Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial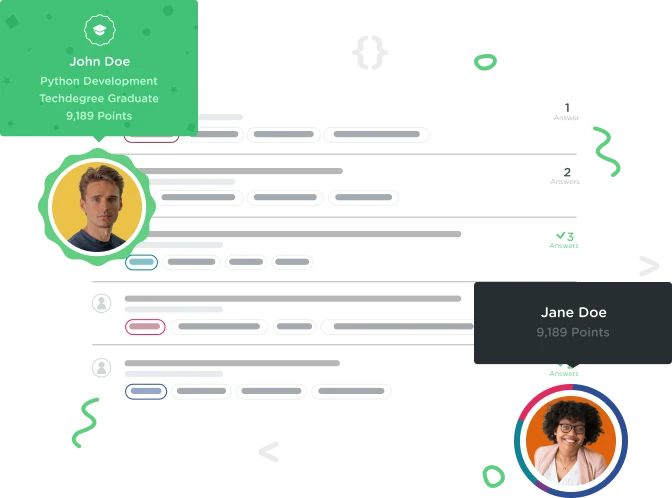

Alexander Pavlenko
654 PointsHello! Could someone please help because i don't really understand the task at hand. And what type is "counter"?
I am also confused about "multiply string by integer". How is this relevant here?
def printer():
while counter:
counter -= 1
print("Hi ")
printer()
7 Answers

dojewqveaq
11,393 PointsHey Alexander!
In python you can multiply a string by an int, and it will print that string how many times you asked it to. For example:
print("_" * 40) # This will print out "_" 40 times
So, the task asks you to pass an argument called "count" to the function, so your have to put that argument between the parenthesis in the function, like this:
def printer(count):
And you don't need to call the function in this exercise, so there is no need to use:
printer()
Now using all that information, your code should look something like this:
def printer(count):
print("Hi" * count)

Alexander Pavlenko
654 PointsThank you! But out of curiosity... Can i complete the task using Loop?

dojewqveaq
11,393 PointsYes! You definetly can. It makes your code a little bigger, but you can do it. The thing is that, you'd have to create a variable to compare the count to. Here is how I did it.
def printer(count):
num = 0
while num < count:
print("Hi")
num += 1
Cheers!

Alexander Pavlenko
654 PointsFrom what i can understand if i do "for count:" loop it does smth while count is True (in other words != 0) can i do smth like this?
def printer(count):
for count:
count -= 1
print("Hi ")

Alexander Pavlenko
654 Pointshow do i add code sections the same way you do?:)

dojewqveaq
11,393 PointsPythonβs for statement iterates over the items of any sequence. So you'd use for loops to go through something like a list of things and execute a block of code while doing so. For example:
list = [1, 2, 3, 4]
for num in list:
print num
This code will print every item in the list. In plain english, it means something like... for every num in this list, I want you to print the num. So the "for count" statement is incorrect. It would read in english: for every count... So it's incomplete. "while count" reads: while count is True, do something.

dojewqveaq
11,393 PointsJust bellow the text field in the "Add an Answer" section, there is a link called Markdown Cheatsheet. There it tells you everything you need to know about how to format your posts. To write a section of code, you need to write a block with three ` . And close it once you are done with your code.

Alexander Pavlenko
654 Pointsmy bad, it was about "while" Keneth gave this example in video https://teamtreehouse.com/library/around-and-around 4:35

dojewqveaq
11,393 PointsWhat do you mean?

Alexander Pavlenko
654 PointsKeneth's sample from that video:
start = 10
while start:
print(start)
start -= 1
I thought i could use it in the task

dojewqveaq
11,393 PointsYou can, but it's slightly different from what you wrote. Here is how I did it.
def printer(count):
while count != 0:
print("Hi")
count -= 1

Alexander Pavlenko
654 Pointsjust did it in Workspaces: both "count != 0" and simply "count" work actually. I think it's crystal clear now, Erik.
I really really appreciate your time on me:) Cheers! Have a great day!

dojewqveaq
11,393 PointsGood to know this works too! No problem! Happy coding =)