Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial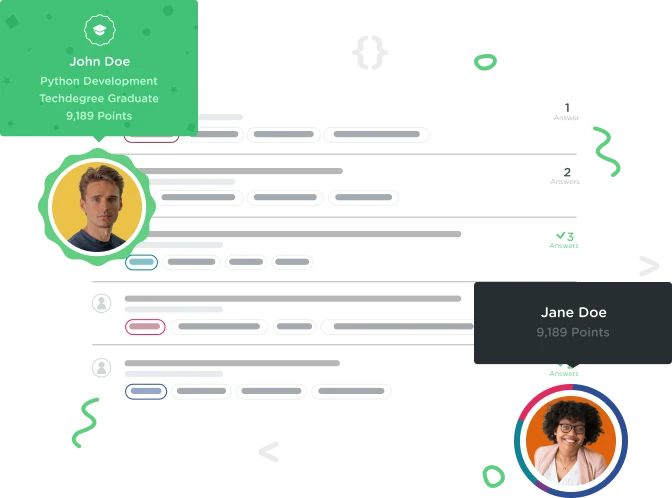

vincent juma
1,159 Pointshello everyone, am quite stuck on the below datetime code. kindly help me complete it.
Create a function named timestamp_oldest that takes any number of POSIX timestamp arguments. Return the oldest one as a datetime object.
Remember, POSIX timestamps are floats and lists have a .sort() method.
# If you need help, look up datetime.datetime.fromtimestamp()
# Also, remember that you *will not* know how many timestamps
# are coming in.
def timestamp_oldest():
1 Answer
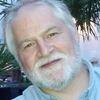
Jeff Muday
Treehouse Moderator 28,720 PointsPOSIX Timestamps are really big numbers which represent date/time. See the example below for two friends, Justin and Hannah. Justin was born December 4, 1992. Hannah was born February 12, 1995. Who is older? Justin, of course! But he will have a smaller number for a timestamp because he was born before her. See below.
import datetime
import time
# suppose two friends birthdays... Justin and Hanna
# make datetime objects justin_dt and hannah_dt
justin_dt = datetime.datetime(1992,12,04)
hannah_dt = datetime.datetime(1995,2,12)
# convert into timestamps justin_ts and hannah_ts
justin_ts = time.mktime(justin_dt.timetuple())
hannah_ts = time.mktime(hannah_dt.timetuple())
# print these out to see what they look like
print("Justin's birthday = {}".format(justin_dt))
print("Justin's timestamp = {}".format(justin_ts))
print("Hannah's birthday = {}".format(hannah_dt))
print("Hannah's timestamp = {}".format(hannah_ts))
Justin is older, and his timestamp for his birthday is a SMALLER number
Justin's birthday = 1992-12-04 00:00:00
Justin's timestamp = 723445200.0
Hannah's birthday = 1995-02-12 00:00:00
Hannah's timestamp = 792565200.0
Now... we can exploit that these are numbers to easily sort them with this function...
def oldest_timestamp(*args):
# this uses args, so we have to convert it to a list
ts_list = list(args)
# sort the list
ts_list.sort()
# return the first element in the list
return ts_list[0]
finally, we can do some testing to see if Justin is indeed older!
# find the older timestamp
older_ts = oldest_timestamp(hannah_ts, justin_ts)
print("The older timestamp is {}".format(older_ts))
# make the timestamp a date_object
older_dt = datetime.datetime.fromtimestamp(older_ts)
print("The older datetime object = {}".format(older_dt))
Success! Justin's smaller timestamp can be back-converted to show his original birthday.
The older timestamp is 723445200.0
The older datetime object = 1992-12-04 00:00:00