();
mCancelTextView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
dialog.dismiss();
}
});
mConfirmTextView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String item;
item = mAssignmentEditText.getText().toString();
mItems.add(item); //The error is here
}
});
Here is the code for my ListAdapter:
public class ListAdapter extends RecyclerView.Adapter<ListAdapter.ViewHolder> {
private ArrayList<ListItem> mList;
private Context mContext;
public ListAdapter(Context context, ArrayList<ListItem> items) {
mContext = context;
mList = items;
}
@Override
public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext())
.inflate(R.layout.list_item_main, parent, false);
ViewHolder viewHolder = new ViewHolder(view);
return viewHolder;
}
@Override
public void onBindViewHolder(ViewHolder holder, int position) {
holder.bindHolder(mList.get(position));
}
@Override
public int getItemCount() {
return mList.size();
}
public class ViewHolder extends RecyclerView.ViewHolder {
public CheckBox mCheckBox;
public ViewHolder(View itemView) {
super(itemView);
mCheckBox = (CheckBox) itemView.findViewById(R.id.checkBox);
}
public void bindHolder(ListItem item) {
mCheckBox.setText(item.getAssignment());
}
}
}
Thank you!
1 Answer

Seth Kroger
56,413 PointsYou're adding a String to the list in the dialog handler but your adapter has a list of ListItems, which I assume is a custom model class of yours. You have a type mismatch as a result. You need to create a new ListItem from your string to add.
dlpuxdzztg
8,243 Pointsdlpuxdzztg
8,243 PointsI'm a little lost, what do you mean by 'create a new ListItem from your string to add'?
Seth Kroger
56,413 PointsSeth Kroger
56,413 PointsI'm assuming your ListItem has a constructor with a String argument for "assignment". or you can use the default constructor and use setAssignment()
dlpuxdzztg
8,243 Pointsdlpuxdzztg
8,243 PointsIt does have an empty constructor:
But in order to use setAssignment(), I need to create a ListItem object in my MainActivity. Should I do just that?
Seth Kroger
56,413 PointsSeth Kroger
56,413 Pointsyes