Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial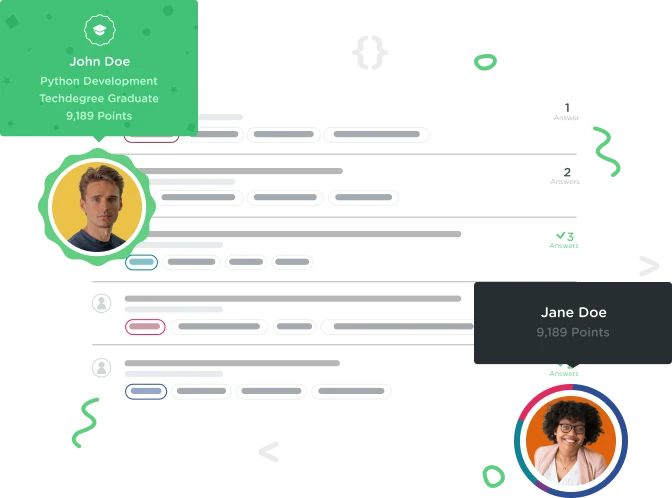
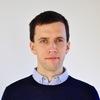
Mazvydas Bartasius
2,359 PointsHaving troubles with property observers. Need help to solve the challange
Can't seem to wrap my head around the concept.
In the editor, I've created a UIViewController subclass, TemperatureController that contains a single property - temperature. Your task is to add a didSet property observer to temperature and set the view's background color depending on value of the temperature. For example, if the temperature is greater than 80, set the view's background color to UIColor.redColor(). Similarly if the temperature is less than 40, set it to blue color, otherwise set it to green color. Note: For changing the view's background color, there's example code in viewDidLoad()
class TemperatureController: UIViewController {
var temperature: Double
init(temperature: Double) {
self.temperature = temperature
super.init()
}
override func viewDidLoad() {
view.backgroundColor = UIColor.whiteColor()
}
}
1 Answer

Tobias Helmrich
31,603 PointsHey there,
to add a property observer to a stored property you have to write the observer inside of curly braces after the property you want to observe, in this case it's temperature
. After that you have to write the code that should be executed each time when the property changed (because we're using didSet
here) inside of curly braces after the observer.
In this case the code inside of the property observer's body should check the temperature and change the background color of the view depending on its value. You can check the different cases with an if, else if and else clause and then give the background a color by using the code you can already see in the viewDidLoad
method.
Like so:
class TemperatureController: UIViewController {
var temperature: Double {
didSet {
if temperature > 80 {
view.backgroundColor = UIColor.redColor()
} else if temperature < 40 {
view.backgroundColor = UIColor.blueColor()
} else {
view.backgroundColor = UIColor.greenColor()
}
}
}
init(temperature: Double) {
self.temperature = temperature
super.init()
}
override func viewDidLoad() {
view.backgroundColor = UIColor.whiteColor()
}
}
I hope that helps, if you have further questions feel free to ask! :)
Mazvydas Bartasius
2,359 PointsMazvydas Bartasius
2,359 PointsThanks, my solution was identical, just had a typo. At least I was on the right track.