Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial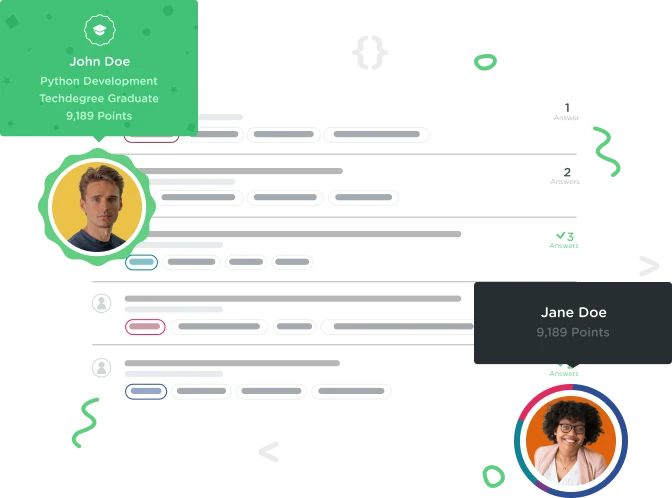

Eric Shelby
28,300 PointsHaving trouble understanding the instructions.
The instructions states that I am supposed to write code that will cause a class of "highlight" to be added to the paragraph element immediately preceding that button inside the parent list item element after the button is clicked. However, I have no idea what that is suppose to look like. Is it suppose to make the paragraph element move up or down or something suppose to happen behind the scenes? I can probably write the code; I just need to know what the final outcome is suppose to be.
const list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
let li = e.target.parentNode;
let hl = li.previousElementSibling;
let ul = li.parentNode;
ul.insertBefore(li, hl);
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>Things to Learn</p>
<ul>
<li><p>Element Selection</p><button>Highlight</button></li>
<li><p>Events</p><button>Highlight</button></li>
<li><p>Event Listening</p><button>Highlight</button></li>
<li><p>DOM Traversal</p><button>Highlight</button></li>
</ul>
</section>
<script src="app.js"></script>
</body>
</html>
2 Answers
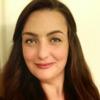
Jennifer Nordell
Treehouse TeacherHi there! When you are looking at the challenge you can enter the "preview" mode and then go back to the "editor" mode at will. Keep in mind, though, that it will often take a few seconds to load the preview. On the preview you will find two tabs: app.js and index.html. It's the index.html
that you're interested in for viewing the results.
It's my belief that there's a CSS file here that we simply can't see. When you click the "Highlight" button, the text immediately to the left of the button should be highlighted. The "highlight" class from the CSS we don't have access to should be applied. This will apply a dark green background with rounded corners and yellow text to that paragraph.
If you want to see what this is going to look like, you can manually add class="highlight"
to one of the paragraphs inside the HTML and then preview it. Make sure to remove that though after you've done the code that will apply the class programmatically.
Hope this helps!

Eric Shelby
28,300 PointsThanks for your input. It helped clear up what I had to do. I never had a problem where I had to add a class to an element using another program before and didn't know what to do. It still took a little while to finally solve the problem, but the input helped steer me on the right path.
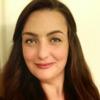
Jennifer Nordell
Treehouse TeacherThat's great! Glad I could help. That being said, it's sort of the beauty and the beast of programming. Sometimes you just need to know the path to take, but ultimately, it's up to you to use the documentation to tell you how to get down that path. It's almost never about memorization but rather how to take this idea and apply it to that problem