Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial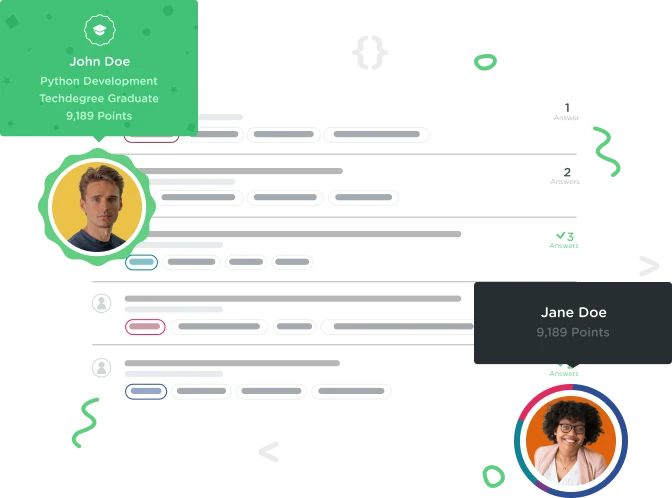
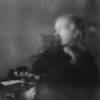
R W
19,894 PointsHaving trouble utilizing 'Enumerate' in Python Collections challenge 'Combo'
I am trying to complete the 'Combo' challenge but when I run my code, it prints out everything three times and, despite working on it all day, I cannot for the life of me figure out how to make it not do that. I suspect that it prints each thing three times due to the use of two for-loops but this is my best attempt so far; nothing else has gotten me this close.
I would prefer to avoid using methods like 'zip' for now and stick with what has been taught in the course.
Any help would be greatly appreciated!!
# combo(['swallow', 'snake', 'parrot'], 'abc')
# Output:
# [('swallow', 'a'), ('snake', 'b'), ('parrot', 'c')]
# If you use list.append(), you'll want to pass it a tuple of new values.
# Using enumerate() here can save you a variable or two.
def combo(it1, it2):
enum_list = list()
for num, item in enumerate(it1):
for j1, j2 in enumerate(it2):
enum_list.append((item, j2))
return enum_list
3 Answers

Seth Kroger
56,413 PointsBecause the for loops are nested inside each other it will take the first item of the first list and combine it with every item of the second, then take the second item and combine it with every item of the second list, and so on. So you only want to have one for loop and find the corresponding item in the second list to combine the two. My quick and dirty solution:
def combo(iter1, iter2):
result = []
iter2_as_list = list(enumerate(iter2))
for i, item1 in enumerate(iter1):
j, item2 = iter2_as_list[i]
result.append((item1, item2))
return result

Rodrigue Loredon
1,338 PointsThanks R W, Dan and Seth. Thanks to your insights I was able to achieve the challenge with my code written as follows:
iter1 = ['swallow', 'snake', 'parrot']
iter2 = 'abc'
def combo(iter1,iter2):
final_list = []
iter2_as_list = list(iter2)
for i, item1 in enumerate(iter1):
item2 = iter2_as_list[i]
final_list.append((item1,item2))
return final_list

Dan Johnson
40,532 PointsSince we're associating elements from each iterable by their index, you can get away with just using enumerate once.
def combo(iter1, iter2):
zipped = []
# The challenge states that the iterables will be
# of the same length so we don't have to worry about
# validation.
# for the index and value in the enumerated iterable
# Append a new tuple with the first value being the pulled in
# from the for loop, and the other by using the index
# to access the value in the second iterable
return zipped
When you call enumerate, each tuple is in the form (index, value_at_index) where index starts at 0 by default.
You could implement a similar solution using range.