Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial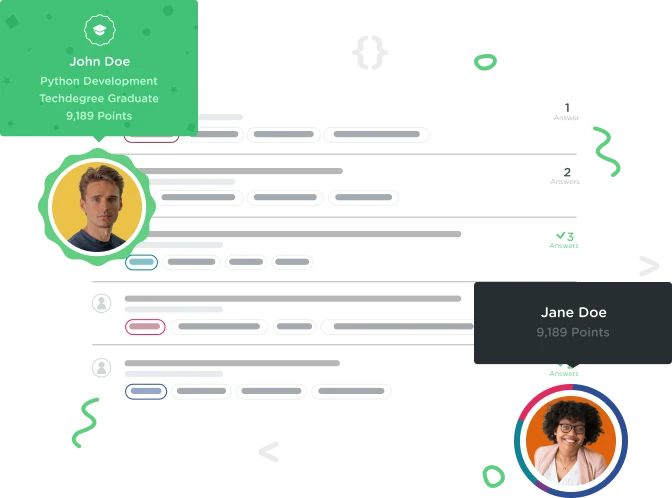
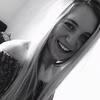
Nadja Summerscales-Gunn
3,441 PointsHeader(...): A valid React element (or null) must be returned. You may have returned undefined.
function Header(props) { return ( <div className="header"> <h1>{props.title}</h1> </div> ); }
Header.propTypes = { title: React.PropTypes.string.isRequired, }
function Application(props) { return ( <div className="scoreboard"> <Header title={props.title}/>
<div className="players">
<div className="player">
<div className="player-name">
Jim Hoskins
</div>
<div className="player-score">
<div className="counter">
<button className="counter-action decrement"> - </button>
<div className="counter-score"> 31 </div>
<button className="counter-action increment"> + </button>
</div>
</div>
</div>
<div className="player">
<div className="player-name">
Andrew Chalkey
</div>
<div className="player-score">
<div className="counter">
<button className="counter-action decrement"> - </button>
<div className="counter-score"> 33 </div>
<button className="counter-action increment"> + </button>
</div>
</div>
</div>
</div>
</div>
); }
Application.propTypes = { title: React.PropTypes.string, };
Application.defaultProps = { title: "Scoreboard", }
ReactDOM.render(<Application />, document.getElementById('container'));
Why is my code throwing me the error: Header(...): A valid React element (or null) must be returned. You may have returned undefined, an array or some other invalid object.
I can't seem to see what the issue is...
1 Answer
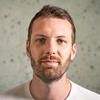
Thomas Dimnet
Python Development Techdegree Graduate 43,629 PointsHi there, I think I know where your code is wrong: you forgot the return statement :).
Try to wrap your big block of code within a return statement and it should work fine.
Keep me in touch :).
Paolo Scamardella
24,828 PointsPaolo Scamardella
24,828 PointsAs far as I can tell, your code seems right. I copied your code and pasted it online, and it is working. The error is saying the Header component is not returning a valid react element.
If you can reformat or indent your code properly, I can take another look at it.