Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial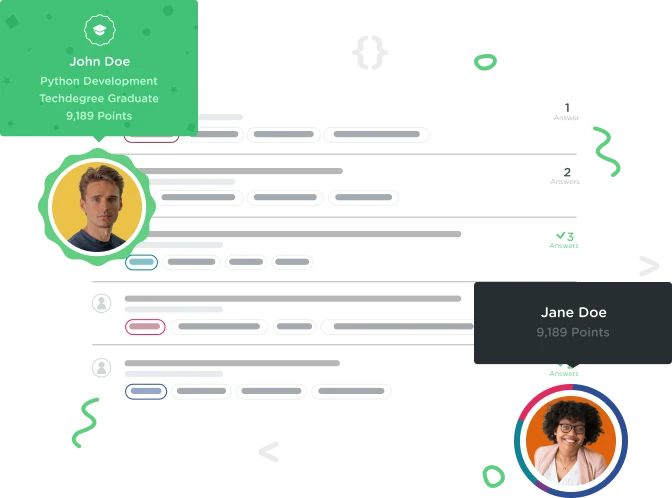
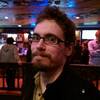
Chris Andruszko
18,392 PointsHaving trouble showing and hiding...
I'm creating super simple apps just for practice. The apps allow a user find the areas of certain shapes. The user can select what shape they want to find the area of using radio buttons.
The apps are hidden on page load using this code:
$(document).ready(function(){
$("#triangle").hide();
$("#rectangle").hide();
$("#circle").hide();
});
Here are the radio buttons:
<h2>I need to find the area of a:</h2>
<form>
Triangle <input type="radio" name="A" id="triangle_radio" onClick="showTriangle();" />
<br>
Rectangle <input type="radio" name="A" id="rectangle_radio" onClick="showRectangle();" />
<br>
Circle <input type="radio" name="A" id="circle_radio" onClick="showCircle();" />
</form>
When a radio is selected, the corresponding app appears on the page using the function associated with its onClick event:
function showTriangle() {
$("#triangle").show();
}
function showRectangle(){
$("#rectangle").show();
}
function showCircle(){
$("#circle").show();
}
This works pretty well. But if the user clicks on all the radio buttons, all the apps show on the page (even though only one radio is selected at a time). I want the apps to show one at a time. So if the triangle app's radio is selected, it appears. Then, if the rectangle radio is selected, that appears and the triangle app disappears.
I tried to accomplish this with the triangle app. I got rid of its onClick event, and wrote a conditional statement:
if($("#triangle_radio").attr("checked") == "checked") {
$("#triangle").show();
}
This did not work. Now the triangle app's radio button does nothing.
So how do I make each app appear one at a time, and disappear when its radio is no longer selected?
I hope I explained my problem decently. I'm still quite new to all this, so sometimes I even have trouble explaining it to myself.
I created a CodePen of the entire web page in its current condition: http://codepen.io/sketchcrush/pen/OPmMwe
As you'll see, the rectangle and circle apps appear when their radio is selected. The apps calculate just fine. But the triangle app (the one with the conditional statement) does nothing when you select its radio.
Any help is greatly appreciated.
-Chris A.
1 Answer
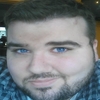
Marcus Parsons
15,719 PointsHey Chris Andruszko,
The easiest way to do this is to hide all other divs when the current one is clicked. There are a number of different ways to do this. But, I found a very easy way (with some very slight modifications) that will allow to you add as many "area" finders (or other such selectors as you wish). All I did was change the first "div" where all of the radio buttons are housed to a "section" element (which will act the very same way as a div, just with a different name). Then, added a line of jQuery to each existing show functions:
<!-- changed div element to a section element and added onclick handler for triangle-->
<section class="backing">
<h2>I need to find the area of a:</h2>
<form>
Triangle <input type="radio" name="A" id="triangle_radio" onClick="showTriangle();" />
<br>
Rectangle <input type="radio" name="A" id="rectangle_radio" onClick="showRectangle();" />
<br>
Circle <input type="radio" name="A" id="circle_radio" onClick="showCircle();" />
</form>
</section>
function showRectangle(){
$("#rectangle").show();
//Hide all other divs that are siblings of this selector
$("#rectangle").siblings("div").hide();
}
function showCircle(){
$("#circle").show();
//Hide all other divs that are siblings of this selector
$("#circle").siblings("div").hide();
}
//Changed to a function instead of checking for attribute
function showTriangle() {
$("#triangle").show();
//Hide all other divs that are siblings of this selector
$("#triangle").siblings("div").hide();
}
I hope that helps.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsOh and here is the codepen with the updated code.
Chris Andruszko
18,392 PointsChris Andruszko
18,392 PointsPerfect! Thanks so much! Like I said, I'm still very new to this, so I didn't even think about doing it the way you did it. I'm always happy to learn something new.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsAnd I'm always happy to help! I'm always learning myself, because when we stop learning, we stop growing. And I think that helping others allows you to not only retain knowledge better but give your brain a bit of a workout, too. haha :)