Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial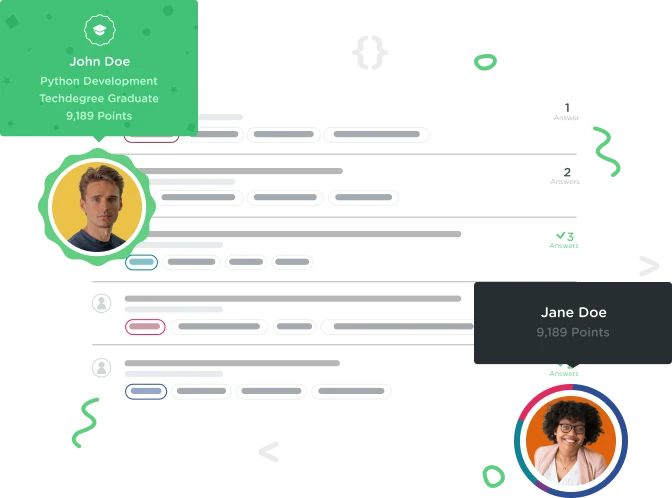

Joey Frazer
Courses Plus Student 129 PointsHaving trouble appending child.
I'm having trouble adding a child to a ul. Also is this the correct way to get it's input value?
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Todo List Application</title>
</head>
<body>
<h1>My Todo List</h1>
<input type="text" id="inputBox">
<ul>
</ul>
</body>
<script src="js/main.js" type="text/javascript"></script>
</html>
var input = document.getElementById('inputBox'),
ul = document.getElementsByTagName('ul');
input.addEventListener('change', function(){
ul.appendChild(input.value);
});
1 Answer
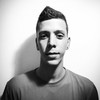
Jesus Mendoza
23,289 PointsHey Joey,
- To append a child element to another element you first have to create the element that you want to append.
- document.getElementsByTagName() returns an array, so, even if it only contains one element you have to access to it using [].
For example:
var input = document.getElementById('inputBox'),
ul = document.getElementsByTagName('ul');
/* We can pass an event argument (e) to our callback function
(which contains the event object that comes when you trigger
a new event, in this case "change". */
input.addEventListener('change', function(e){
// We create a new li element using createElement.
var li = document.createElement('li');
/* We set its innerText to the value of the input,
we can also access the event object passing it as an argument to
our callback function and then use e.target.value */
li.innerText = input.value;
ul[0].appendChild(li);
});
Good luck!
Joey Frazer
Courses Plus Student 129 PointsJoey Frazer
Courses Plus Student 129 PointsThank you so much!