Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial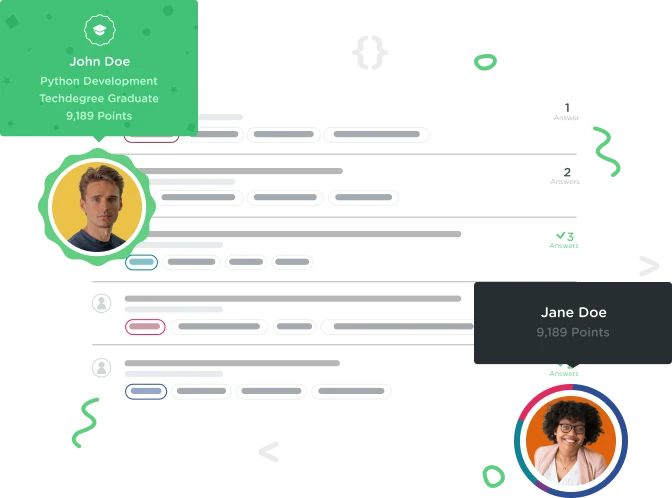

Samith Vijayan
11,318 PointsHaving trouble with the code
Not abl;e to execute the code kindly help resolving this pblm
/*
1. Store correct answers
- When quiz begins, no answers are correct
*/
let correct=0;
// 2. Store the rank of a player
let rank;
// 3. Select the <main> HTML element
const main=document.querySelector('main');
/*
4. Ask at least 5 questions
- Store each answer in a variable
- Keep track of the number of correct answers
*/
const answer1=prompt('enter the programe that have the name of a gem');
if(answer1.toUpperCase() === 'RUBY'){
correct+=1;
}
const answer2=prompt("Name a programe language that's also a snake.");
if(answer2. toUpperCase() === 'PYTHON'){
correct+=1;
}
const answer3=prompt("what language do you use to styleweb pages");
if (answer3. toUpperCase()==='CSS'){
correct+=1;
}
const answer4=prompt("What language do you use to build the structure of webpage");
if(answer4. toUpperCase()='HTML'){
correct+=1;
}
const answer5=prompt("what language do you prefer to interact with user");
if(answe5.toUpperCase()="JAVASCRIPT");
{
correct+=1;
}
/*
5. Rank player based on number of correct answers
- 5 correct = Gold
- 3-4 correct = Silver
- 1-2 correct = Bronze
- 0 correct = No crown
*/
if( correct===5)
{
rank="Gold";
}
if(correct>=3)
{
rank="silver";
}
if(correct>=2){correct
rank="bronze";
}
if(correct===0)
{
rank="no crown";
}
// 6. Output results to the <main> element
main.innerHTML=
`<h2>You got ${correct} out of 5 questions correct
</h2>
<p>Crown earned<strong>${rank}</strong><p>`;
1 Answer
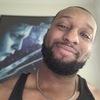
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsHi Samith,
Your code looks pretty good for the most part. Great job getting this far.
There only a few small things I notice that would lead to your code not working exactly the way you would expect it to.
First, after question 5, your if condition reads if(answe5.toUpperCase()...
. You'll want to make sure you squeeze an "r" in between the "e" and "5", as answe5 is an undefined variable.
Second, in your section of if statements, one of your statements is if(correct >= 2) ...
, this should be: if(correct >= 1)...
otherwise, you have no scenario where one single right answer is covered. Which means that if I only answer one of the questions correctly, rank will remain undefined and the string printed between the main tags will be You got 1 question out of 5 correct | Crown earned undefined
Finally, there are at least two ways you can do the if statements to get the correct results. First I'll explain what's going on now using an example. So... let's say I answer all five questions correctly. The first if condition tests if correct is strictly equal to five. And it is, so that will evaluate to true. rank now holds the value of Gold. The next condition tests if correct is greater than or equal to three. Again this would evaluate to true (as five is greater than three), so rank becomes Silver. Next the app tests if correct is greater than or equal to two (or one once you've made the change). Again this evaluates to true, so now rank holds the value Bronze. The last condition fails, so rank remains Bronze.
So the first way you can fix this little bug is to reverse the order of the conditions. You could start by testing if correct is equal to zero, then greater than or equal to one, then greater than or equal to three, then five. In that case, if I get all five questions correct, rank will still change multiple times (from Bronze, to Silver, to Gold), but in the end it will be the correct value (based on your intentions).
The other way (and the one that potentially causes your app to be more efficient) would be to chain your conditions with if...else statements. It would look something like...
if (correct === 5) {//...
} else if (correct >= 3) {//...
} else if (//...) {/*...*/}
In this situation, whenever one of the conditions evaluates to true, that corresponding code block runs and then the app exits that sequence of if conditions.
Hope that makes sense. If you need any more help, just let me know.