Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial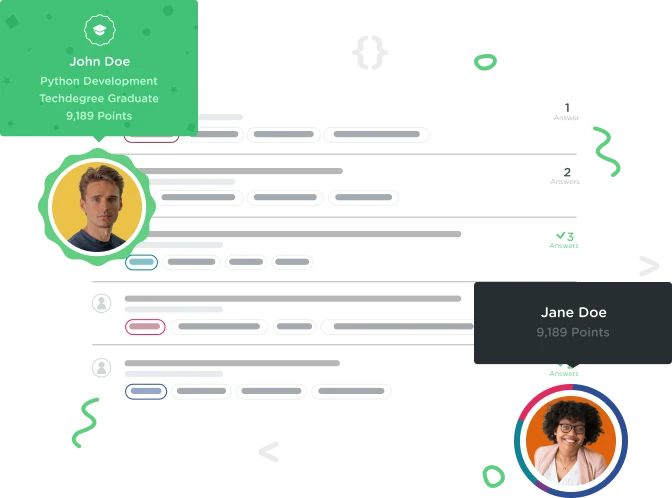

Nhan Duong
Full Stack JavaScript Techdegree Student 5,566 PointsHello, I think my code is correct, but I keep getting this "Bummer: Cannot read property "1" of Null" error?
I've tried everything and can't pass this challenge. It's going on 3 hours and I'm really not sure what I'm doing wrong. When I refresh the challenge, the paragraph texts are blue which means it's working correctly but when I click "Check Work" it won't let me pass. What am I doing wrong?
var section = document.querySelector('section');
var paragraphs = section.children;
for (let i = 0; i <= paragraphs.length; i++) {
paragraphs[i].style.color = 'blue';
}
<!DOCTYPE html>
<html>
<head>
<title>Child Traversal</title>
</head>
<body>
<section>
<p>This is the first paragraph</p>
<p>This is a slightly longer, second paragraph</p>
<p>Shorter, last paragraph</p>
</section>
<footer>
<p>© 2019</p>
</footer>
<script src="app.js"></script>
</body>
</html>
2 Answers
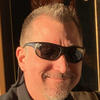
Peter Vann
36,427 PointsHi Nhan!
This passes:
var section = document.querySelector('section');
var paragraphs = section.children;
for (let i = 0; i < paragraphs.length; i++) { // Your issue is here (i <= paragraphs.length) (one loop too many)
paragraphs[i].style.color = 'blue';
}
<= is your mistake. It should be <
The length of an array is always 1 greater than the last possible ordinal position (because the indexing is zero-based).
In other words, an array of length 10 has elements indexed from 0-9.
Does that make sense?
BTW, I'm actually not a big fan of zero-based indexing - it leads to lots of confusion*, in my book, so don't feel bad.
*(The third element of an array has an index of 2 - kinda stupid if you ask me, but I didn't write the language, I just have to live with it!?! LOL)
I hope that helps.
Stay safe and happy coding!
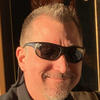
Peter Vann
36,427 PointsHi Nhan!
I actually wrote a program in Python to help me understand the Monty Hall problem (by letting you play the game "automatically" many times in a row, say, like 10,000 times where you "stay" each time and 10.000 where you "switch".
And you can then clearly see that you will win twice as often if you switch than if you stay (a clear 66.666%/33.333% split).
More info:
https://www.youtube.com/watch?v=4Lb-6rxZxx0
Anyway, I mention it because, in the program, you have to pick door # 1, 2, or 3.
I stored the doors in an array, but I gave it four elements of "door" objects.
However, I made doors[0] a bogus "door" (that never got used).
That way I could call the doors by using doors[1], doors[2], or doors[3], which made it SO MUCH EASIER to keep the doors straight in my head and NOT have to do the zero-based ordinal-position mental math constantly!?! LOL
I hope that helps.
Stay safe and happy coding!

Nhan Duong
Full Stack JavaScript Techdegree Student 5,566 PointsPeter, that is pretty awesome! Definitely keeps it straighter in your head! Thank you for the answers and insight.
Nhan Duong
Full Stack JavaScript Techdegree Student 5,566 PointsNhan Duong
Full Stack JavaScript Techdegree Student 5,566 PointsPeter, thanks for the quick reply! I literally sat for hours trying to figure out what went wrong and it came down to that! Haha. I agree, zero-based indexing may lead to a lot of confusion. I will be on the look out next time anything similar to arrays pop up on loops! Thank you again!