Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial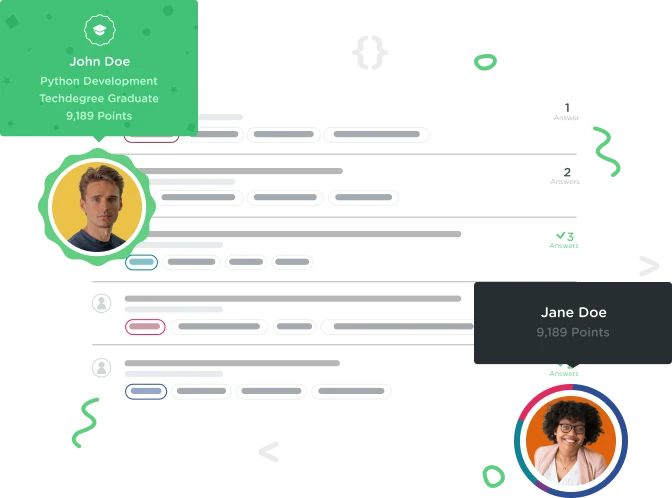

Jason Ladieu
10,635 PointsHaving trouble with getting a function to call. The same function works in a similar program. What am I missing here?
I can't get the try_again() function to call correctly. I keep getting the following error.
Traceback (most recent call last): File "/Users/jasonladieu/Desktop/Untitled.py", line 54, in <module> start_game() File "/Users/jasonladieu/Desktop/Untitled.py", line 50, in start_game com_random_number_game(1, 10) File "/Users/jasonladieu/Desktop/Untitled.py", line 22, in com_random_number_game try_again() File "/Users/jasonladieu/Desktop/Untitled.py", line 4, in try_again again = input("Would you like to play again?\n") File "<string>", line 1, in <module> NameError: name 'yes' is not defined Jasons-iMac:Desktop jasonladieu$
Here is my code:
import random
def try_again():
again = input("Would you like to play again?\n")
again = str(again.upper())
if again == "YES":
return com_random_number_game()
else:
quit()
def com_random_number_game(a, b):
guess = random.randint(a, b)
print("Welcome to the number guessing game.")
user_num = input("Enter a number between " + str(a) + " and " + str(b) + " for the computer to guess.\n")
user_num = int(user_num)
if guess == user_num:
print("The computer guessed your number! The computer guessed " + str(guess) + ".")
try_again()
if guess < user_num:
low_guess = guess + 1
print("The computer guessed lower than your number. The computer guessed " + str(guess) + ". Time to guess again!\n")
guess_two = random.randint(low_guess, b)
if guess_two == user_num:
print("The computer guessed your number! The computer guessed " + str(guess_two) + ".")
try_again()
else:
print("The computer did not guess your number. The computer guessed " + str(guess_two) + ".")
try_again()
if guess > user_num:
high_guess = guess - 1
print("The computer guessed higher than your number. The computer guessed " + str(guess) + ". Time to guess again!\n")
guess_two = random.randint(a, high_guess)
if guess_two == user_num:
print("The computer guessed your number! The computer guessed " + str(guess_two) + ".")
try_again()
else:
print("The computer did not guess your number. The computer guessed " + str(guess_two) + ".")
try_again()
else:
print("Something is wrong!")
quit()
com_random_number_game(1, 10)
1 Answer
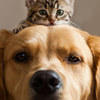
Devin Scheu
66,191 PointsIt seems that your returning com_random_number_game() in your try_again() function without giving it any parameter's even though you ask for them in the function.
Jason Ladieu
10,635 PointsJason Ladieu
10,635 PointsEven if i change that I get the same error.