Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial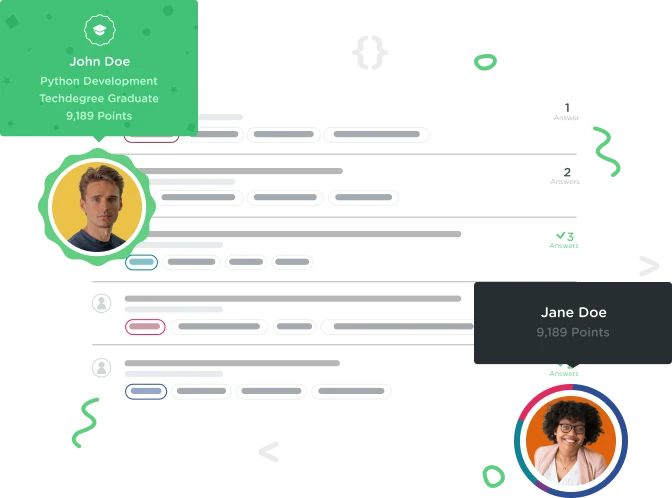

Narendra Thapa
996 Pointshello! i am little bit confused should i use if statement?
function max(value1, value2){ if( value1 > value2){ alert("the larger number is " + value1); }else{ alert(value2) } }max(10,9);
function max(x, y)
{
if()
}max(5,4);
1 Answer
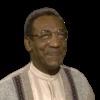
Jake Salo
13,175 PointsHi Narendra, what you need to do is test to see if x is bigger than y. To do this, you will need an if statement. You then need to return the larger of the two numbers. So you can format your function like this:
function max(x, y) {
if(x > y) {
return x;
} else {
return y;
}
}
and you can then call it within an alert like this :
alert( max(6, 11) );
So in this case, it would return y because 11 is greater than 6.
Let me know if you're still confused :)
Narendra Thapa
996 PointsNarendra Thapa
996 PointsHello jake you are so kind i'am glad you reply me thank you ! i will shortly tell you
Narendra Thapa
996 PointsNarendra Thapa
996 Pointshi jake, your code run smoothly :-) thank you for your support!! could you tell me one more thing where we use return keyword and what is the use of it?
Jake Salo
13,175 PointsJake Salo
13,175 PointsOf course - no problem!
When it comes to functions, you have the option to 'return' a value. What this means is that when you go to use the function, it will then send back the value you chose to return. So you can then use this value in other places in your program.
For example:
The very last variable we made (number) would then hold the value 4. This is because we 'returned' num1 + num2, which is the same as returning 2+2. This function can then be called in lots of places, but with different numbers. The benefit of this is so you don't need lots and lots of functions all doing the same thing. I hope this makes sense to you :)