Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial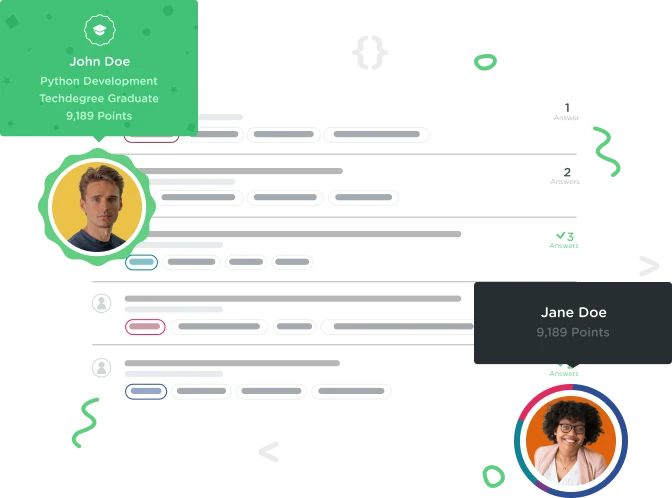
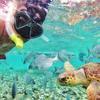
Gaspar Santiago
3,377 PointsHaving trouble with my getTileCount method. Not quite sure where I'm going wrong.
Can anyone tell me where I'm going wrong?
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount(char tile)
{
int count = 0;
for(tile: mHand.toCharArray())
{
if(mHand.indexOf(tile) >= 0 )
{
count = tile;
}
count++;
}
return count;
}
}
3 Answers
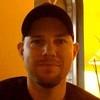
Jeremy Hill
29,567 PointsYour code should look like this:
public int getTileCount(char tile)
{
int count = 0;
for(char letter : mHand.toCharArray()){
if(letter == tile )
count++;
}
return count;
}
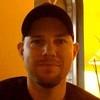
Jeremy Hill
29,567 PointsIt does require a char to be passed in because it is supposed to count the number of tiles that are in the mHand that match the one being passed in.

Eric Slater
6,990 PointsI'm a little confused by this. I thought 'if' statements had to be followed by {} which is where the count++ would go. but when I do that I get a compile error.

Craig Fender
7,605 PointsJeremy Hill,
Okay, that makes sense. I misunderstood the method. In that case, your solution is perfect.
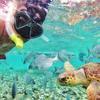
Gaspar Santiago
3,377 PointsThanks guys! I should also probably not do this for hours on end... that might help. haha!
Craig Fender
7,605 PointsCraig Fender
7,605 PointsIt looks like you're trying to do two different things with your getTileCount() method. What you're doing is creating a foreach loop to iterate through each element in the mHand char array, but then you're treating mHand as an array, looking for the index of the value tile passed to the method. This is trying to do two different things. It also seems to do something different than the meaning of getTileCount, which should count how many separate elements are in the player's hand.
If I understand what this class, and specifically this method is doing (I haven't went through this course), it seems to be creating a Scrabble Player who has a hand full of Scrabble tiles. If you want to get the tile count of how many pieces the player has, then first you don't want to pass any tile to the method, and then you want the length of the array or string. You can accomplish this in two ways:
public int getTileCount() { return mHand.toCharArray().length; }
Or...
public int getTileCount() { return mHand.length(); }
Both ways should do the trick. It's just whichever you prefer. I hope that helps.