Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial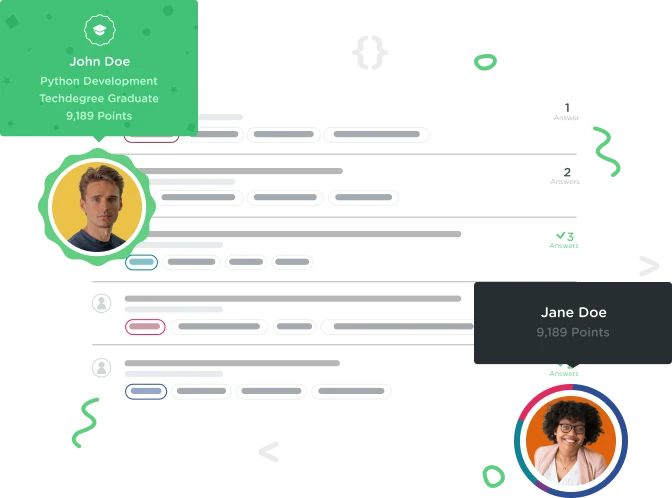
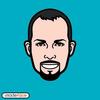
alborz
Full Stack JavaScript Techdegree Graduate 30,885 PointsHaving trouble getting Carrierwave to render the uploaded image
Hi! I'm running into some trouble getting the Carrierwave gem to render the uploaded image. It seems like the image is being uploaded, but when I click on 'show' in my application it doesn't render the image.
Here are some of my files:
image_uploader.rb
# encoding: utf-8
require 'carrierwave'
class ImageUploader < CarrierWave::Uploader::Base
# Include RMagick or MiniMagick support:
# include CarrierWave::RMagick
include CarrierWave::MiniMagick
# Choose what kind of storage to use for this uploader:
storage :file
# storage :fog
# Override the directory where uploaded files will be stored.
# This is a sensible default for uploaders that are meant to be mounted:
def store_dir
"uploads/#{model.class.to_s.underscore}/#{mounted_as}/#{model.id}"
end
# Provide a default URL as a default if there hasn't been a file uploaded:
# def default_url
# # For Rails 3.1+ asset pipeline compatibility:
# # ActionController::Base.helpers.asset_path("fallback/" + [version_name, "default.png"].compact.join('_'))
#
# "/images/fallback/" + [version_name, "default.png"].compact.join('_')
# end
# Process files as they are uploaded:
# process :scale => [200, 300]
#
# def scale(width, height)
# # do something
# end
# Create different versions of your uploaded files:
version :thumb do
process :resize_to_fit => [50, 50]
end
# Add a white list of extensions which are allowed to be uploaded.
# For images you might use something like this:
def extension_white_list
%w(jpg jpeg gif png)
end
# Override the filename of the uploaded files:
# Avoid using model.id or version_name here, see uploader/store.rb for details.
# def filename
# "something.jpg" if original_filename
# end
end
article.rb:
class Article < ActiveRecord::Base
validates :title, presence: true,
length: { minimum: 5 }
validates :text, presence: true,
length: { minimum: 5 }
mount_uploader :image, ImageUploader
end
views/articles/_form.html.erb:
<%= form_for(@article, html: { multipart: true }) do |f| %>
<% if @article.errors.any? %>
<div id="error_explanation">
<h2><%= pluralize(@article.errors.count, "error") %> prohibited this article from being saved:</h2>
<ul>
<% @article.errors.full_messages.each do |message| %>
<li><%= message %></li>
<% end %>
</ul>
</div>
<% end %>
<div class="field">
<%= f.label :title %><br>
<%= f.text_field :title %>
</div>
<div class="field">
<%= f.label :text %><br>
<%= f.text_area :text %>
</div>
<div class="actions"><br>
<%= f.submit %>
</div><br>
</div class="picture">
<%= f.file_field :picture %><br>
</div>
<% end %>
views/articles/show.html.erb:
<p id="notice"><%= notice %></p>
<p>
<strong>Title:</strong>
<%= @article.title %>
</p>
<p>
<strong>Text:</strong>
<%= @article.text %>
</p>
<h1><%= @article.title %></h1>
<p>
<%= @article.text %>
</p>
<%= image_tag @article.image_url(:thumb) %>
<%= link_to 'Edit', edit_article_path(@article) %> |
<%= link_to 'Back', articles_path %>
Thanks!
3 Answers
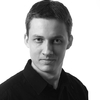
Maciej Czuchnowski
36,441 PointsPlease upload the app to github. This is not enough information.

Alex Stophel
iOS Development Techdegree Student 11,552 PointsHead into the rails console (rails c) and try some commands to see if you can get at the image url. I'd start with these:
a = Article.find(id_of_article_with_img)
i = a.image
p i
This should put the image object in the console for you to inspect. Do you see a URL? Try calling i.url
or i.thumb.url
to see if the property exists. Let us know if you run into any issues. I agree with Maciej Czuchnowski, though; push a branch to GitHub and we can take a better look.
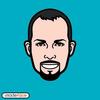
alborz
Full Stack JavaScript Techdegree Graduate 30,885 PointsHey guys sorry for the late response.
Here is my GitHub with the app pushed: https://github.com/amesbahi/travel_log
I tried Maciej's approach and I kept getting an error when I tried to "show" the article - it said undefined method 'url' for nil:NilClass.
Alex when I tried your suggestion I got the following without any errors:
irb(main):002:0> a = Article.find(2)
Article Load (0.1ms) SELECT "articles".* FROM "articles" WHERE "articles"."id" = ? LIMIT 1 [["id", 2]]
=> #<Article id: 2, title: "testing", text: "testing", created_at: "2015-03-25 20:34:53", updated_at: "2015-03-25 20:34:53", picture: nil>
irb(main):003:0> i = a.image
=> #<ImageUploader:0x007fdc584b7aa8 @model=#<Article id: 2, title: "testing", text: "testing", created_at: "2015-03-25 20:34:53", updated_at: "2015-03-25 20:34:53", picture: nil>, @mounted_as=:image>
irb(main):004:0> p i
#<ImageUploader:0x007fdc584b7aa8 @model=#<Article id: 2, title: "testing", text: "testing", created_at: "2015-03-25 20:34:53", updated_at: "2015-03-25 20:34:53", picture: nil>, @mounted_as=:image>
=> #<ImageUploader:0x007fdc584b7aa8 @model=#<Article id: 2, title: "testing", text: "testing", created_at: "2015-03-25 20:34:53", updated_at: "2015-03-25 20:34:53", picture: nil>, @mounted_as=:image>
irb(main):005:0> i.url
=> nil
irb(main):006:0> i
=> #<ImageUploader:0x007fdc584b7aa8 @model=#<Article id: 2, title: "testing", text: "testing", created_at: "2015-03-25 20:34:53", updated_at: "2015-03-25 20:34:53", picture: nil>, @mounted_as=:image>
irb(main):007:0> i.thumb.url
=> nil
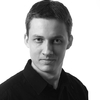
Maciej Czuchnowski
36,441 PointsI made a pull request that fixes your problem. Look through the changes and merge the request if you like it. Then just do git pull
on your machine to have the new code locally. Let me know if you have any questions. The code now works.
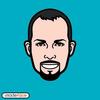
alborz
Full Stack JavaScript Techdegree Graduate 30,885 PointsThanks for the pull request!