Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial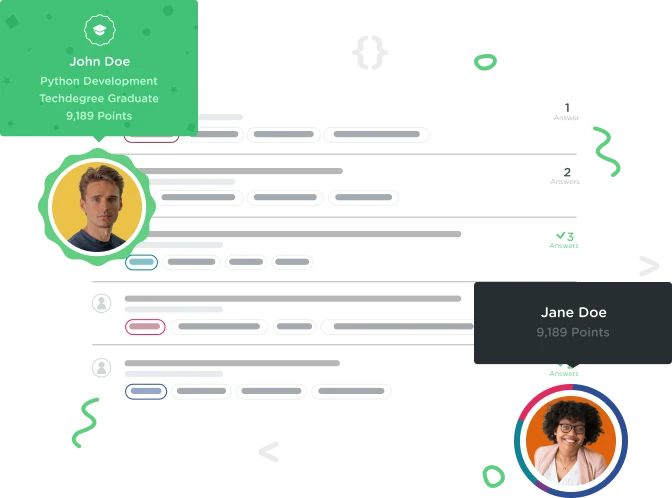
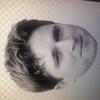
Eugene Yan
3,896 PointsHello, i have tried to run PDB in my code in Wing IDE; and every time I run the code, an exception occurs.
My code is something like this: import pdb pdb.set_trace()
def test(what): if what == 0: return 0 print('dream lvl: {}'.format(what)) recur = rec(what-1)
test(10)
and this shows up: Cannot read termcap database; using dumb terminal settings. Traceback (most recent call last): File "/Applications/Wing101.app/Contents/Resources/src/debug/tserver/sandbox.py", line 2, in <module> if __name_ == 'main': File "/Library/Frameworks/Python.framework/Versions/3.4/lib/python3.4/pdb.py", line 1579, in set_trace Pdb().set_trace(sys._getframe().f_back) File "/Library/Frameworks/Python.framework/Versions/3.4/lib/python3.4/bdb.py", line 251, in set_trace sys.settrace(self.trace_dispatch) File "/Applications/Wing101.app/Contents/Resources/bin/3.4/src/debug/tserver/dbgserver.py", line 624, in _SysSetTraceReplacement builtins.RuntimeError: WARNING: sys.settrace(<bound method Pdb.trace_dispatch of <pdb.Pdb object at 0x102676048>>) called: This breaks some debugger functionality. Use 'Ignore this exception location' in the Exceptions tool and restart debugging to suppress this warning
The code works fine if I don't import pdb and call the set_trace function.
1 Answer
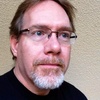
Chris Freeman
Treehouse Moderator 68,441 PointsIn running your code, I get the error NameError: name 'rec' is not defined
due to the line recur = rec(what-1)
. Did you mean to say:
recur = test(what-1)
Also, you might want to move the call to the pdb.set_trace()
to precisely where it should begin. For example, if placed as the first line within the test() function code block:
import pdb
def test(what):
pdb.set_trace() # <-- new location
if what == 0:
return 0
print('dream lvl: {}'.format(what))
recur = test(what-1) # <-- rename 'rec' to 'test'
# call test function
test(10)
In ipython
this would look like:
In [28]: def test(what):
pdb.set_trace() # <-- new location
if what == 0:
return 0
print('dream lvl: {}'.format(what))
recur = test(what-1) # <-- rename 'rec' to 'test'
....:
In [29]: test(10)
> <ipython-input-28-d012234bbc40>(3)test()
-> if what == 0:
(Pdb) cont
dream lvl: 10
> <ipython-input-28-d012234bbc40>(3)test()
-> if what == 0:
(Pdb) cont
dream lvl: 9
> <ipython-input-28-d012234bbc40>(3)test()
-> if what == 0:
(Pdb) cont
dream lvl: 8
> <ipython-input-28-d012234bbc40>(3)test()
-> if what == 0:
(Pdb) cont
dream lvl: 7
> <ipython-input-28-d012234bbc40>(3)test()
-> if what == 0:
(Pdb) cont
dream lvl: 6
> <ipython-input-28-d012234bbc40>(3)test()
-> if what == 0:
(Pdb) cont
dream lvl: 5
> <ipython-input-28-d012234bbc40>(3)test()
-> if what == 0:
(Pdb) cont
dream lvl: 4
> <ipython-input-28-d012234bbc40>(3)test()
-> if what == 0:
(Pdb) cont
dream lvl: 3
> <ipython-input-28-d012234bbc40>(3)test()
-> if what == 0:
(Pdb) cont
dream lvl: 2
> <ipython-input-28-d012234bbc40>(3)test()
-> if what == 0:
(Pdb) cont
dream lvl: 1
> <ipython-input-28-d012234bbc40>(3)test()
-> if what == 0:
(Pdb) cont
In [30]:
To help make your code examples easier to read, you might want to include triple-quotes as seen in the Markdown Cheatsheet.
Eugene Yan
3,896 PointsEugene Yan
3,896 Pointscode:
import pdb
pdb.set_trace()
def test(what):
test(10)