Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial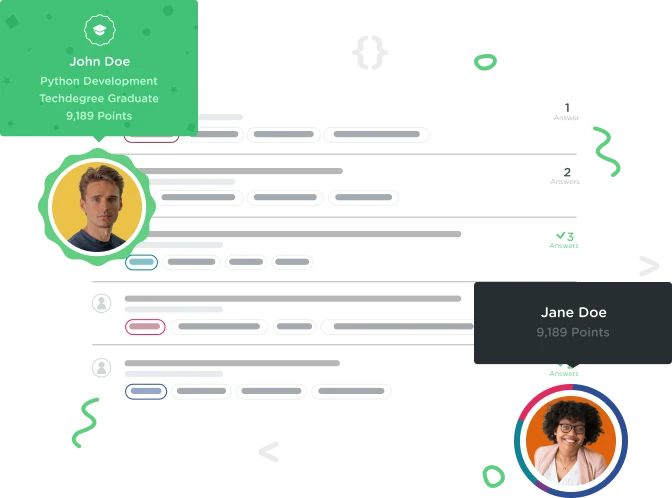

Pedro Laplaza
1,960 PointsHaving trouble with the task Not being able to solve it
I am not being able to solve the problem, something seems to be missing.
Here is what i am asked to do: In our Treehouse model struct below we have a property named friends. It is a dictionary that holds information about our friends including their profile pictures. In the ViewController class, using the key profilePicture, retrieve the image name as a string from the dictionary and assign it to a constant named profileImageName. I’ve already created an instance of the Treehouse struct and stored a reference to the friends library in a constant named friends for you to use.
Once you have the image name, create an instance of UIImage using the image name and store it in a constant called profilePicture.
And here the code give to me:
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
let friends = Treehouse().friends
}
}
// Treehouse.swift
struct Treehouse {
let friends = [
"firstName": "Susan",
"lastName": "Olson",
"profilePicture": "susan_profile.png"
]
}
So i created a new struct to handle the data and return them as strings and this is how my files look now.
// Treehouse.swift
struct Treehouse {
let friends = [
"firstName": "Susan",
"lastName": "Olson",
"profilePicture": "susan_profile.png"
]
}
struct Information {
var firstName: String?
var lastName: String?
var profilePicture:String?
init(){
let friendsList = Treehouse().friends
let treehouseFriends = friendsList
firstName = treehouseFriends["firstName"] as String!
lastName = treehouseFriends["lastName"] as String!
profilePicture = treehouseFriends["profilePicture"] as String!
}
}
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
let friends = Treehouse().friends
let information = Information()
let profileImageName = information.profilePicture
}
}
And i get an error saying " Bummer! Make sure you assign the image to a constant named profilePicture and check your syntax." If i change my constant name to profilePicture i get the same error but with profileImageName
I am pretty sure this is not the best way to solve it, but it works on the playground
Can someone help me here please?
3 Answers
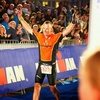
Steve Hunter
57,712 PointsHi Pedro,
You need to add two lines to the challenge.
First, you access the friends
dictionary with the key profilePicture
to bring back the image file name and store that in the constant, profileImageName
(remember to unwrap this as you're accessing a dictionary). You then use the UIImage
method named:
to generate the image from the filename and store that in the profilePicture
constant:
let friends = Treehouse().friends
let profileImageName = friends["profilePicture"] as String!
let profilePicture = UIImage(named: profileImageName)
I hope that makes sense.
Steve.

Pedro Laplaza
1,960 Pointswow, i really went the other way around, i thought that should be part of the struct and not the viewController Thanks man If you can help me a little more, do you know why when i run this on a playground i get "nil" on the "let profilePicture"?
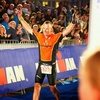
Steve Hunter
57,712 PointsIs that your code or the lines I posted? I'm just starting a playground ...
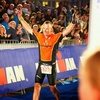
Steve Hunter
57,712 PointsI get an error in the playground about not using the profilePicture
constant - that's understandable. As soon as I use profilePicture
, that goes away.
Given that the file, susan_profile.png
, doesn't exist, we're bound to come up with an error somewhere.
What errors are you getting?

Pedro Laplaza
1,960 PointsNo errors, just "nil" on the last line, but as you said, this might be because i am calling a UIImage element that i dont actually have
This is my playground
import UIKit
struct Treehouse {
let friends = [
"firstName": "Susan",
"lastName": "Olson",
"profilePicture": "susan_profile.png"
]
}
let friends = Treehouse().friends
let profileImageName = friends["profilePicture"] as String!
let profilePicture = UIImage(named: profileImageName)
Thanks for your help again man
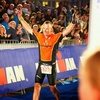
Steve Hunter
57,712 PointsYes, that's because you're asking for a file that doesn't exist. Kindly, the compiler throws a nil
rather than crashing dramatically! ;-)
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsYour final code looks like:
There's no changes to the other file, it is all in
viewDidLoad()
.