Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial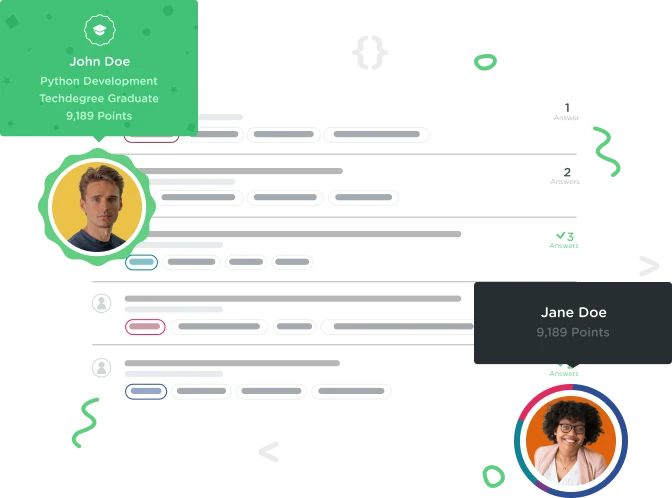

Jesse Jones
2,639 PointsHello. Please help me understand this error. Thank you.
Here is the error I'm receiving in the console: Uncaught TypeError: Cannot read property '0' of undefined at quiz.js:16
(line 16 of my code is the line directly under my for loop with the variable answer). The console is highlighting the zero in the second pair of brackets after questions in the prompt.
When I preview the page it is asking me all three questions in the array, but then it stops working. I'm assuming it's not able to store the answers. Something to do with there being three answers perhaps?
Thank you for the help. I hope this all displays properly; this is my first question on Treehouse :)
Here is the code:
function print(message) {
document.write(message);
}
var questions = [
["What's 2 times 2?", 4],
["What's 1 times 2?", 2],
["What's 3 times 2?", 6]
];
var correctAnswers = 0;
var wrongAnswers =0;
for (var i = 0; i <= questions.length; i += 1) {
var answer = prompt(questions[i][0]);
if (answer === questions[i][1]) {
correctAnswers += 1;
} else {
wrongAnswers += 1;
}
}
print('You got ' + correctAnswers + ' right, good job!');
print('You got ' + wrongAnswers + ' wrong, too bad.');
3 Answers

andren
28,558 PointsThe error comes from the <=
comparison in your for loop. The length property of an array starts at 1, while the index of an array (which you use to access the questions) starts at 0. Due to this discrepancy you end accessing an index that does not exist with that condition. You can fix that issue by simply using <
as a condition instead.
There is another issue with your code though that has to do with the fact that everything that comes from a prompt is treated as a string, even a pure number. Since you store the solution as an int you therefore need to use the parseInt
function on the answer before you compare it to the solution. Otherwise the answer (even if correct) will not be considered equal to the solution since you use the triple equals check which compares variable type in addition to variable value.

Jesse Jones
2,639 PointsOh wow, I would never have figured that out. Thank you very much!
I did catch the parseInt problem after posting, but I would never have guessed removing the equals sign from <= would do the trick. To be sure, I think what you're describing is that my i variable (accessing the array index) would have only reached the value of 2. Which would leave the loop unfinished because it could never reach the length of the array (in this case 3). Am I understanding correctly?
For the record here is my successful code.
function print(message) {
document.write(message);
}
var questions = [
["What's 2 times 2?", 4],
["What's 1 times 2?", 2],
["What's 3 times 2?", 6]
];
var correctAnswers = 0;
var wrongAnswers =0;
for (var i = 0; i < questions.length; i += 1) {
var answer = parseInt(prompt(questions[i][0]));
if (answer === questions[i][1]) {
correctAnswers += 1;
} else {
wrongAnswers += 1;
}
}
print('You got ' + correctAnswers + ' right, good job!<BR>');
print('You got ' + wrongAnswers + ' wrong, too bad.');

andren
28,558 PointsKind of. The loop does reach 3 but then it ends up throwing an error because 3 is not a valid index. Since you only have three items (index 0, 1, 2). By using less than the loop ends at 2 which is the correct place to end in order to not get outside of the index range.

Jesse Jones
2,639 PointsYou're the best! Thanks again.