Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial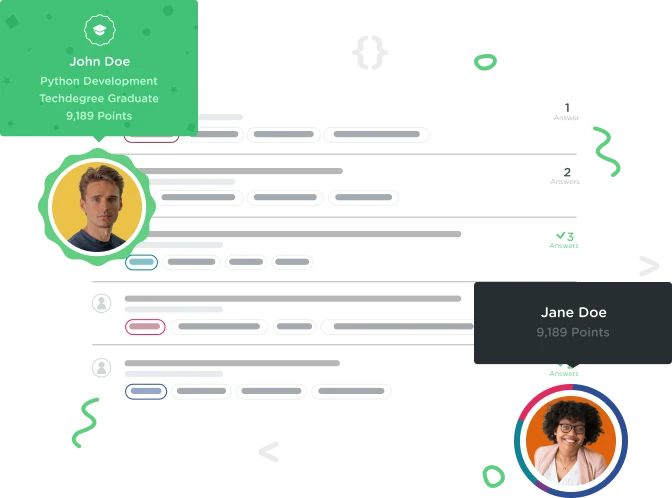
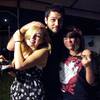
Robert Alonso
4,164 PointsHaving trouble with my sticky nav bar
I'm trying to write a piece of code that will create a sticky nav bar that contains 2 sets of links. One set is going to be on the right, and one set will be on the left. So far I've been able to get the nav bar to stick, but one set of links won't show up. What am I doing wrong that's making the second set of links disappear? Here's my code for reference
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>My Page</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<div class="wrapper">
<div class="header">
<ul class="navl">
<li><a href="#">Gallery</a></li>
<li><a href="#">About</a></li>
<li><a href="#">Home</a></li>
</ul>
<ul class="navr">
<li><a href="#">Contact</a></li>
<li><a href="#">Team</a></li>
<li><a href="#">Showcase</a></li>
</ul>
<div class="logo">h</div>
</div>
</div>
</body>
</html>
.wrapper {
width: 100%;
}
.header ul {
margin-left: -10px;
margin-top: -15px;
padding: 30px;
list-style-type: none;
background: #333;
width: 97%;
position: fixed;
z-index: 10;
}
.logo {
width: 100px;
height: 100px;
color: #fff;
}
ul.navl li a {
display: inline;
padding: 0 10px 0 0;
font-family: Helvetica;
float: left;
color: #FFF;
text-shadow: 2px 2px #000;
font-size: 20px;
text-decoration: none;
z-index: 20;
}
ul.navr li a {
display: inline;
padding: 0 10px 0 0;
font-family: Helvetica;
float: right;
color: #FFF;
text-shadow: 2px 2px #000;
font-size: 20px;
text-decoration: none;
}
4 Answers
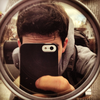
Matt Morris
6,982 PointsI messed around with it, not sure if this is the best approach but it's a good starting point:
.wrapper {
width: 100%;
}
.header ul {
margin-left: -10px;
margin-top: -15px;
padding: 30px;
list-style-type: none;
background: #333;
width: 45%;
position: fixed;
z-index: 10;
}
.headerright ul {
padding: 30px;
list-style-type: none;
background: #576;
width: 45%;
position: fixed;
top: -24px;
left: 50%;
}
.logo {
width: 100px;
height: 100px;
color: #fff;
}
ul.navl li a {
display: inline;
padding: 0 10px 0 0;
font-family: Helvetica;
float: left;
color: #FFF;
text-shadow: 2px 2px #000;
font-size: 20px;
text-decoration: none;
z-index: 20;
}
ul.navr li a {
display: inline;
padding: 0 10px 0 0;
font-family: Helvetica;
float: right;
color: #FFF;
text-shadow: 2px 2px #000;
font-size: 20px;
text-decoration: none;
}
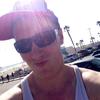
Carsten Pleiser
8,386 PointsHi Robert,
Just some thoughts on your code:
Try to work with the layout first, e.g. leave all the text styles and decoration for now. Also, as James said, make sure you're not repeating yourself (DRY Principle).
Some things I've noticed and maybe a few tips below:
- Do you really want to target the "a" elements, e.g. "ul.navr li a" or would targeting the .navl, .navr classes itself work better?
- .navl & .navr are both children of the header class. When floating them right, make sure you give the header a height, otherwise it will collapse.
- At the moment all .ul (unordered lists) of the header element have the same properties? Is this really what you want? Have a look there and maybe experiment in Codepen or Treehouse Workspace.
Not sure if this helps, I hope it does. Let me know if you're still having trouble. Keep on going, you are very close.
Cheers, Carsten
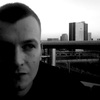
James Ingmire
11,901 PointsBreak down your code so your not repeating yourself, using z-index the same as each other, then target the left and right setting float right and left separately. (''' .navr .navl { display: inline; padding: 0 10px 0 0; font-family: Helvetica color: #FFF; text-shadow: 2px 2px #000; font-size: 20px; text-decoration: none; z-index: 20 } .navr { float: right; } .navl { float: left; } ''') Sorry if i am wrong and wasted your time, may help. Let me know if works. :(
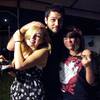
Robert Alonso
4,164 PointsReduced my code to this. It's neater now, but the left part of the nav bar still wont show up
ul.navr li a, ul.navl li a {
display: inline;
padding: 0 10px 0 0;
font-family: Helvetica;
color: #FFF;
text-shadow: 2px 2px #000;
font-size: 20px;
text-decoration: none;
z-index: 40;
}
ul.navr li a {
float: right;
}
ul.navl li a {
float: left;
}
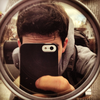
Matt Morris
6,982 Pointsand index.html
<html>
<head>
<meta charset="UTF-8">
<title>My Page</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<div class="wrapper">
<div class="header">
<ul class="navl">
<li><a href="#">Gallery</a></li>
<li><a href="#">About</a></li>
<li><a href="#">Home</a></li>
</ul>
</div>
<div class="headerright">
<ul class="navr">
<li><a href="#">Contact</a></li>
<li><a href="#">Team</a></li>
<li><a href="#">Showcase</a></li>
</ul>
<div class="logo">h</div>
</div>
</div>
</body>
</html>
Robert Alonso
4,164 PointsRobert Alonso
4,164 PointsIt's not quite what I had in mind, but it works. I actually like it better. Thanks! :]
Robert Alonso
4,164 PointsRobert Alonso
4,164 PointsIt's not quite what I had in mind, but it works. I actually like it better. Thanks! :]