Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial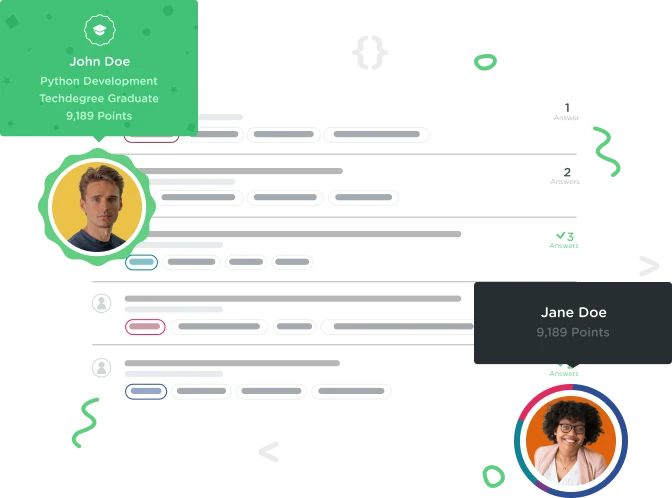
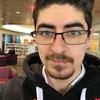
Eddie Licea
1,610 PointsHaving trouble with my code. I'm getting this error: invalid literal for int() with base 10: 'e'.
TICKET_PRICE = 10
tickets_remaining = 100
while tickets_remaining >= 1:
print("There are {} tickets remaining.".format(tickets_remaining))
name = input("What is your name? ")
number_of_tickets = input("How many tickets would you like to buy, {}? ".format(name))
#Expect a ValueError to happen and hande it appropriately... Remember to test it out
try:
number_of_tickets = int(number_of_tickets)
#Raise a avalue error if the request is for more tickets that are available
if number_of_tickets > tickets_remaining:
raise ValueError("There are only {} tickets remaining".format(tickets_remaining))
except ValueError as err:
#include the error text in the output
print("Oh no! We ran into an issue. {}. Please try again...".format(err))
else:
math = number_of_tickets * TICKET_PRICE
print("Your total is ${}".format(math))
proceed = input("Would you like to proceed? Y/N? ")
if proceed.lower() == "y":
# TODO: Gather credit card information and process it.
print("SOLD!")
tickets_remaining -= number_of_tickets
else:
print("Thank you anyway {}".format(name))
print("Sorry the tickets are sold out")
I don't get what is going on. I type in blue when I am prompted for the number of tickets, i'm expecting the value error to show up as I set it up but it doesn't.
3 Answers
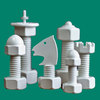
Steven Parker
231,269 PointsI tried this in the workspace and it seems to run as I would expect:
How many tickets would you like to buy, Joe? blue
Oh no! We ran into an issue. invalid literal for int() with base 10: 'blue'. Please try again...
The "Oh no..." part clearly indicates the ValueError exception was caught, and the inserted message indicates the cause. If I type the letter 'e' instead of the word "blue" I'll get the same inserted message as you saw.

Mathew V L
2,910 PointsSteven Parker Is that really expected? The ". invalid literal for int() with base 10: 'blue'. Please try again..." should run the same as if we were inputting 1k tickets correct? When I type in 1000 which is a ValueError, I get "Oh no, we ran into an issue. There are only 100 tickets remaining. please try again!!!! ."
Kind of confused but any help would be appreciated!

Mathew V L
2,910 PointsI looked at another thread and found this:
Hey! I had the same issue so I started to google it and try to change my code. The thing I realized that an error occurs when we want a character to be an integer. But it's not true and we have no exception for it (I dont't know why as it's ValueError).
And after a long time i found the solution:
try:
number_tickets = int(number_tickets)
if number_tickets > tickets_remaining:
raise ValueError
except ValueError as err:
print("Try again. We have only {} tickets.".format(tickets_remaining))
Definitely resolved the issue, but not sure it's what we want
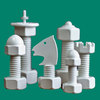
Steven Parker
231,269 PointsTyping 1000 would not generate a value error, but you raise one explicitly:
if number_of_tickets > tickets_remaining:
raise ValueError("There are only {} tickets remaining".format(tickets_remaining))
And the message shown is the one you passed to the "raise" function.
So this is also exactly the result for what is programmed. I suppose the real issue is what do you want it to do?

Mathew V L
2,910 PointsHi, Thanks Steven Parker!! for the help. from the video, the teacher says we want to make all errors understandable. So when we tried to use a string in "How many tickets?" I wasn't expecting invalid literal, I expected it to show "Oh no! We ran into an issue. There are only {} tickets remaining". tickets_remaining. Please try again.
To simply put it, how raise an error to output something else besides "invalid literal for int() with base 10"
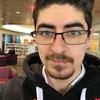
Eddie Licea
1,610 PointsThat's exactly what I wanted to know lol.