Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial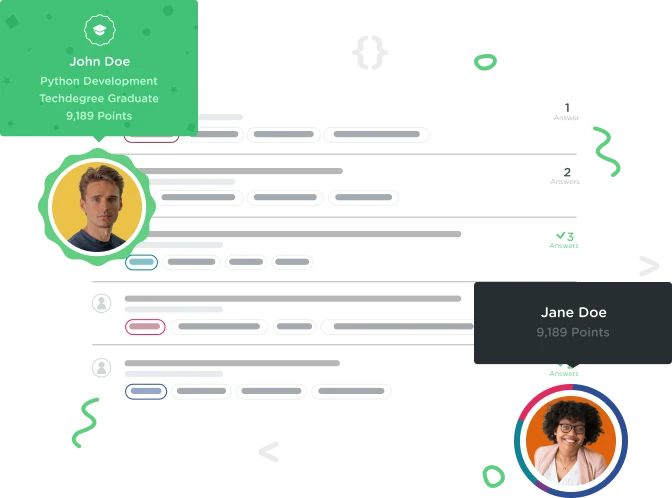

Kevin Lankford
1,983 PointsHaving trouble initializing mTags. Am I not adding the arguments correctly?
I'm getting an error thats saying there aren't the correct amount of arguments in the constructor. My idea of setting the constructor was to initialize the variables within the class. Am I not getting the concept of the task? Any help is appreciated, thank you.
package com.example.model;
import java.util.List;
import java.util.Set;
public class Course {
private String mTitle;
private Set<String> mTags;
public Course(String title, Set<String> tag) {
mTitle = title;
mTags = tag;
// TODO: initialize the set mTags
}
public void addTag(String tag) {
// TODO: add the tag
}
public void addTags(List<String> tags) {
// TODO: add all the tags passed in
}
public boolean hasTag(String tag) {
// TODO: Return whether or not the tag has been added
return false;
}
public String getTitle() {
return mTitle;
}
}
1 Answer
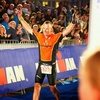
Steve Hunter
57,712 PointsHi Kevin,
To initialise the mTags
variable, you need to assign a new instance of HashSet
to it. You don't need to change the parameters that the constructor takes. Something like:
public Course(String title) {
mTitle = title;
// TODO: initialize the set mTags
mTags = new HashSet<String>();
}
Make sure you also import the HashSet
at the top of the code.
I hope that helps,
Steve.
Kevin Lankford
1,983 PointsKevin Lankford
1,983 PointsThank you! So in this perspective, am I establishing that I am using a collection of type Set when initializing in the class "Set<String> mTags;" and then specifying when I get into the constructor that I will be using a HashSet "mTags = new HashSet<String>();?
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHi Kevin,
The class definition has a space for its own content, so the template for each instance contains a memory space for the member variables. But this is just so the application can assess the overhead required for each created instance. If you never create an instance, the compiler will allocate no memory for the class.
The constructor, in this example, initialises the
mTags
variable, because that's the task this challenge wants you to complete. So, the memory overhead (hole) has been created because the compiler knows what an instance of each class needs. Then, the constructor here has created anmTags
instance ofSet
and assigned that to the memory hole.You are then free to use the Set as you wish.
Creating the declaration in the class definition isn't the same as initialising it. The member variables are declared in the class. In this case, you initialise the
mTitle
with a value by passing a value into the constructor. ThemTags
member variable is initialised with a blank instance ofHashSet
, ready to be used later. The memory management of that occurs outside of theCourse
class.I hope that made some sense!
Steve.