Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial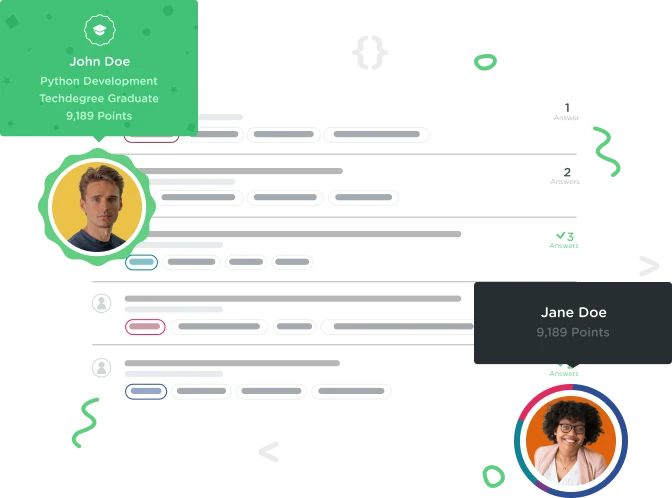
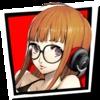
Amanda Stewart
1,371 PointsHaving trouble with overriding the Equals method practice problem
I've been bashing my head against this for a week, and tried numerous things. The problem asks us to check and see if two VocabularyWords are the same, but I don't know where the second VocabularyWord should be coming from. I've tried changing it so that the obj that is entered is an array, and running it through a foreach loop, I've tried comparing it to Word, and a dozen other thngs, but nothing seems to work. I've decided to step back and ask for help because it's becoming more than a bit frustrating and demoralizing.
Right now the only error this throws is that it returns true even when the words are not equal.
using System;
namespace Treehouse.CodeChallenges
{
public class VocabularyWord
{
public string Word { get; private set; }
public VocabularyWord(string word)
{
Word = word;
}
public override string ToString()
{
return Word;
}
public override bool Equals(object obj)
{
if(!(obj is VocabularyWord))
{ return false; }
VocabularyWord that = obj as VocabularyWord;
return this == that;
}
}
}
1 Answer

andren
28,558 PointsYour code is incredibly close to correct. The issue is that you seem to have slightly misunderstood what the challenge is asking you to do. The challenge description is as follows
Add an Equals method to the VocabularyWord class that returns true if the words of two VocabularyWord objects are the same.
Notice that it asks you to return true
if the words of two VocabularyWord
objects are equal, rather than asking you to compare the VocabularyWord
objects themselves. The VocabularyWord
object has a property called Word
which is the thing the challenge actually wants you to compare.
If you modify your method so that it compares the Word
properties of the two VocabularyWord
objects like this:
public override bool Equals(object obj)
{
if(!(obj is VocabularyWord))
{
return false;
}
VocabularyWord that = obj as VocabularyWord;
return this.Word == that.Word;
}
Then your code will pass.
Amanda Stewart
1,371 PointsAmanda Stewart
1,371 PointsThank you so much. That was exactly what I needed, I was going insane trying to figure out what I was doing wrong!