Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial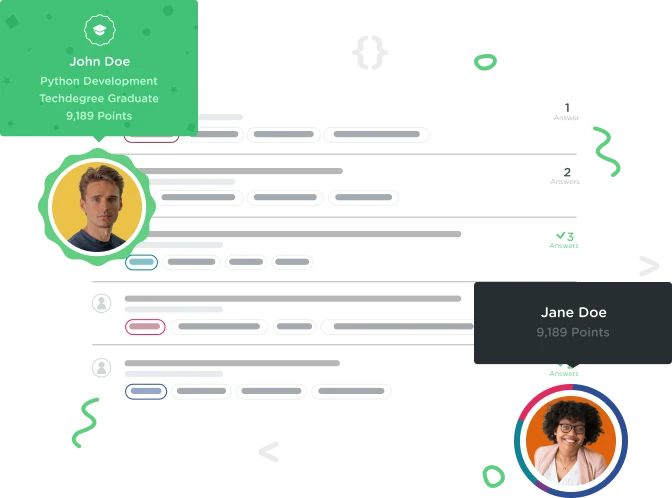
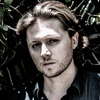
Richard Nash
24,862 PointsHaving trouble understanding this code challenge. Please help...
This is confusing me quite a bit... I feel like I'm close, but I'm missing something conceptually.
Here is the question:
Around line 17, create a function named 'arrayCounter' that takes in a parameter which is an array. The function must return the length of an array passed in or 0 if a 'string', 'number' or 'undefined' value is passed in.
And here is my code:
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Foundations: Functions</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>JavaScript Foundations</h1>
<h2>Functions: Return Values</h2>
<script>
function arrayCounter(array) {
if (typeof array === 'string', 'number', 'undefined') {
return 0;
} else {
return array.length;
}
}
</script>
</body>
</html>
If you can spot what I'm missing I would really appreciate it. Thank you.
I found an answer that worked here: https://teamtreehouse.com/forum/unable-to-get-it-through
but most of the information in the answer has not been introduced yet in the course, so I do not understand the information very well. Here is the answer that worked:
function arrayCounter (list) {
if (typeof list == "string" || typeof list == "number" || typeof list == "undefined") {
return 0;
} else {
return list.length;
}
}
the tall vertical lines the why the term list was chosen over array and separating everything out is rather confusing to me at this point. Also, is it trying to count the number of items in the array of the individual characters? The following array [ 123, 456, 789 ] 3 items and 9 characters... confused about this.
Also confused about the parameter term. Are arguments and parameters the same thing in this situation?
And can I not only have a 'string' or a 'number' or another variable or function inside of a function?
2 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Richard,
The reason that your if condition isn't working out is because of operator precedence and also how the comma operator works.
The following link contains a table listing operator precedence:
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Operator_Precedence#Table
You have 3 operators in your condition - typeof
, ===
, and ,
From that table we can see that the typeof
operator has highest precedence (4), the equality comparison operator ===
is next highest (9) and the comma ,
operator has the lowest precedence of all (18).
So the typeof
operator has to be evaluated first in the following condition:
if (typeof array === 'string', 'number', 'undefined') {
typeof array
will return some string indicating the type of variable it is, let's say 'number'
Now we have:
if ('number' === 'string', 'number', 'undefined') {
The ===
is evaluated next. 'number' === 'string'
will be false
since those strings are not equal. However, it could be true
if typeof
had returned a string type.
Now we have:
if (false, 'number', 'undefined') {
Now the comma operator can finally be evaluated. The way this works is that each comma separated expression is evaluated (if needed) and then the last expression is returned. So this false, 'number', 'undefined'
is going to become 'undefined'
when it is evaluated because 'undefined' is the last expression.
Now we're down to this:
if ('undefined') {
'undefined' is then converted to a Boolean
which becomes true
So you end up with:
if (true) {
This means your function will always return 0 regardless of what you pass into it.
In the code you found, the two pipe characters ||
are for the logical OR operator. You'll also find that in the table linked above (#14)
You could read this code if (typeof list == "string" || typeof list == "number" || typeof list == "undefined") {
in english as "if the type is 'string' OR the type is 'number' OR the type is 'undefined'" So if any of those 3 boolean expressions are true then the whole thing is true and you'll end up returning 0. This may seem more verbose to you than what you were trying to do but it's necessary to make the logic work out right.
Since I don't think this was covered in the course you can also write it out like this:
if (typeof list == "string") {
return 0;
}
if (typeof list == "number") {
return 0;
}
if (typeof list == "undefined") {
return 0;
}
return list.length;
This is more code to write and is repetitive but should be more inline with what you've learned up to this point.
The other questions at the end -
The parameter can be any valid variable name. You used 'array' the other person used 'list'. It's whatever variable name you want to use.
The purpose of the function is to count the number of elements in an array, not how many characters.
Informally, parameters and arguments can be used somewhat interchangeably. More formally, parameters are the variables used as inputs in the function definition whereas arguments are what you pass into the function when you're calling it.
So in your code, array
is a parameter for the function.
When you call it like this:
var ages = [34, 59, 18];
arrayCounter(ages);
ages
is an argument to the function.
I can't make out what your very last question is asking.

Roberto Alicata
Courses Plus Student 39,959 PointsThe "||" means a boolean OR condition.
Then the "return list.lenght" return the number of items in the array
Arguments and parameters are the same thing in this context.
Richard Nash
24,862 PointsRichard Nash
24,862 Pointswow... you rock jason! thank you :-) And Laura helped me with the last question, which was basically concerning the use of vocabulary, as I was getting my wires crossed a little bit.