Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial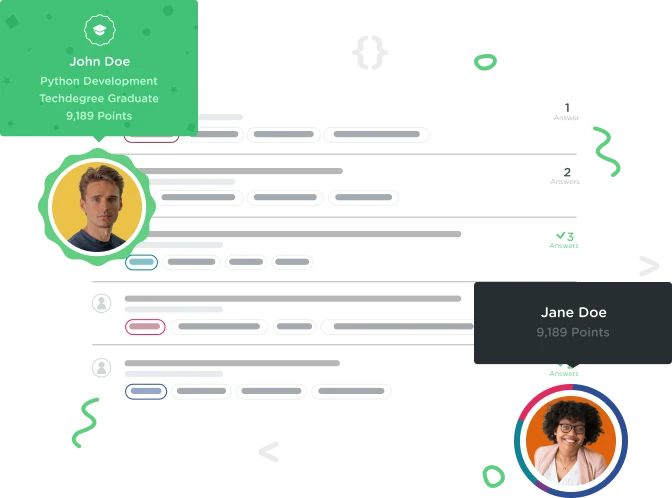
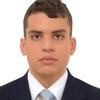
Juan Jaramillo
5,482 PointsHaving trouble validating a String
I'm asked to validate that the String the program is passed either has letters or only the dollar symbol. I'm doing this by converting the String to a char array and validating each element. Still, when the program is passed a number it still lets it go through instead of throwing the exception I'm using. The problem is happening on the metod normalizeDiscountCode that starts on line 11.
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
private String normalizeDiscountCode (String discountCode){
boolean isValid = false;
for ( char letter : discountCode.toCharArray() ) {
if ( Character.isLetter(letter) || letter == '$' ) {
isValid = true;
}
}
if ( isValid ) {
return discountCode.toUpperCase();
} else{
throw new IllegalArgumentException("Invalid discount code");
}
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
try{
this.discountCode = normalizeDiscountCode(discountCode);
} catch ( IllegalArgumentException iae ) {
System.out.println(iae.getMessage());
}
}
}
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}
2 Answers
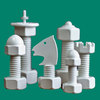
Steven Parker
231,275 PointsOne logic issue left: A "bad" character will not be a letter AND it will not be a dollar sign (not "or").
if ( !(Character.isLetter(letter)) && !(letter == '$') ) {
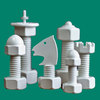
Steven Parker
231,275 PointsThe current logic sets "isValid = true
" when any character is a letter or "$", no matter what the others are.
It might work better to begin by setting "isValid = true
", and then in the loop set it to false if any character is not a letter or "$". For efficiency, you could also throw the exception immediately since you don't need to examine any other characters.
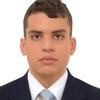
Juan Jaramillo
5,482 PointsI did as you suggested and changed my method to this:
private String normalizeDiscountCode(String discountCode){ boolean isValid = true; for ( char letter : discountCode.toCharArray() ) { if ( !(Character.isLetter(letter)) || !(letter == '$') ) { throw new IllegalArgumentException("Invalid discount code."); } } return discountCode.toUpperCase(); }
Now, I'm getting an error when the exception pops up: Bummer: java.lang.IllegalArgumentException: Invalid discount code. (Look around Order.java line 19)
I don't know why since I think I'm doing exactly what they asked me for : In the normalizeDiscountCode verify that only letters or the $ character are used. If any other character is used, throw a IllegalArgumentException with the message Invalid discount code.
I'm sorry if this is something obvious but I really can't seem to find the problem by myself here