Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial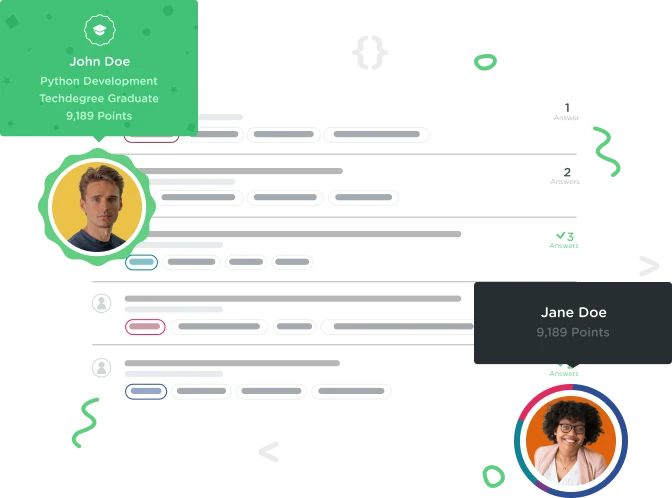
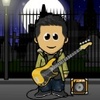
Cisco Rendon
Courses Plus Student 37,825 PointsHaving Trouble with Repository Challenge 2!! UGH
I have done everything we were taught in the videos and I keep getting the Bummer!. I have done everything short of calculus to figure out the answer. Maybe if I had 2 PhD's in engineering it would be a little simpler but it just ain't lol Anyone have an idea of where either I or the challenge went wrong?
Much appreciated
using Treehouse.Models;
namespace Treehouse.Data
{
public class VideoGamesRepository
{
// TODO Add GetVideoGames method
// TODO Add GetVideoGame method
private static VideoGame[] _videoGames = new VideoGame[]
{
new VideoGame()
{
Id = 1,
Title = "Super Mario 64",
Description = "Super Mario 64 is a 1996 platform video game developed and published by Nintendo for the Nintendo 64.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = true
},
new VideoGame()
{
Id = 2,
Title = "Mario Kart 64",
Description = "Mario Kart 64 is a 1996 go-kart racing game developed and published by Nintendo for the Nintendo 64 video game console.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = false
}
};
public VideoGame[] GetVideoGame(int id)
{
VideoGame videoGameToReturn = null;
foreach (var videoGame in _videoGames)
{
if (videoGame.Id == id)
{
videoGameToReturn = videoGame;
break;
}
}
return videoGame;
}
}
}
namespace Treehouse.Models
{
// Don't make any changes to this class!
public class VideoGame
{
public int Id { get; set; }
public string Title { get; set; }
public string Description { get; set; }
public string[] Characters { get; set; }
public string Publisher { get; set; }
public bool Favorite { get; set; }
public string DisplayText
{
get
{
return Title + " (" + Publisher + ")";
}
}
}
}
4 Answers
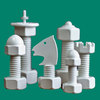
Steven Parker
231,275 PointsIt says "Congrats! You completed the challenge!" when I paste your code in (and replace the task 1 function).
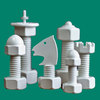
Steven Parker
231,275 PointsIt looks like you're working on task 2 but I don't see the method that should have been added for task 1., did you accidentally remove it?
In multi-task challenges, the work from each task should remain unless the next task specifically instructs otherwise.
Then, in the task 2 function I see two issues:
- the return type should be "VideoGame" (just one, not an array)
- the loop establishes "videoGameToReturn" but the return statement tries to use "videoGame"
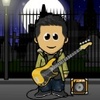
Cisco Rendon
Courses Plus Student 37,825 PointsSteven, Thanks for replying. In the instructions for task 2, it changed some of the coding from task 1. I had it originally and it gave me an error code in the preview screen. So i started tweaking it as per the codes and it just got more tedious from there.
Here are the instructions as noted:
Add a GetVideoGame method to the VideoGamesRepository class. The GetVideoGame method should accept an id parameter of type int. The GetVideoGame method should return the VideoGame object instance from the _videoGames private static field array of VideoGame objects whose Id property matches the provided id parameter value.
I did use videoGameToReturn but it was marked as wrong as well. I had been chopping away at it for over an hour and i just got flustered.
When you say "the return type should be "VideoGame" (just one, not an array)" clear it up for me, maybe I am confusing myself. Thanks for the reply. i do appreciate it.
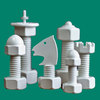
Steven Parker
231,275 PointsOnce you pass task 1, that function should not be modified. It should remain as-is when you add the new function for task 2.
In the new function, "videoGame" is the loop variable and is no longer accessible when the return statement is reached. So it's important to "return videoGameToReturn;
" instead. It was probably "wrong" only because it did not match the declared return type.
And when I say "the return type should be "VideoGame" (just one, not an array)" I'm referring to the function declaration:
public VideoGame[] GetVideoGame(int id)
The brackets after "VideoGame" indicate that the return type is an array. This was the correct return type for the task 1 function ("GetVideoGames" plural). But this time the return type will be a single object, so the brackets should not be there.
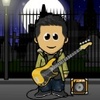
Cisco Rendon
Courses Plus Student 37,825 PointsSteven, this is what I got and it's still wrong. I am not sure if it's because I am tired or it's after 1am but it's still wrong. I appreciate the assist but i think this is one challenge i will not pass anytime soon. I tried using the answer of return videoGameToReturn and the way it's marked but still wrong.
public VideoGame GetVideoGame(int id)
{
VideoGame videoGameToReturn = null;
foreach (var videoGame in _videoGames)
{
if (videoGame.Id == id)
{
videoGameToReturn = videoGame;
break;
}
}
return videoGame;
}
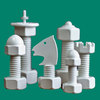
Steven Parker
231,275 Pointsit's important to "return videoGameToReturn;", because "videoGame" is the loop variable and is no longer accessible when the return statement is reached.
After making that change, this last code should pass (assuming you have also put back the task 1 function).
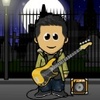
Cisco Rendon
Courses Plus Student 37,825 PointsSteven,
Thanks for the assist but it is not going thru and I am not wasting anymore time on this challenge. I am at the end of the rope for it and at this point I could careless what the answer is. If i miss one challenge, so what. Thanks for the assist but it isn't helping. Truly, much appreciated, I really mean it.
And for giggles, here is my code:
public VideoGame GetVideoGame(int id)
{
VideoGame videoGameToReturn = null;
foreach (var videoGame in _videoGames)
{
if (videoGame.Id == id)
{
videoGameToReturn = videoGame;
break;
}
}
return videoGameToReturn;
}
Cisco Rendon
Courses Plus Student 37,825 PointsCisco Rendon
Courses Plus Student 37,825 Pointshahaha I wish it said that to me, but it's beyond the point. Thanks for the assist. Again, i truly appreciate it. You are a gentleman and a scholar...
Steven Parker
231,275 PointsSteven Parker
231,275 PointsIt should work, as long as you also put the other function (GetVideoGames, plural) back with it.
Anyway, if it still won't pass you might report it to the Support folks.
Happy coding!