Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial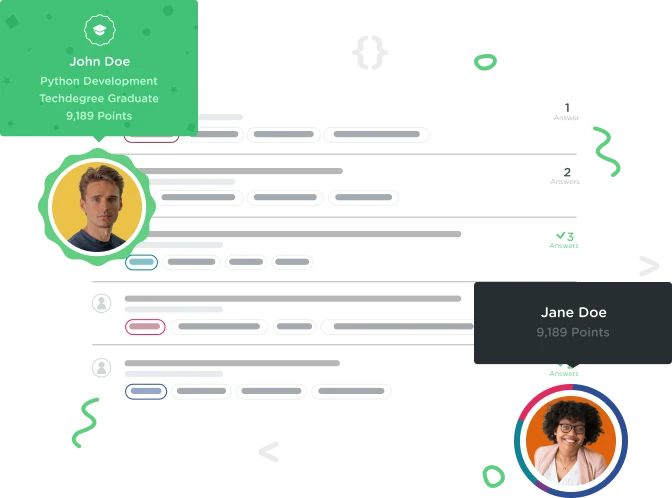

Luke Walz
5,271 PointsHaving trouble understanding what throw, catch, and try mean.
What does it actually mean to 'throw an exception'? Why is this necessary?
Why would I want my program to 'catch an exception'?
Does try just test something and, if it does not find an exception, run the code within it?
Thanks
4 Answers

andren
28,558 PointsI think the easiest way to illustrate the point and use of exceptions is by providing a practical example of code where exception handling is used.
Let's say you were creating a program that asked the user to input two numbers and then told them what they added up to, doing so would be pretty simple:
int number1 = 0;
int number2 = 0;
System.Console.WriteLine("Enter a number: ");
number1 = int.Parse(System.Console.ReadLine());
System.Console.WriteLine("Enter a second number: ");
number2 = int.Parse(System.Console.ReadLine());
Console.WriteLine(number1+number2);
You just ask for two numbers and then print them added together, but since the console returns all input as strings you have to convert them to int first, using int.Parse.
Now the code above will work perfectly fine if a user does what is asked, enter two numbers, however what will happen if a user decided to enter something besides a number, like a word or a letter?
Well what will happen is that when the int.Parse method gets passed the string it will realize that there is no way it can convert the string into a number, but then what will it do? It has a return type of int so in order to finish executing it has to return a number to you, but doing so is impossible, it has encountered an error that it cannot solve by itself. Therefore it raises an exception, an exception is a way to tell the method that called you that for one reason or another you cannot do what you were asked to do, it's called throwing because the exception is "thrown" back to the method that called it.
And once the exception is thrown one of two things can happen, if the method has a catch block prepared that is setup to "catch" the exception then the code within it will be run, if there is no catch block setup to catch it then the exception will cause the program to crash.
The reason this is useful is that it allows you to write code that will only run if a problem occurs, for example for the above code I could do something like this:
int number1 = 0;
int number2 = 0;
Start:
System.Console.WriteLine("Enter a number: ");
try
{
number1 = int.Parse(System.Console.ReadLine());
}
catch (Exception)
{
Console.WriteLine("You need to enter a number!");
goto Start;
}
System.Console.WriteLine("Enter a second number: ");
try
{
number2 = int.Parse(System.Console.ReadLine());
}
catch (Exception)
{
Console.WriteLine("You need to enter a number!");
goto Start;
}
Console.WriteLine(number1+number2);
Note: Normally I wouldn't recommend using goto, loops tend to be more efficient but I use it in this example for the sake of simplicity.
With the way the code is written now, if a user does enter something other than a number, the program will simply prompt the user again, solving the problem.
This is a pretty simple example but hopefully it gets the point across, try and catch allow you to handle errors that the methods you call cannot solve on their own, when you throw an exception you can also specify the exception type, and then also write catch blocks that only catch certain types of exceptions, that way you can have different code that runs depending on the type of error that occurred.
So to sum up and more directly answer your questions, when you wrap code with "try" it does run the code right away, but if an exception occurs it will look for a catch block that is setup to deal with the exception, in contrast if an exception was thrown when using code without a "try" then the program would crash right away due to the unhandled exception.
This post ended up being way longer (and more complex) than I intended it to, but hopefully it helps you understand the concepts I talked about, if you have more questions about it, or think my example was unclear then feel free to ask follow up questions.

Cheikh Faye Ndiaye
7,298 Pointsthank you. it Clear for me now.

Yu-Yang Hsiao
6,295 PointsThanks for the detailed explanation. It's really helpful !!

Isaac Warbrick
1,109 PointsVery helpful response thankyou

Andrew Pike
9,861 PointsThis was extremely helpful for me.
Luke Walz
5,271 PointsLuke Walz
5,271 PointsThanks for the thorough and informative response. It clears things up for me.
Purvi Agrawal
7,960 PointsPurvi Agrawal
7,960 PointsThank You for the Detailed explanation. It cleared some dust !!!
Charlie Harcourt
8,046 PointsCharlie Harcourt
8,046 PointsWow brilliant answer! Really understand it now, thank you :D
Nathan Thompson
7,280 PointsNathan Thompson
7,280 PointsThis was a perfect explanation. Thank you for taking the time to explain in great detail.