Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial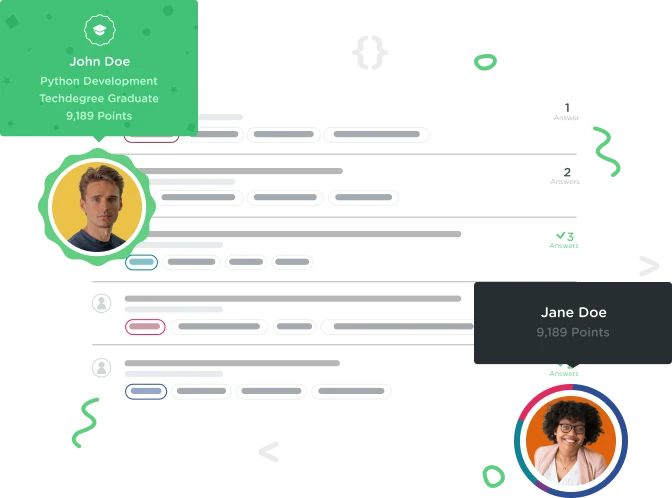
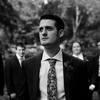
Christopher Gardner
12,719 PointsHaving trouble with multiple &&
// Players haven't tried any questions yet, so set to false
var answer1 = false;
var answer2 = false;
var answer3 = false;
var answer4 = false;
var answer5 = false;
// Question 1
var question1 = prompt('What is the reserved phrase to start a JavaScript variable?');
if (question1.toUpperCase === 'VAR') {
answer1 = true;
}
// Question 2
var question2 = prompt('What coding language has nothing to do with JavaScript, but could be confused to similar?');
if (question2.toUpperCase === 'JAVA') {
answer2 = true;
}
// Question 3
var question3 = prompt('What program converts program language into machine-code or lower form to be executed by a computer?');
if (question3.toUpperCase === 'COMPILER') {
answer3 = true;
}
// Question 4
var question4 = prompt('What HTML tag (minus the \'<>\') would you use to place JavaScript into your HTML file?');
if (question4.toUpperCase === 'SCRIPT') {
answer4 = true;
}
var question5 = prompt('What year is it?');
if (parseInt(question5) === 2019) {
answer5 = true;
}
if ( answer1 === true && answer2 === true && answer3 === true && answer4 === true && answer5 === true ) {
document.write('Congrats! You have got all 5 correct! You won the gold crown!');
}
The document .write on the last if statement won't display with the comparisons shown as they are. However, if I do the below, it runs. Why is this?
if ( answer1, answer2, answer3, answer4, answer5 ) {
document.write('Congrats! You have got all 5 correct! You won the gold crown!');
}
2 Answers

martinjones1
Front End Web Development Techdegree Graduate 44,824 PointsHi Christopher,
My apologies, I totally missed that and though you did not already include that!
OK, so I spent some more time debugging and the problem is simply that "toUpperCase" should be "toUpperCase()" as it is a function call.
The end code should look like that below:
// Players haven't tried any questions yet, so set to false
var answer1 = false;
var answer2 = false;
var answer3 = false;
var answer4 = false;
var answer5 = false;
// Question 1
var question1 = prompt('What is the reserved phrase to start a JavaScript variable?');
if (question1.toUpperCase() === 'VAR') {
answer1 = true;
}
// Question 2
var question2 = prompt('What coding language has nothing to do with JavaScript, but could be confused to similar?');
if (question2.toUpperCase() === 'JAVA') {
answer2 = true;
}
// Question 3
var question3 = prompt(
'What program converts program language into machine-code or lower form to be executed by a computer?');
if (question3.toUpperCase() === 'COMPILER') {
answer3 = true;
}
// Question 4
var question4 = prompt('What HTML tag (minus the \'<>\') would you use to place JavaScript into your HTML file?');
if (question4.toUpperCase() === 'SCRIPT') {
answer4 = true;
}
var question5 = prompt('What year is it?');
if (parseInt(question5) === 2019) {
answer5 = true;
}
if (answer1 === true && answer2 === true && answer3 === true && answer4 === true && answer5 === true) {
document.write('Congrats! You have got all 5 correct! You won the gold crown!');
}
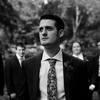
Christopher Gardner
12,719 PointsAh, can't believe I missed that haha! Thank you!

martinjones1
Front End Web Development Techdegree Graduate 44,824 PointsYou are quite close to the solution, so don't want to rob you of the satisfaction of solving it.
Essentially the && operator is doing what is should be.
It is just that the last question is returning false.
Think about why this could be, why is it not true for the Number, 2019. Knowing this will help you decide on the best option for you to fix it :)
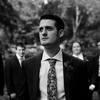
Christopher Gardner
12,719 PointsI've tried several things, and can't quite seem to figure it out. Is it something to do with the parseInt that I've done wrong? I'm just confused as to why this won't work:
if ( answer1 === true && answer2 === true && answer3 === true && answer4 === true && answer5 === true ) { document.write('Congrats! You have got all 5 correct! You won the gold crown!'); }
but this will:
if ( answer1, answer2, answer3, answer4, answer5 ) { document.write('Congrats! You have got all 5 correct! You won the gold crown!'); }
Thanks!
passakorn chantarapakorn
2,472 Pointspassakorn chantarapakorn
2,472 Pointsi think it could be used this condition for test result 1-5 answer